Assume that word is a variable of type String that has been assigned a value. Assume furthermore that this value always contains the letters "dr" followed by at least two other letters. For example: "undramatic", "dreck", "android", "no-drip". Assume that there is another variable declared, drWord, also of type String. Write the statements needed so that the 4-character substring word of the value of word starting with "dr" is assigned to drWord. So, if the value of word were "George slew the dragon" your code would assign the value "drag" to drWord. can't figure out what's wrong with my code!
Assume that word is a variable of type String that has been assigned a value. Assume furthermore that this value always contains the letters "dr" followed by at least two other letters. For example: "undramatic", "dreck", "android", "no-drip". Assume that there is another variable declared, drWord, also of type String. Write the statements needed so that the 4-character substring word of the value of word starting with "dr" is assigned to drWord. So, if the value of word were "George slew the dragon" your code would assign the value "drag" to drWord. can't figure out what's wrong with my code!
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Assume that word is a variable of type String that has been assigned a value. Assume furthermore that this value always contains the letters "dr" followed by at least two other letters. For example: "undramatic", "dreck", "android", "no-drip".
Assume that there is another variable declared, drWord, also of type String. Write the statements needed so that the 4-character substring word of the value of word starting with "dr" is assigned to drWord. So, if the value of word were "George slew the dragon" your code would assign the value "drag" to drWord.
can't figure out what's wrong with my code!

Transcribed Image Text:### Understanding Substring and Find Methods in String Manipulation
In this example, we are working with a line of code that applies string manipulation methods, specifically `substring` and `find`, to extract a specific part of a string. Let's break down the code step by step for better understanding:
```cpp
drWord = word.substrinf(word.find("dr", 0), 4);
```
#### Breakdown of the Code:
1. **Variable Assignment**:
```cpp
drWord =
```
This part of the code indicates that we are assigning a value to the variable `drWord`.
2. **Finding Substring**:
```cpp
word.find("dr", 0)
```
The `find` method is being called on the `word` variable. It searches for the substring `"dr"` starting from the 0th index of `word`.
- `word.find("dr", 0)` will return the index position of the first occurrence of "dr" within the `word` string.
3. **Extracting Substring**:
```cpp
word.substrinf(word.find("dr", 0), 4)
```
The `substrinf` method is being used to extract a substring from the `word` variable. `substring` requires two parameters:
- The starting index from where the extraction begins, which is given by `word.find("dr", 0)`.
- The length of the substring to be extracted, specified as `4` in this case.
It is important to note that `substrinf` should likely be `substr`. There appears to be a typo in the provided code.
### Corrected Code:
We can correct the small typo to make the code functional:
```cpp
drWord = word.substr(word.find("dr", 0), 4);
```
#### Explanation of the Corrected Code:
- `word.find("dr", 0)` locates the position of "dr" in the `word` string.
- `word.substr(start_pos, 4)` extracts the substring starting at `start_pos` of length `4`.
#### Operational Example:
If `word` is `"adrive"`:
- `word.find("dr", 0)` returns `1` because "dr" starts at index 1.
- `word.substr(1, 4)` will return `"driv"`.
This code demonstrates
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
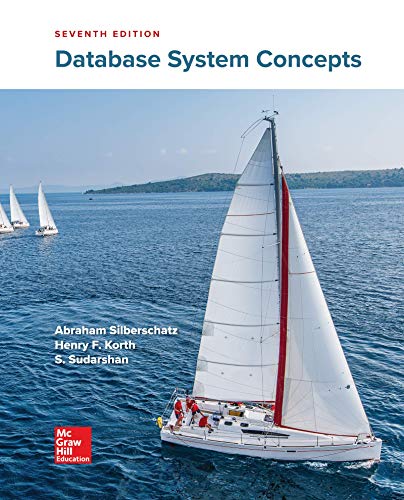
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
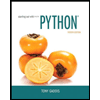
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
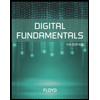
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
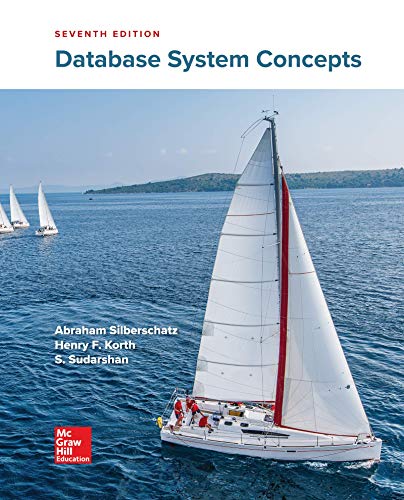
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
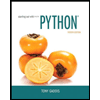
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
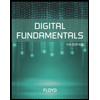
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
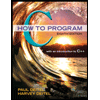
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
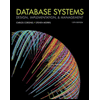
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
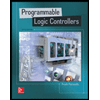
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education