EECE1080C/CS1021C Lab Functions 2 Topics covered: C++ Program Development Practice, Loops, Functions and Arrays B. Taylor Series Use filename: TayLor.cpp Background - You should know from your calculus classes that any well- behaved function can be represented as an infinite series known as a Taylor Series (see: https://www.mathsisfun.com/algebra/taylor-series.html).The focus of this lab element is to compute trigonometric function values for sin and cos by explicitly using their (truncated) Taylor (actually, McLaurin) Series representations. These are given by sin(x) = x - x³/3! + x°/5! - x'/7!... cos(x) = 1 - x²/2! + x*/4! - x*/6!... %3D Notice that sin(x) has odd exponent values and cos(x) has even exponent values. Later on, in your math classes you will learn about odd and even functions and their properties. sin is an odd function and cos is an even function. When you call a math function in your code, say, for example, log10(), your computer actually gets the result by constructing and evaluating a truncated Taylor Series for the function. This lab element requires the development of several functions. Prototypes and a brief description are given in the following. • int factorial(const int &); - use function from Part A double degreesTORadians(const double &); - As the name implies, this function converts the angle value which is input with units of degrees to radians. Recall, radians = degrees(7/180.) • double mySine(const double &, int, double 8); - This function takes 3 arguments and returns a type double value. The return value and arguments are: o [return type double] - the function returns the truncated Taylor Series sum which is the evaluation of sin(x), where x is the first argument (see the following) o const double &x - angle value in radians int i - position of term in Taylor series that is to be assigned to next argument double &result ith term (2nd argument) in truncated Taylor Series for
EECE1080C/CS1021C Lab Functions 2 Topics covered: C++ Program Development Practice, Loops, Functions and Arrays B. Taylor Series Use filename: TayLor.cpp Background - You should know from your calculus classes that any well- behaved function can be represented as an infinite series known as a Taylor Series (see: https://www.mathsisfun.com/algebra/taylor-series.html).The focus of this lab element is to compute trigonometric function values for sin and cos by explicitly using their (truncated) Taylor (actually, McLaurin) Series representations. These are given by sin(x) = x - x³/3! + x°/5! - x'/7!... cos(x) = 1 - x²/2! + x*/4! - x*/6!... %3D Notice that sin(x) has odd exponent values and cos(x) has even exponent values. Later on, in your math classes you will learn about odd and even functions and their properties. sin is an odd function and cos is an even function. When you call a math function in your code, say, for example, log10(), your computer actually gets the result by constructing and evaluating a truncated Taylor Series for the function. This lab element requires the development of several functions. Prototypes and a brief description are given in the following. • int factorial(const int &); - use function from Part A double degreesTORadians(const double &); - As the name implies, this function converts the angle value which is input with units of degrees to radians. Recall, radians = degrees(7/180.) • double mySine(const double &, int, double 8); - This function takes 3 arguments and returns a type double value. The return value and arguments are: o [return type double] - the function returns the truncated Taylor Series sum which is the evaluation of sin(x), where x is the first argument (see the following) o const double &x - angle value in radians int i - position of term in Taylor series that is to be assigned to next argument double &result ith term (2nd argument) in truncated Taylor Series for
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
C++
![ЕЕСE1080C/CS1021C
Lab Functions 2
Topics covered: C++ Program Development Practice, Loops, Functions and Arrays
в. Таylor Series
Use filename: Taylor.cpp
Background - You should know from your calculus classes that any well-
behaved function can be represented as an infinite series known as a Taylor
Series (see: https://www.mathsisfun.com/algebra/taylor-series.html).The
focus of this lab element is to compute trigonometric function values for
sin and cos by explicitly using their (truncated) Taylor (actually,
McLaurin) Series representations. These are given by
sin(x) = x - x³/3! + x$/5! - x'/7!...
cos (x) = 1 - x²/2! + x*/4! - x6/6!...
