Implement the copy constructor for the StringVar class using the options given on the right side window. Place the code into the left side using the arrows. NOTE: Be careful! There are decoys! Assume that StringVar.h has the following declaration: #include class StringVar { public: StringVar() : max_length(20) { // Default constructor size is 20 value = new char[max_length+1]; value[0] = '\0'; } StringVar(int size); // Takes an int for size StringVar(const char cstr[]); // Takes a c-string and copies it StringVar(const StringVar& strObj); // Copy Constructor ~StringVar(); // Destructor int size() const { return max_length; } // Access capacity const char* c_str() const { return value; } // Access value int length() const { return strlen(value); } // Access length StringVar& operator= (const StringVar& rightObj); std::istream& operator>> (std::istream& in, StringVar& strVar); std::ostream& operator<< (std::ostream& out, const StringVar& strVar); private: int max_length; char* value; }; Organize blocks of code:
Implement the copy constructor for the StringVar class using the options given on the right side window. Place the code into the left side using the arrows. NOTE: Be careful! There are decoys!
Assume that StringVar.h has the following declaration:
#include <iostream>
class StringVar {
public:
StringVar() : max_length(20) { // Default constructor size is 20
value = new char[max_length+1];
value[0] = '\0';
}
StringVar(int size); // Takes an int for size
StringVar(const char cstr[]); // Takes a c-string and copies it
StringVar(const StringVar& strObj); // Copy Constructor
~StringVar(); // Destructor
int size() const { return max_length; } // Access capacity
const char* c_str() const { return value; } // Access value
int length() const { return strlen(value); } // Access length
StringVar& operator= (const StringVar& rightObj);
std::istream& operator>> (std::istream& in, StringVar& strVar);
std::ostream& operator<< (std::ostream& out, const StringVar& strVar);
private:
int max_length;
char* value;
};
Organize blocks of code:
![O Exit Full Screen
RESPONSE AREA
ANSWER BANK
Organize answer blocks in the proper order:
Move the necessary blocks over into the response area:
strcpy(strVar.value, value);
# #include "StringVar.h"
4
: size(strVar.size())
value = new char[strVar.length()+1];
# #include <cstring>
: max_length(strVar.length())
strcpy(value, strVar.c_str());
int max_length;
char value[strVar.max_length];
: StringVar::StringVar(const StringVar& strVar)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff5bb9956-2539-4c8d-9ee9-02ed0be838be%2F92e09d3a-b38e-4ea7-a85d-617c4b7d27f9%2Fkcwgfcl_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

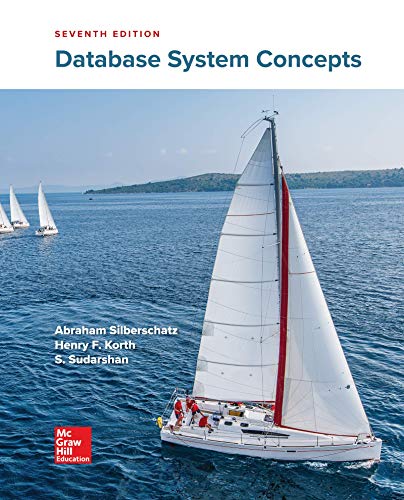
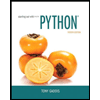
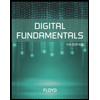
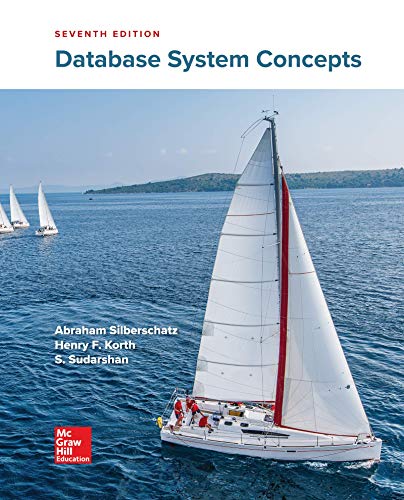
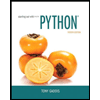
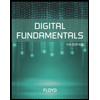
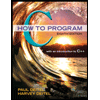
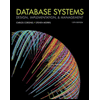
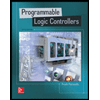