Implement the following code so that fugitives and hunters can not step on each other and maximum move is 4*n(board size). import javax.swing.*; import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener; public class HuntingGame { private int n; private JButton[][] boardButtons; private int fugitiveX, fugitiveY; private int[][] hunters; // positions of the four hunters private JFrame frame; private boolean isFugitiveTurn = true; public HuntingGame(int boardSize) { n = boardSize; boardButtons = new JButton[n][n]; fugitiveX = n / 2; fugitiveY = n / 2; hunters = new int[][] { {0, 0}, {0, n - 1}, {n - 1, 0}, {n - 1, n - 1} }; initializeBoard(); updateBoard(); } private void initializeBoard() { frame = new JFrame("Hunting Game"); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setLayout(new GridLayout(n, n)); // Create board with buttons for (int i = 0; i < n; i++) { for (int j = 0; j < n; j++) { boardButtons[i][j] = new JButton(); boardButtons[i][j].setBackground(Color.WHITE); boardButtons[i][j].addActionListener(new MoveListener(i, j)); frame.add(boardButtons[i][j]); } } frame.setVisible(true); } private void updateBoard() { // Clear board for (int i = 0; i < n; i++) { for (int j = 0; j < n; j++) { boardButtons[i][j].setBackground(Color.WHITE); boardButtons[i][j].setText(""); } } // Set fugitive position boardButtons[fugitiveX][fugitiveY].setBackground(Color.BLUE); boardButtons[fugitiveX][fugitiveY].setText("F"); // Set hunter positions for (int[] hunter : hunters) { boardButtons[hunter[0]][hunter[1]].setBackground(Color.RED); boardButtons[hunter[0]][hunter[1]].setText("H"); } } private class MoveListener implements ActionListener { private int x, y; public MoveListener(int x, int y) { this.x = x; this.y = y; } @Override public void actionPerformed(ActionEvent e) { if (isFugitiveTurn) { // Fugitive move if (Math.abs(x - fugitiveX) + Math.abs(y - fugitiveY) == 1) { // Check if move is valid fugitiveX = x; fugitiveY = y; isFugitiveTurn = false; } } else { // Hunter move: select a hunter that moves one step for (int i = 0; i < 4; i++) { int hx = hunters[i][0]; int hy = hunters[i][1]; if (Math.abs(x - hx) + Math.abs(y - hy) == 1) { // Check if move is valid hunters[i][0] = x; hunters[i][1] = y; isFugitiveTurn = true; break; } } } updateBoard(); checkGameEnd(); } } private void checkGameEnd() { // Check if fugitive is surrounded and cannot move int[][] directions = {{1, 0}, {-1, 0}, {0, 1}, {0, -1}}; boolean isSurrounded = true; for (int[] dir : directions) { int newX = fugitiveX + dir[0]; int newY = fugitiveY + dir[1]; if (newX >= 0 && newX < n && newY >= 0 && newY < n) { boolean occupied = false; for (int[] hunter : hunters) { if (hunter[0] == newX && hunter[1] == newY) { occupied = true; break; } } if (!occupied) { isSurrounded = false; break; } } } if (isSurrounded) { JOptionPane.showMessageDialog(frame, "Hunters win! Fugitive is surrounded."); frame.dispose(); } }}
Implement the following code so that fugitives and hunters can not step on each other and maximum move is 4*n(board size).