Notice that sin(x) has odd exponent values and cos(x) has even exponent
values. Later on, in your math classes you will learn about odd and even
functions and their properties. sin is an odd function and cos is an even
function. When you call a math function in your code, say, for example,
log10(), your computer actually gets the result by constructing and
evaluating a truncated Taylor Series for the function.
This lab element requires the development of several functions. Prototypes
and a brief description are given in the following.
• int factorial (const int &); - use function from Part A
• double degrees ToRadians(const double &); - As the name implies, this
function converts the angle value which is input with units of degrees to
radians. Recall, radians = degrees ( 7/180.)
• double mySine(const double &, int, double &); - This function takes 3
arguments and returns a type double value. The return value and arguments
are:
o [return type double] - the function returns the truncated Taylor
Series sum which is the evaluation of sin(x), where x is the first
argument (see the following)
o const double &x - angle value in radians
o int i - position of term in Taylor series that is to be assigned to
next argument
o double &result
sin(x). This term is effectively returned to main() since it is called
by reference. For example, the function call:
ith term (2nd argument) in truncated Taylor Series for
double sinevalue = mySine(PI/4., 3, seriesTerm); // 2nd term
Loaded into seriesTerm](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F217f4d7c-fdc3-480b-ac26-bc970533e530%2Fbc4f4de8-48df-407e-906b-39164acb3079%2F3qhb1c3_processed.png&w=3840&q=75)
Transcribed Image Text:ЕЕСE1080C/CS1021C
Lab Functions 2
Topics covered: C++ Program Development Practice, Loops, Functions and Arrays
в. Таylor Series
Use filename: Taylor.cpp
Background - You should know from your calculus classes that any well-
behaved function can be represented as an infinite series known as a Taylor
Series (see: https://www.mathsisfun.com/algebra/taylor-series.html).The
focus of this lab element is to compute trigonometric function values for
sin and cos by explicitly using their (truncated) Taylor (actually,
McLaurin) Series representations. These are given by
sin(x) = x - x³/3! + x$/5! - x'/7!...
cos (x) = 1 - x²/2! + x*/4! - x6/6!...
Notice that sin(x) has odd exponent values and cos(x) has even exponent
values. Later on, in your math classes you will learn about odd and even
functions and their properties. sin is an odd function and cos is an even
function. When you call a math function in your code, say, for example,
log10(), your computer actually gets the result by constructing and
evaluating a truncated Taylor Series for the function.
This lab element requires the development of several functions. Prototypes
and a brief description are given in the following.
• int factorial (const int &); - use function from Part A
• double degrees ToRadians(const double &); - As the name implies, this
function converts the angle value which is input with units of degrees to
radians. Recall, radians = degrees ( 7/180.)
• double mySine(const double &, int, double &); - This function takes 3
arguments and returns a type double value. The return value and arguments
are:
o [return type double] - the function returns the truncated Taylor
Series sum which is the evaluation of sin(x), where x is the first
argument (see the following)
o const double &x - angle value in radians
o int i - position of term in Taylor series that is to be assigned to
next argument
o double &result
sin(x). This term is effectively returned to main() since it is called
by reference. For example, the function call:
ith term (2nd argument) in truncated Taylor Series for
double sinevalue = mySine(PI/4., 3, seriesTerm); // 2nd term
Loaded into seriesTerm
![ЕЕСE:1080C/CS1021C
Lab Functions 2
Topics covered: C++ Program Development Practice, Loops, Functions and Arrays
will return the truncated Taylor Series evaluation for sin(T/4) and
assign it to the variable double sinevalue. The third argument,
seriesTerm is passed to mySine by reference. In the body of the
function, the 3rd term (2nd argument = 3) in the Taylor series expansion
[= (1/4)5/5!] is assigned to this argument. Since it is passed by
reference, the value of this term is available in your main() function
in the variable, seriesTerm
o This function sets up a loop to sum up the individual terms in the
