I keep getting these errors when I run the code below: Code is in C++ ____animal.cpp_______ #include "animal.h" Animal::Animal() { } Animal::Animal(string name,string gender, string color, double weight) { this->name = name; this->gender = gender; this->color = color; this->weight = weight; } void Animal::PrintInfo() { cout<<"Name : "< using namespace std; class Animal { private: double weight; string name; string gender; string color; public: Animal(); Animal(string,string , string, double); virtual void PrintInfo(); }; #endif ___horse.h___ #ifndef __HORSE_H #define __HORSE_H #include "animal.h" class Horse : public Animal { private: string breed; int id; string comments; public: Horse(); Horse(string,double,string,string,int,string,string); void PrintInfo(); }; #endif ____horse.cpp____ #include "horse.h" Horse::Horse() { } Horse::Horse(string breed, double weight , string name ,string gender, int id, string color , string comments):Animal(name,gender,color,weight) { this->breed = breed; this->id = id; this->comments = comments; } void Horse::PrintInfo() { Animal::PrintInfo(); cout<<"Breed : "<breed<id<comments< #include void clearBuffer() { cin.clear(); cin.ignore(numeric_limits::max(),'\n'); } int main() { int size = 5; Horse *snakes = new Horse[5]; string breed; double weight; string name; string gender; int id; string color ; string comments; for(int i = 0;i> weight; clearBuffer(); cout<<"Enter Name : "; getline(cin,name); cout<<"Enter Gender : "; getline(cin,gender); cout<<"Enter Id : "; cin >> id; clearBuffer(); cout<<"Enter Color : "; getline(cin,color); cout<<"Enter Comments : "; getline(cin,comments); snakes[i] = Horse(breed,weight,name,gender,id,color,comments);; } for(int i = 0;iPrintInfo(); } delete[] snakes; return 0; }
I keep getting these errors when I run the code below: Code is in C++
____animal.cpp_______
#include "animal.h"
Animal::Animal()
{
}
Animal::Animal(string name,string gender, string color, double weight)
{
this->name = name;
this->gender = gender;
this->color = color;
this->weight = weight;
}
void Animal::PrintInfo()
{
cout<<"Name : "<<name<<endl;
cout<<"Gender : "<<gender<<endl;
cout<<"Color : "<<color<<endl;
cout<<"Weight : "<<weight<<endl;
}
__animal.h___
#ifndef __ANIMAL_H
#define __ANIMAL_H
#include<iostream>
using namespace std;
class Animal
{
private:
double weight;
string name;
string gender;
string color;
public:
Animal();
Animal(string,string , string, double);
virtual void PrintInfo();
};
#endif
___horse.h___
#ifndef __HORSE_H
#define __HORSE_H
#include "animal.h"
class Horse : public Animal
{
private:
string breed;
int id;
string comments;
public:
Horse();
Horse(string,double,string,string,int,string,string);
void PrintInfo();
};
#endif
____horse.cpp____
#include "horse.h"
Horse::Horse()
{
}
Horse::Horse(string breed, double weight , string name ,string gender, int id, string color , string comments):Animal(name,gender,color,weight)
{
this->breed = breed;
this->id = id;
this->comments = comments;
}
void Horse::PrintInfo()
{
Animal::PrintInfo();
cout<<"Breed : "<<this->breed<<endl;
cout<<"Id : "<<this->id<<endl;
cout<<"Comments : "<<this->comments<<endl;
}
____ horsemain.cpp_____
#include "horse.h"
#include<limits>
#include<cstdlib>
void clearBuffer()
{
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(),'\n');
}
int main()
{
int size = 5;
Horse *snakes = new Horse[5];
string breed;
double weight;
string name;
string gender;
int id;
string color ;
string comments;
for(int i = 0;i<size;i++)
{
cout<<"\nEnter Breed : ";
getline(cin,breed);
cout<<"Enter Weight : ";
cin >> weight;
clearBuffer();
cout<<"Enter Name : ";
getline(cin,name);
cout<<"Enter Gender : ";
getline(cin,gender);
cout<<"Enter Id : ";
cin >> id;
clearBuffer();
cout<<"Enter Color : ";
getline(cin,color);
cout<<"Enter Comments : ";
getline(cin,comments);
snakes[i] = Horse(breed,weight,name,gender,id,color,comments);;
}
for(int i = 0;i<size;i++)
{
Animal *animal;
animal = &snakes[i];
cout<<"\n";
animal->PrintInfo();
}
delete[] snakes;
return 0;
}
![## Understanding Compiling Errors in C Programming
### Error Message Transcript:
```
/usr/bin/make -j8 -e -f Makefile
---------Building project:[ animal.h - Debug ]---------
/usr/bin/g++ -o Debug/animal.h @"animal.h.txt" -L.
Undefined symbols for architecture x86_64:
"_main", referenced from:
implicit entry/start for main executable
ld: symbol(s) not found for architecture x86_64
clang: error: linker command failed with exit code 1 (use -v to see invocation)
make[1]: *** [Debug/animal.h] Error 1
make: *** [All] Error 2
=== build ended with errors (1 errors, 0 warnings) ===
```
### Explanation:
This error output occurs when attempting to compile a project using the `make` build tool in a C or C++ environment. Here's a breakdown of the error and potential solutions:
- **Undefined Symbols**: The error message indicates "Undefined symbols for architecture x86_64." This typically means the compiler is looking for the `main` function, which is the entry point of any C or C++ program.
- **Implicit Entry**: The message "_main, referenced from: implicit entry/start for main executable" means that the `main` function is missing or not correctly linked. In C/C++ programs, `main` is essential as it is the starting point of execution.
- **Linker Command Failed**: The error "linker command failed with exit code 1" signifies that the linker could not complete its task, which is often due to missing symbols or incorrect paths to libraries or source files.
- **Makefile Error**: The `make` tool exits with errors denoted by "make: *** [All] Error 2", indicating that the build process cannot proceed because of the errors encountered.
### Solutions:
1. **Ensure Main Function Exists**: Verify that there is a `main` function in your source files. If your project is a library without a `main` function, adjust your build settings accordingly.
2. **Check File Extensions**: Ensure that you're compiling source files (like `.cpp` or `.c`), not header files (`.h`) which typically don't contain a `main` function.
3. **Linking Libraries**: Make sure all necessary libraries are correctly linked. Check your `Makefile` or](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7b9451f1-a2f9-47cb-b8b6-654ac5ea1321%2F23100457-ea04-4575-8fd7-5d35c94417af%2Fiqv22om_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

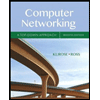
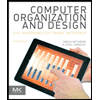
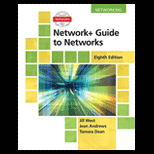
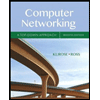
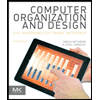
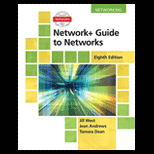
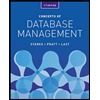
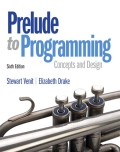
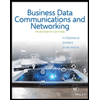