I have created the following code in java, replit, that's a guessing game. Attached are the classes I've created as separate files. Since I can only upload 2 files, I have written the code in class Main at the very end. Now I need to create and write data into a CSV file, tracking the results of multiple players scores in the Guessing Game. This will require the use of several imported java class including : java.io.File java.io.FileWriter java.util.Scanner java.util.Random java.util.UUID java.io.IOException; And I know nothing about this, so I really need help. You would need to modify all my classes/files accordingly. Also ignore the results.csv file that I tried creating in the pdfs attached. Basically you would need to use the File object along with the Player object, and the properties of the player class is what needs to be written into the CSV. I haven't included any properties of the player class yet (like private UUID id; private String name; private int totalGames; private int totalGuesses; private int bestGame; something along those lines). Use a File and the Scanner to resume a players set of games as read from the CSV. This is how class main looks like: import java.util.Scanner; import java.util.Random; public class Main { public static void main(String[] args) { Scanner console = new Scanner(System.in); Random random = new Random(); Game game = new Game(console, random); game.startGame(); console.close(); } }
I have created the following code in java, replit, that's a guessing game. Attached are the classes I've created as separate files. Since I can only upload 2 files, I have written the code in class Main at the very end. Now I need to create and write data into a CSV file, tracking the results of multiple players scores in the Guessing Game. This will require the use of several imported java class including :
- java.io.File
- java.io.FileWriter
- java.util.Scanner
- java.util.Random
- java.util.UUID
- java.io.IOException;
And I know nothing about this, so I really need help. You would need to modify all my classes/files accordingly. Also ignore the results.csv file that I tried creating in the pdfs attached. Basically you would need to use the File object along with the Player object, and the properties of the player class is what needs to be written into the CSV. I haven't included any properties of the player class yet (like private UUID id; private String name; private int totalGames; private int totalGuesses; private int bestGame; something along those lines). Use a File and the Scanner to resume a players set of games as read from the CSV.
This is how class main looks like:
import java.util.Scanner;
import java.util.Random;
public class Main {
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
Random random = new Random();
Game game = new Game(console, random);
game.startGame();
console.close();
}
}



Step by step
Solved in 3 steps

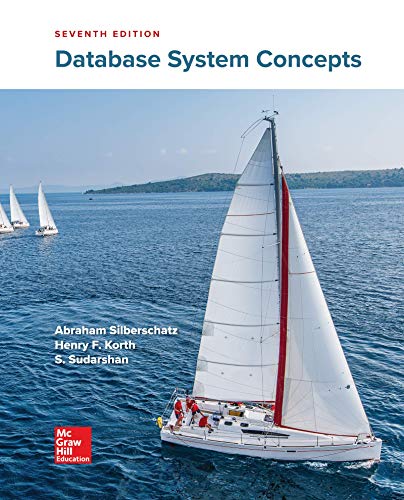
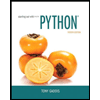
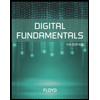
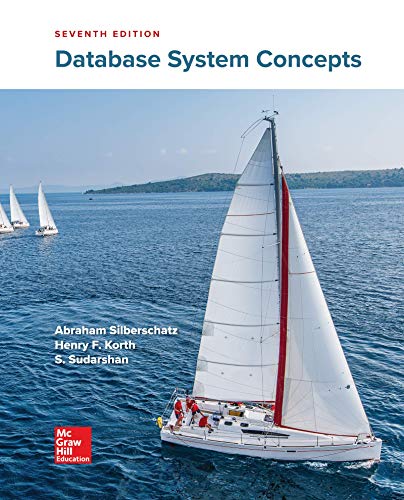
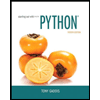
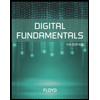
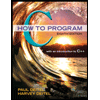
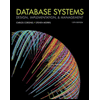
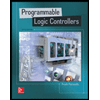