his code is correct but can you do call this createKidPerson() in the main Code: #include using namespace std; typedef struct { int age; char gender; } Person; void displayPerson(Person); Person createKidPerson(Person father, Person mother); int main(void) { Person father; Person mother; father.gender = 'M'; mother.gender = 'F'; cout<<"Enter the Male Person's age: "; cin>>father.age; cout<<"Enter the Female Person's age: "; cin>>mother.age; Person kid = createKidPerson(father, mother); displayPerson(kid); return 0; } Person createKidPerson(Person father, Person mother) { Person p; if((father.age==mother.age) && (father.age%2==1)){ p.gender='F'; } else if(father.age>=mother.age) p.gender='M'; else p.gender='F'; p.age=1; return p; } void displayPerson(Person p) { cout << "\nPERSON DETAILS:" << endl; cout << "Age: " << p.age << endl; cout << "Gender: "; if(p.gender == 'M') { cout << "Male"; } else { cout << "Female"; } }
This code is correct but can you do call this createKidPerson() in the main
Code:
#include <iostream>
using namespace std;
typedef struct {
int age;
char gender;
} Person;
void displayPerson(Person);
Person createKidPerson(Person father, Person mother);
int main(void) {
Person father;
Person mother;
father.gender = 'M';
mother.gender = 'F';
cout<<"Enter the Male Person's age: ";
cin>>father.age;
cout<<"Enter the Female Person's age: ";
cin>>mother.age;
Person kid = createKidPerson(father, mother);
displayPerson(kid);
return 0;
}
Person createKidPerson(Person father, Person mother)
{
Person p;
if((father.age==mother.age) && (father.age%2==1)){
p.gender='F';
}
else if(father.age>=mother.age)
p.gender='M';
else
p.gender='F';
p.age=1;
return p;
}
void displayPerson(Person p)
{
cout << "\nPERSON DETAILS:" << endl;
cout << "Age: " << p.age << endl;
cout << "Gender: ";
if(p.gender == 'M') {
cout << "Male";
} else {
cout << "Female";
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

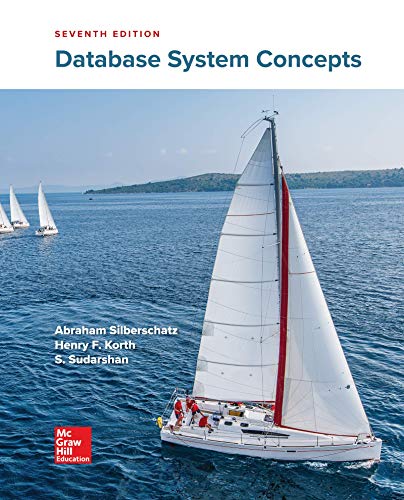
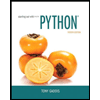
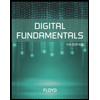
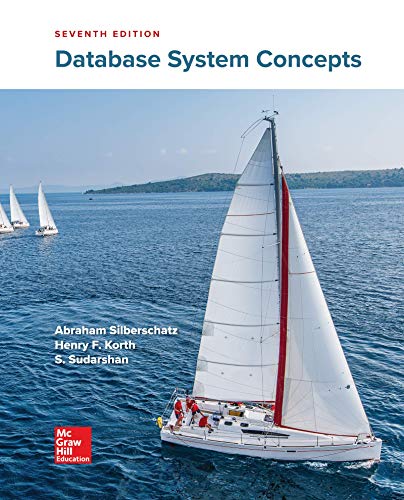
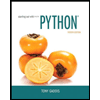
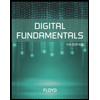
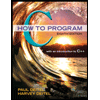
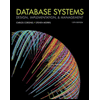
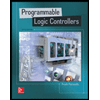