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class HuntingGame {
private int n;
private JButton[][] boardButtons;
private int fugitiveX, fugitiveY;
private int[][] hunters; // positions of the four hunters
private JFrame frame;
private boolean isFugitiveTurn = true;
public HuntingGame(int boardSize) {
n = boardSize;
boardButtons = new JButton[n][n];
fugitiveX = n / 2;
fugitiveY = n / 2;
hunters = new int[][] { {0, 0}, {0, n - 1}, {n - 1, 0}, {n - 1, n - 1} };
initializeBoard();
updateBoard();
}
private void initializeBoard() {
frame = new JFrame("Hunting Game");
frame.setSize(600, 600);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new GridLayout(n, n));
// Create board with buttons
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
boardButtons[i][j] = new JButton();
boardButtons[i][j].setBackground(Color.WHITE);
boardButtons[i][j].addActionListener(new MoveListener(i, j));
frame.add(boardButtons[i][j]);
}
}
frame.setVisible(true);
}
private void updateBoard() {
// Clear board
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
boardButtons[i][j].setBackground(Color.WHITE);
boardButtons[i][j].setText("");
}
}
// Set fugitive position
boardButtons[fugitiveX][fugitiveY].setBackground(Color.BLUE);
boardButtons[fugitiveX][fugitiveY].setText("F");
// Set hunter positions
for (int[] hunter : hunters) {
boardButtons[hunter[0]][hunter[1]].setBackground(Color.RED);
boardButtons[hunter[0]][hunter[1]].setText("H");
}
}
private class MoveListener implements ActionListener {
private int x, y;
public MoveListener(int x, int y) {
this.x = x;
this.y = y;
}
@Override
public void actionPerformed(ActionEvent e) {
if (isFugitiveTurn) {
// Fugitive move
if (Math.abs(x - fugitiveX) + Math.abs(y - fugitiveY) == 1) { // Check if move is valid
fugitiveX = x;
fugitiveY = y;
isFugitiveTurn = false;
}
} else {
// Hunter move: select a hunter that moves one step
for (int i = 0; i < 4; i++) {
int hx = hunters[i][0];
int hy = hunters[i][1];
if (Math.abs(x - hx) + Math.abs(y - hy) == 1) { // Check if move is valid
hunters[i][0] = x;
hunters[i][1] = y;
isFugitiveTurn = true;
break;
}
}
}
updateBoard();
checkGameEnd();
}
}
private void checkGameEnd() {
// Check if fugitive is surrounded and cannot move
int[][] directions = {{1, 0}, {-1, 0}, {0, 1}, {0, -1}};
boolean isSurrounded = true;
for (int[] dir : directions) {
int newX = fugitiveX + dir[0];
int newY = fugitiveY + dir[1];
if (newX >= 0 && newX < n && newY >= 0 && newY < n) {
boolean occupied = false;
for (int[] hunter : hunters) {
if (hunter[0] == newX && hunter[1] == newY) {
occupied = true;
break;
}
}
if (!occupied) {
isSurrounded = false;
break;
}
}
}
if (isSurrounded) {
JOptionPane.showMessageDialog(frame, "Hunters win! Fugitive is surrounded.");
frame.dispose();
}
}
}

Step by step
Solved in 2 steps

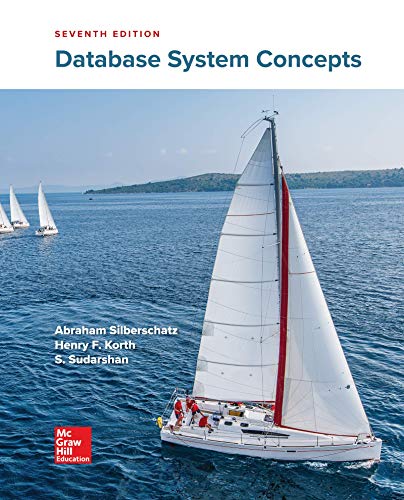
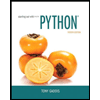
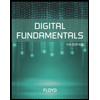
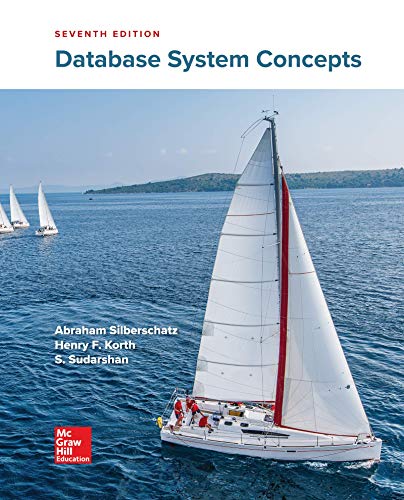
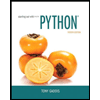
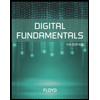
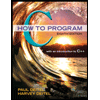
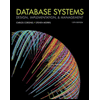
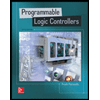