Implement the following updates and test the hangman program as specified for **ONE SINGLE** game play where the game ends when the player loses the game (**Note:** game cannot be won yet!). 4. Set the following variable in your program ``` std::string wordToGuess = "Programming"; // NOTICE the word doesn't get set to all uppercase at initialization ``` 5. Convert the `wordToGuess` to all uppercase and output 6. A game play is played where the program will continue to prompt the player for a `letterToGuess` and check if the `letterToGuess` is *found* or *not found* in `wordToGuess`. Each time the result is *not found*, the `wrongGuesses` is incremented. Repeat this process until the player loses when `wrongGuesses == 6` 1) Prompt the user for the `letterToGuess` 2) Captitalize the `letterToGuess`, so that a case-insensitive search can be completed by the program 3) Indicate to the user whether or not the `letterToGuess` was *found* or *not found* in the `wordToGuess` (case insensitive search). 4) ALWAYS Output the hangman board that corresponds to the number of `wrongGuesses` after each guess **NOT** just for the incorrect guesses, but for **ALL** guesses: correct or incorrect!. 5) The user **CANNOT** win the game yet or guess the word correctly yet. Current code C++ #include #include "myfuncts.h" // ONLY include header file (.h files) int main() { conststd::stringBOARD1 = " -------|\n | |\n |\n |\n |\n |\n -----\n"; conststd::stringBOARD2 = " -------|\n | |\n O |\n |\n |\n |\n -----\n "; conststd::stringBOARD3 = " -------|\n | |\n O |\n | |\n |\n |\n -----\n"; conststd::stringBOARD4 = " -------|\n | |\n O |\n -| |\n |\n |\n -----\n"; conststd::stringBOARD5 = " -------|\n | |\n O |\n -|- |\n |\n |\n -----\n"; conststd::stringBOARD6 = " -------|\n | |\n O |\n -|- |\n / |\n |\n -----\n"; conststd::stringBOARD7 = " -------|\n | |\n O |\n -|- |\n / \\ |\n |\n -----\n"; return 0; } Screenshots attached are start and finish of expected output
Implement the following updates and test the hangman program as specified for **ONE SINGLE** game play where the game ends when the player loses the game (**Note:** game cannot be won yet!).
4. Set the following variable in your program
```
std::string wordToGuess = "
// NOTICE the word doesn't get set to all uppercase at initialization
```
5. Convert the `wordToGuess` to all uppercase and output
6. A game play is played where the program will continue to prompt the player for a `letterToGuess` and check if the `letterToGuess` is *found* or *not found* in `wordToGuess`. Each time the result is *not found*, the `wrongGuesses` is incremented. Repeat this process until the player loses when `wrongGuesses == 6`
1) Prompt the user for the `letterToGuess`
2) Captitalize the `letterToGuess`, so that a case-insensitive search can be completed by the program
3) Indicate to the user whether or not the `letterToGuess` was *found* or *not found* in the `wordToGuess` (case insensitive search).
4) ALWAYS Output the hangman board that corresponds to the number of `wrongGuesses` after each guess **NOT** just for the incorrect guesses, but for **ALL** guesses: correct or incorrect!.
5) The user **CANNOT** win the game yet or guess the word correctly yet.
Current code C++



Step by step
Solved in 4 steps with 5 images

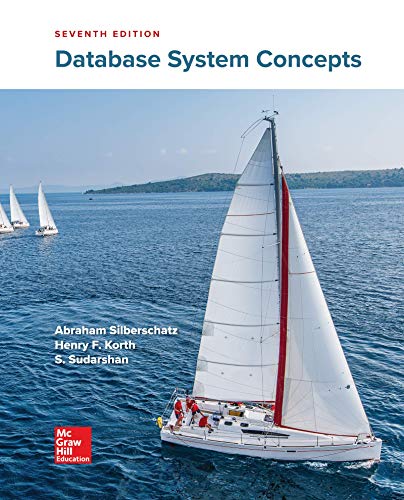
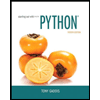
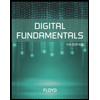
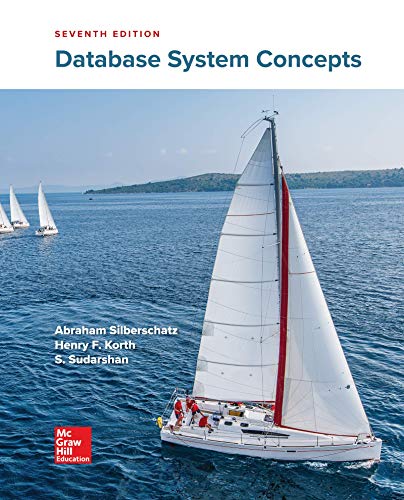
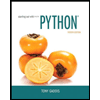
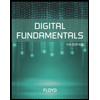
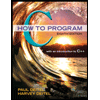
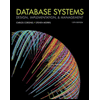
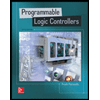