Help me correct my C++ code please! Pictures included. My current code with the error picture; #include using namespace std; class Date { int day; int month; int year; public: Date (int d, int m, int y); void set_day (int day); void set_month (int month); void set_year (int year); int get_day (); int get_month (); int get_year (); void display (); }; Date::Date (int d, int m, int y) { if (m < 1 || m >12) month = 1; else month = m; day = d; year = y; } void Date::set_day (int day) { this->day = day; } void Date::set_month (int month) { this->month = month; } void Date::set_year (int year) { this->year = year; } int Date::get_day () { return day; } int Date::get_month () { return month; } int Date::get_year () { return year; } void Date::display () { if (month < 10){ cout << "0" << month; } else{ cout << month; } cout << "/"; if (day < 10){ cout << "0" << day; } else{ cout << day; } cout << "/"; cout << year << endl; } int main () { int day, month, year; cout << "Enter the month, "; cin >> day; cout << "the day, "; cin >> month; cout << "and the year:"; cin >> year; Date date (day, month, year); date.display (); system ("pause"); return 0; }
Help me correct my C++ code please! Pictures included. My current code with the error picture;
#include <iostream>
using namespace std;
class Date
{
int day;
int month;
int year;
public:
Date (int d, int m, int y);
void set_day (int day);
void set_month (int month);
void set_year (int year);
int get_day ();
int get_month ();
int get_year ();
void display ();
};
Date::Date (int d, int m, int y)
{
if (m < 1 || m >12)
month = 1;
else
month = m;
day = d;
year = y;
}
void Date::set_day (int day)
{
this->day = day;
}
void Date::set_month (int month)
{
this->month = month;
}
void Date::set_year (int year)
{
this->year = year;
}
int Date::get_day ()
{
return day;
}
int Date::get_month ()
{
return month;
}
int Date::get_year ()
{
return year;
}
void Date::display ()
{
if (month < 10){
cout << "0" << month;
}
else{
cout << month;
}
cout << "/";
if (day < 10){
cout << "0" << day;
}
else{
cout << day;
}
cout << "/";
cout << year << endl;
}
int main ()
{
int day, month, year;
cout << "Enter the month, ";
cin >> day;
cout << "the day, ";
cin >> month;
cout << "and the year:";
cin >> year;
Date date (day, month, year);
date.display ();
system ("pause");
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

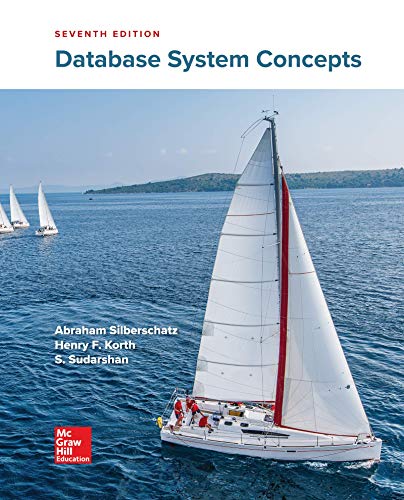
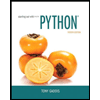
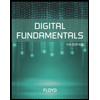
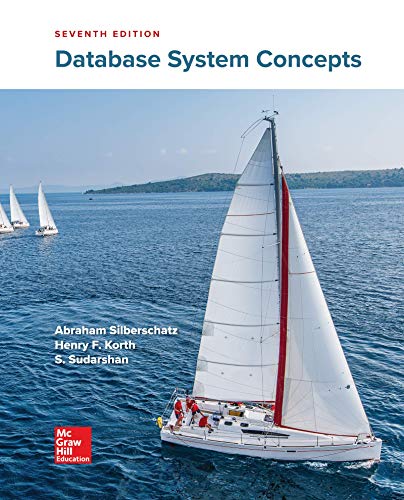
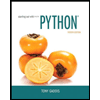
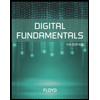
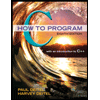
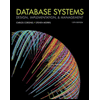
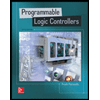