Hi - So currently I have code in java (in replit.com) that writes to a player_results.csv file I've created based the results of a game played in the console, with a random ID, the player's name, total games, total guesses, and best game. It's basically a guessing game where, using a scanner, the program asks for the user's name, "thinks" of a number, and you have to guess it based on whether the program says the number you guessed is higher or lower than the number it's thinking. It asks to play the game again, and if you say something that starts with "y" or "Y" (Example: Yeehaw or yes or Yass or even Yeet or something) then it continues, otherwise it stops the game and the results are printed out(total games, total guesses, etc. you'll see in my code). If you see one of the files I have attached, it has main.java's code along with the output of the console in the screenshot. After I played the game (twice) as you see in the console, the output in player_results.csv was the following: 4ee9f207-6840-4d3c-bd3d-48c810282f70,Sid,1,5,5 a5e157d1-9bff-4d63-b814-6d8cabf5423a,Shri,2,11,5 I have also attached Game.java, and Player.java looks like this: import java.util.Scanner; import java.util.UUID; public class Player { private UUID id; private String name; private int totalGames; private int totalGuesses; private int bestGame; public Player() { this.id = UUID.randomUUID(); this.name = null; this.totalGames = 0; this.totalGuesses = 0; this.bestGame = Integer.MAX_VALUE; } public UUID getId() { return id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getTotalGames() { return totalGames; } public void setTotalGames(int totalGames) { this.totalGames = totalGames; } public int getTotalGuesses() { return totalGuesses; } public void setTotalGuesses(int totalGuesses) { this.totalGuesses = totalGuesses; } public int getBestGame() { return bestGame; } public void setBestGame(int bestGame) { this.bestGame = bestGame; } public int playGame(Scanner console, int answer) { System.out.println("I'm thinking of a number between 1 and " + Game.MAX + "..."); int guesses = 0;
Hi - So currently I have code in java (in replit.com) that writes to a player_results.csv file I've created based the results of a game played in the console, with a random ID, the player's name, total games, total guesses, and best game. It's basically a guessing game where, using a scanner, the program asks for the user's name, "thinks" of a number, and you have to guess it based on whether the program says the number you guessed is higher or lower than the number it's thinking. It asks to play the game again, and if you say something that starts with "y" or "Y" (Example: Yeehaw or yes or Yass or even Yeet or something) then it continues, otherwise it stops the game and the results are printed out(total games, total guesses, etc. you'll see in my code). If you see one of the files I have attached, it has main.java's code along with the output of the console in the screenshot. After I played the game (twice) as you see in the console, the output in player_results.csv was the following:
4ee9f207-6840-4d3c-bd3d-48c810282f70,Sid,1,5,5
a5e157d1-9bff-4d63-b814-6d8cabf5423a,Shri,2,11,5
I have also attached Game.java, and Player.java looks like this:
import java.util.Scanner;
import java.util.UUID;
public class Player {
private UUID id;
private String name;
private int totalGames;
private int totalGuesses;
private int bestGame;
public Player() {
this.id = UUID.randomUUID();
this.name = null;
this.totalGames = 0;
this.totalGuesses = 0;
this.bestGame = Integer.MAX_VALUE;
}
public UUID getId() {
return id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getTotalGames() {
return totalGames;
}
public void setTotalGames(int totalGames) {
this.totalGames = totalGames;
}
public int getTotalGuesses() {
return totalGuesses;
}
public void setTotalGuesses(int totalGuesses) {
this.totalGuesses = totalGuesses;
}
public int getBestGame() {
return bestGame;
}
public void setBestGame(int bestGame) {
this.bestGame = bestGame;
}
public int playGame(Scanner console, int answer) {
System.out.println("I'm thinking of a number between 1 and " + Game.MAX + "...");
int guesses = 0;
while (true) {
System.out.print("Your guess? ");
if (console.hasNextInt()) {
int guess = console.nextInt();
guesses++;
if (guess == answer) {
System.out.println("You got it right in " + guesses + " guess" + (guesses == 1 ? "!" : "es!"));
break;
} else if (guess < answer) {
System.out.println("It's higher.");
} else {
System.out.println("It's lower.");
}
} else {
System.out.println("Invalid input. Please enter a valid integer.");
console.next();
}
}
return guesses;
}
}
Now I want help with this: Please add code to read from my csv file. Convert those strings from the csv file into a player object that you can use to resume a series of games.
