I have a coding assignment that I'm having trouble on. The language that I am coding in is C. I'm trying to pass an array of structs into a function, and then print out a certain member of the struct, to make sure I'm accessing them correctly. However, I keep coming across this error and I'm not at all sure why. Project3_soccer.c: In function ‘findPlayer’:Project3_soccer.c:108:17: error: expected expression before ‘playerData’printf("%d",playerData[i].playerNumber); ^~~~~~~~~~ My code is below: #include <stdio.h>#include <stdbool.h> typedef struct playerData_struct {int playerNumber;char playerName[100];double playerRating;} playerData; int findPlayer(int whichPlayer, const playerData jerseyNumbers[], int maxJersyCount); bool jerseyValid(int playerJerseyNumber); bool ratingValid(double playerRating); void printPlayer(playerData* player); int main(void) {int i = 0;int j = 0;int index = 0;double rating = 0;struct playerData_struct players[10];char menuOp = 'x';while (menuOp != 'q') {// Menuprintf("\nMENU\n");printf("a - Add a new player\n");printf("u - Update player information\n");printf("r - Remove a player from the roster\n");printf("d - Display player information\n");printf("p - Print the full roster\n");printf("s - Print \"Star\" players\n");printf("q - Quit\n"); printf("\nChoose an option: ");scanf(" %c", &menuOp); switch (menuOp) { case 'a':for (j = 0;j < 10;j++) {players[j].playerNumber = j + 3;printf("%d\n",players[j].playerNumber);}index = findPlayer(7,players,j);printf("%d",index);/*if (j == 0) {printf("Enter player jersey number: ");scanf("%d",&player[j].playerNumber);if (jerseyValid(player[j].playerNumber) == 1) {printf("Enter player first or nick name: ");scanf("%s",player[j].playerName);printf("Enter player rating: ");scanf("%lf",&player[j].playerRating);}else {break;}}*/case 'u': case 'r': case 'd': case 'p':printf("ROSTER\n------\n");for (i = 0;i < 10;i++) {if ((players[i].playerNumber > 0) && (players[i].playerNumber) < 99) {printf("%s\n",players[i].playerName);printf("%d\n",players[i].playerNumber);printf("%lf\n",players[i].playerRating);printf("\n");}}case 's':printf("Enter minimum STAR rating: ");scanf("%lf",&rating);printf("MY STARS\n--------\n");for (i = 0;i < 10;i++) {if (players[i].playerRating > rating) {printf("%s\n",players[i].playerName);printf("%d\n",players[i].playerNumber);printf("%lf\n",players[i].playerRating);printf("\n");}}break;case 'q':break;default:;}}return 0;} int findPlayer(int whichPlayer, const playerData jerseyNumbers[], int maxJersyCount) {int index = 0;printf("%d",playerData[i].jerseyNumbers);return index;} bool jerseyValid(int playerJerseyNumber) {if ((playerJerseyNumber > 0) && (playerJerseyNumber < 100)) {return true;}else {return false;}} bool ratingValid(double playerRating) {if ((playerRating >= 0) && (playerRating <= 100)) {return true;}else {return false;}}
I have a coding assignment that I'm having trouble on. The language that I am coding in is C. I'm trying to pass an array of structs into a function, and then print out a certain member of the struct, to make sure I'm accessing them correctly. However, I keep coming across this error and I'm not at all sure why.
Project3_soccer.c: In function ‘findPlayer’:
Project3_soccer.c:108:17: error: expected expression before ‘playerData’
printf("%d",playerData[i].playerNumber);
^~~~~~~~~~
My code is below:
#include <stdio.h>
#include <stdbool.h>
typedef struct playerData_struct {
int playerNumber;
char playerName[100];
double playerRating;
} playerData;
int findPlayer(int whichPlayer, const playerData jerseyNumbers[], int maxJersyCount);
bool jerseyValid(int playerJerseyNumber);
bool ratingValid(double playerRating);
void printPlayer(playerData* player);
int main(void) {
int i = 0;
int j = 0;
int index = 0;
double rating = 0;
struct playerData_struct players[10];
char menuOp = 'x';
while (menuOp != 'q') {
// Menu
printf("\nMENU\n");
printf("a - Add a new player\n");
printf("u - Update player information\n");
printf("r - Remove a player from the roster\n");
printf("d - Display player information\n");
printf("p - Print the full roster\n");
printf("s - Print \"Star\" players\n");
printf("q - Quit\n");
printf("\nChoose an option: ");
scanf(" %c", &menuOp);
switch (menuOp) {
case 'a':
for (j = 0;j < 10;j++) {
players[j].playerNumber = j + 3;
printf("%d\n",players[j].playerNumber);
}
index = findPlayer(7,players,j);
printf("%d",index);
/*
if (j == 0) {
printf("Enter player jersey number: ");
scanf("%d",&player[j].playerNumber);
if (jerseyValid(player[j].playerNumber) == 1) {
printf("Enter player first or nick name: ");
scanf("%s",player[j].playerName);
printf("Enter player rating: ");
scanf("%lf",&player[j].playerRating);
}
else {
break;
}
}*/
case 'u':
case 'r':
case 'd':
case 'p':
printf("ROSTER\n------\n");
for (i = 0;i < 10;i++) {
if ((players[i].playerNumber > 0) &&
(players[i].playerNumber) < 99) {
printf("%s\n",players[i].playerName);
printf("%d\n",players[i].playerNumber);
printf("%lf\n",players[i].playerRating);
printf("\n");
}
}
case 's':
printf("Enter minimum STAR rating: ");
scanf("%lf",&rating);
printf("MY STARS\n--------\n");
for (i = 0;i < 10;i++) {
if (players[i].playerRating > rating) {
printf("%s\n",players[i].playerName);
printf("%d\n",players[i].playerNumber);
printf("%lf\n",players[i].playerRating);
printf("\n");
}
}
break;
case 'q':
break;
default:
;
}
}
return 0;
}
int findPlayer(int whichPlayer, const playerData jerseyNumbers[], int maxJersyCount) {
int index = 0;
printf("%d",playerData[i].jerseyNumbers);
return index;
}
bool jerseyValid(int playerJerseyNumber) {
if ((playerJerseyNumber > 0) && (playerJerseyNumber < 100)) {
return true;
}
else {
return false;
}
}
bool ratingValid(double playerRating) {
if ((playerRating >= 0) && (playerRating <= 100)) {
return true;
}
else {
return false;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

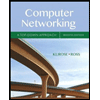
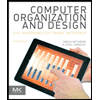
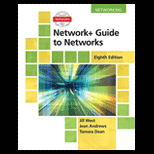
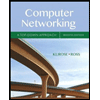
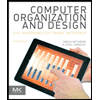
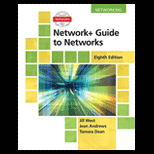
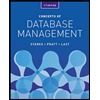
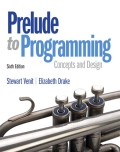
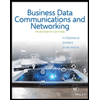