Taylor Series. You will need to track the series sum using two
variables in your mySine function: double sum_old = 0., sum_new =
(appropriate initial value here); The variables sum_old and sum_new
must be continually updated as each new term is added to the Taylor
Series
o The Taylor Series is truncated (or stopped) when adding the next term
to series results in a change to the series sum that is less than the
specified tolerance value (=10-6). More explicitly, the Taylor Series
is truncated when fabs(sum_new - sum_old) <= tolerance;
• double myCosine(const double &, int, double &); - This function should be
patterned after your mySine function but should use the Taylor Series for
cos (x)
• main() - You should construct a main() function to test your code. Some
requirements are:
o Set up a loop and all the mySine and myCosine functions for a total of
40 separate angle values starting at -45° and incrementing by +15°
(angle values should be: -45º, -30°, -15°, go, 15°,..., 540º)
o Results should be output in a table format with appropriate
formatting. Output should look something like -
sin()
-0.7071
-0.5000
-0.2588
Degrees
Radians
cos ()
0.7071
0.8660
-45
-0.7854
-0.5236
-30
-15
-0.2618
0.9659
0.0000
0.0000
1.0000
15
0.2618
0.2588
0.9659
30
0.5236
0.5000
0.8660
540
9.4248
-0.0000
-1.0000
Use fixed, setw and setprecision stream manipulators to control
formatting](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F217f4d7c-fdc3-480b-ac26-bc970533e530%2Fbc4f4de8-48df-407e-906b-39164acb3079%2Fc1kllls_processed.png&w=3840&q=75)
Transcribed Image Text:ЕЕСE:1080C/CS1021C
Lab Functions 2
Topics covered: C++ Program Development Practice, Loops, Functions and Arrays
will return the truncated Taylor Series evaluation for sin(T/4) and
assign it to the variable double sinevalue. The third argument,
seriesTerm is passed to mySine by reference. In the body of the
function, the 3rd term (2nd argument = 3) in the Taylor series expansion
[= (1/4)5/5!] is assigned to this argument. Since it is passed by
reference, the value of this term is available in your main() function
in the variable, seriesTerm
o This function sets up a loop to sum up the individual terms in the
Taylor Series. You will need to track the series sum using two
variables in your mySine function: double sum_old = 0., sum_new =
(appropriate initial value here); The variables sum_old and sum_new
must be continually updated as each new term is added to the Taylor
Series
o The Taylor Series is truncated (or stopped) when adding the next term
to series results in a change to the series sum that is less than the
specified tolerance value (=10-6). More explicitly, the Taylor Series
is truncated when fabs(sum_new - sum_old) <= tolerance;
• double myCosine(const double &, int, double &); - This function should be
patterned after your mySine function but should use the Taylor Series for
cos (x)
• main() - You should construct a main() function to test your code. Some
requirements are:
o Set up a loop and all the mySine and myCosine functions for a total of
40 separate angle values starting at -45° and incrementing by +15°
(angle values should be: -45º, -30°, -15°, go, 15°,..., 540º)
o Results should be output in a table format with appropriate
formatting. Output should look something like -
sin()
-0.7071
-0.5000
-0.2588
Degrees
Radians
cos ()
0.7071
0.8660
-45
-0.7854
-0.5236
-30
-15
-0.2618
0.9659
0.0000
0.0000
1.0000
15
0.2618
0.2588
0.9659
30
0.5236
0.5000
0.8660
540
9.4248
-0.0000
-1.0000
Use fixed, setw and setprecision stream manipulators to control
formatting
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
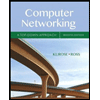
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
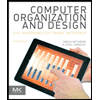
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
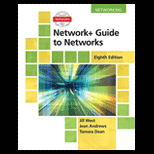
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
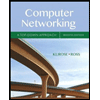
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
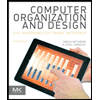
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
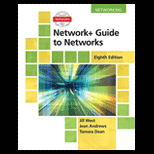
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
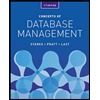
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
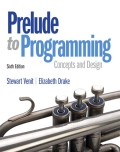
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
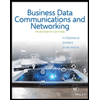
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY