1. Implement a class: a. A Student class with a private nested GPA class and three data members: name, major, b. Use c++11 member initialization to set the default name and major to grade (` is of type GPA) "blank" and GPA to 0.0 for all objects. c. Implement multiple constructors with constructor delegation. d. GPA class: 1) All appropriate accessor and mutator functions. 2) Implement a getLetterGrade function which returns a letter based upon the GPA as such: >=3.5 A, >=2.5 B, >=1.5 C, >=1 D, all else F e. Student class: 1) All appropriate accessor and mutator functions. 2) Apply the const member function modifier as needed. 3) Implement a display member function that prints object data as demonstrated in the output example. Instantiate two objects and display their data as such: Output Example Name: John Williams Major: Music GPA: 4.00 Grade: A Name: Isaac Asimov Major: English GPA: 3.33 Grade: B
I'm stuck on this question and I don't know how I should be approaching this. What should I do?
My code so far:
-----------------
#include <iostream>
using namespace std;
class Student{
public:
Student():grade(0.0){}
void setGrade(double value){
grade = value;
}
void setName(string value){
name = value;
}
void setMajor(string value){
major = value;
}
double getGrade(){
return grade;
}
string getName(){
return name;
}
string getMajor(){
return major;
}
void addStudent(double grade, string value1, string value2){
Student::setGrade(grade);
Student::setName(value1);
Student::setMajor(value2);
}
void display(){
cout<<"Name: "<<Student::getName()<<endl;
cout<<"Major: "<<Student::getMajor()<<endl;
cout<<"GPA: "<<Student::getGrade()<<endl;
cout<<"Grade: ";
}
private:
class GPA{
public:
GPA(){}
void getLetterGrade(double grade) const{
if (grade >= 3.5){
cout<<"A";
}
if (grade >= 2.5 && grade < 3.5){
cout<<"B";
}
if (grade >= 1.5 && grade < 2.5){
cout<<"C";
}
if (grade >=1 && grade < 1.5){
cout<<"D";
}
else cout<<"F";
}
};
string name;
string major;
double grade;
};
int main() {
Student s1;
s1.addStudent(4.0,"John Williams","Music");
s1.display();
cout<<endl;
cout<<endl;
Student s2;
s2.addStudent(3.33,"Isaac Asimov","English");
s2.display();
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

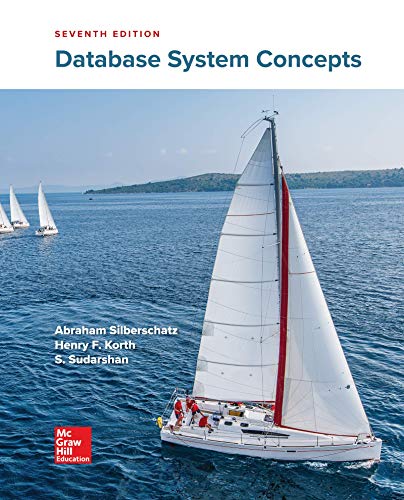
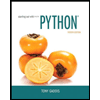
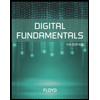
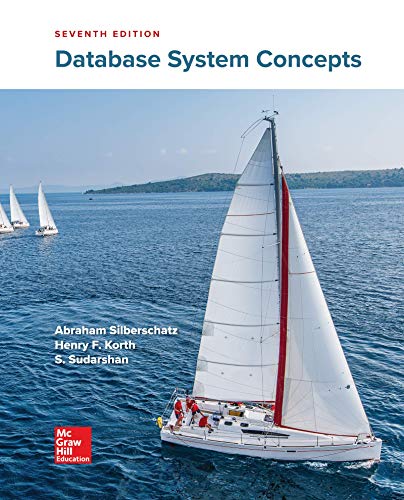
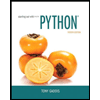
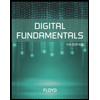
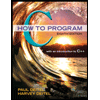
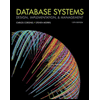
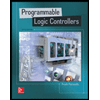