![Search
classpath
project
J-04-Ging Game.pdf
Game Ja
GameManagejas
player_results.com
1. Playjava
Packager Fian
-
p
-Took
Authentication
Char
Code Search
> Conce
D
Dil Debugger
Doce
Extensions
Pack
POL
Ⓒ Secrets
Shell
hbi
Syer Dependencias
Threads
C
Main.java
Gja
B
1 Import java.io.*;
2 Import java.util.Scanner;
3 tmport java.util.Randon;
4
5, public class Game {
56
7
11,
៧៣ ៧.១.១៨ ១ ១ ន ដ ន ន ដ វ ន ន ន ន ន ដ អ ឧ ឩ ខន ៦គ.ខ៥៥១ ឧឧ ផ ១ គគី
12
13
15,
28
30
31
32
33
34
35
36
37
38
39
48
41
42
43
45
46
47
38
31
32
33,
35
AAAAASENO388688RÉNAZARERA
37
19
01
02
03,
06
07
09
78
71,
72
73
75
76
77
78
79
38
Cariss 81
82
839
85}
public static final in MAX = 100;
}
Playeva x
private final Scanner console;
private final Random randon;
private static final String CSV_FILE_PATH"player_results.csv";
74 }
public Genel Scanner console, Random random) {
this.console console;
this.randon randon;
}
public void startGame() {
int totalGames= 0;
int totalGuesses - 8;
}
player. x
int bestGame= Integer.MAX_VALUE;
while (true) {
}
}
Player player = new Player();
// Prompt the user for their name
System.out.print("Enter your name: ");
String playerName console.next();
player.setName(playerName);
int answer = 1 + random.nextInt(MAX);
int guesses - player.playGame(console, answer);
totalGames++;
totalGuesses + guesses;
Gameja
bestGame Math.min(bestGame, guesses);
player.setTotalGames (totalGames);
player.setTotalGuesses (totalGuesses);
player.setBestGame(bestGame);
savePlayerToCSV(player);
System.out.println("Play again y/n?");
String playAgain console.next().toLowerCase();
break;
if (playAgain.startsWith("y")) {
}
private vold printoverallStatistics(int totalGames, int totalGuesses, int bestGame) {
+ totalGames);
printoverallStatistics(totalGames, totalGuesses, bestGame);
private void savePlayer ToCSV(Player player) {
System.out.println("Total games:
System.out.printin("Total guesses: + totalGuesses);
System.out.println("Guesses/game: +(totalGames=0?0 totalGuesses / totalGames));
System.out.println("Best game:" + bestGame);
try (Filewriter writer = new FileWriter(CSV_FILE_PATH, true);
BufferedWriter bw new Bufferedwriter(writer);
PrintWriter out new PrintWriter(bw)) {
out.println(player.getId() +
player.getName()
player.getTotalGames() -
player.getTotalGuesses() +
player.getBestGame());
} catch (10Exception e) {
e.printStackTrace();
}
public static vold main(String[] args) {
Scanner console
new Scanner(System.in);
Randon randon - new Random();
Game game - new Game(console, random);
game.startGame();
console.close();](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7b74556d-13eb-4ded-8ba4-3b168abe325e%2F9630230e-6da8-4101-84b6-85de20369326%2Fn5s2avk_processed.png&w=3840&q=75)
![Search
✓ Files
Packager files
target
pom.xml
.settings
.classpath
.project
04 - Guessing Game.pdf
Game.java
GameManager.java
Main.java
player_results.csv
Player.java
✓ Tools
Recent
All
CAI
до
Authentication
Chat
Q Code Search
> Console
+J
...
Ctrl Shift F
Main.java Ⓒ X
Main.java
39
40
41
42
43
44
45
46
47
48 */
49 import java.util.Scanner;
50
import java.util.Random;
51
52
53
54 v
55
56
57
58
59
60
61
62
63
64
65
66
Player.javax player_results.csv X
Overall results:
Total games = 3
Total guesses = 19
Guesses/game
= 6.3
= 4
Best game
}
...where only the specifics of the games are different.
The formatting of the results, should match exactly.
public class Main {
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
Random random = new Random();
}
Game.java
Game game = new Game(console, random);
game.startGame();
console.close();
+
> Console X
Run
Enter your name: Sid
I'm thinking of a number between 1 and 100...
Your guess? 40
It's lower.
Your guess? 30
It's lower.
Your guess? 20
It's higher.
Your guess? 25
It's higher.
Your guess? 28
You got it right in 5 guesses!
Play again y/n?
Yaaassssss
Enter your name: Shri
I'm thinking of a number between 1 and 100...
Your guess? 50
It's higher.
Your guess? 70
It's lower.
Your guess? 60
It's higher.
Shell X +
Your guess? 67
It's lower.
Your guess? 62
It's lower.
Your guess? 61
You got it right in 6 guesses!
Play again y/n?
Nawww
Total games: 2
Total guesses: 11
Guesses/game: 5
Best game: 5
38s on 21:53:21, 01/03 ✓](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7b74556d-13eb-4ded-8ba4-3b168abe325e%2F9630230e-6da8-4101-84b6-85de20369326%2Facrowkf_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

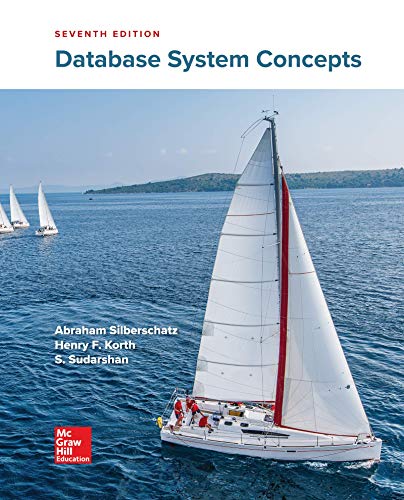
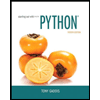
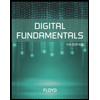
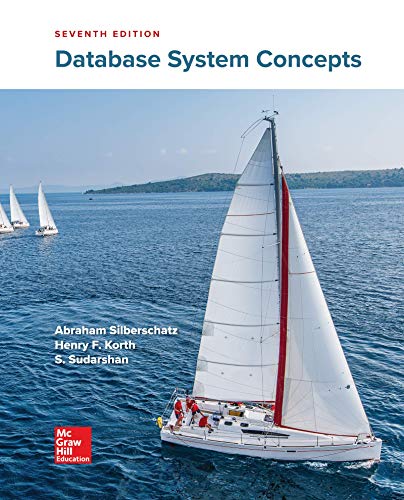
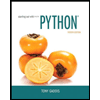
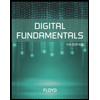
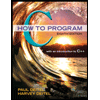
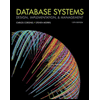
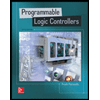