In Java, Test file(Test.java) package for_vehicles; public class Test { public static void main(String[] args) { Automobile a1 = new Sedan("Honda", 4, 25, 5, "Civic", SedanType.SMALL, 4, 150.0); Automobile a2 = new Truck("Chevy", 4, 20, 3, "Silverado", 4, 13000, TruckTypes.PICKUP); TwoWheeler t1 = new Bicycle("Trek", 2, "Skye SLX", 10, 110, true, true); TwoWheeler t2 = new MotorCycle("BMW", 2, "R18", 761, 50, 91); Vehicle[] veharr = {a1, a2, t1, t2}; for(Vehicle v : veharr) { System.out.println(v.toString()); } System.out.println("The total number of Vehicles should be 4: "); System.out.println(Vehicle.numVehicles); } } The link for "here": https://stackoverflow.com/questions/5385894/how-to-zip-up-a-java-eclipse-project-so-it-can-be-easily-unfolded-onto-another-c
In Java,
Test file(Test.java)
package for_vehicles;
public class Test {
public static void main(String[] args) {
Automobile a1 = new Sedan("Honda", 4, 25, 5, "Civic", SedanType.SMALL, 4, 150.0);
Automobile a2 = new Truck("Chevy", 4, 20, 3, "Silverado", 4, 13000, TruckTypes.PICKUP);
TwoWheeler t1 = new Bicycle("Trek", 2, "Skye SLX", 10, 110, true, true);
TwoWheeler t2 = new MotorCycle("BMW", 2, "R18", 761, 50, 91);
Vehicle[] veharr = {a1, a2, t1, t2};
for(Vehicle v : veharr) {
System.out.println(v.toString());
}
System.out.println("The total number of Vehicles should be 4: ");
System.out.println(Vehicle.numVehicles);
}
}
The link for "here": https://stackoverflow.com/questions/5385894/how-to-zip-up-a-java-eclipse-project-so-it-can-be-easily-unfolded-onto-another-c



Step by step
Solved in 3 steps

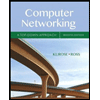
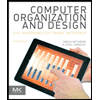
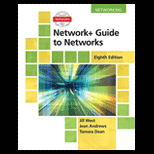
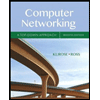
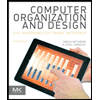
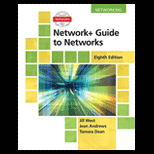
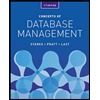
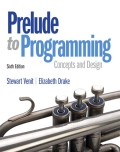
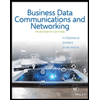