Abstracting Calculations. We can use functions to hide the complexity of a calculation away from the main part of a program. You will design a new version of the program you created below. In this new version, you will create a function for the formula used in that program. Your program shall: Define a function for the formula used in the program with the appropriate parameters, and return value. Use a meaningful name for this function. Call the function in the main part of the program. Run and test the program to make sure it works correctly Summary The program is to take the number of miles driven and the gallons of gas used and then calculate the car's miles-per-gallon using the formula: MPG = Miles driven / Gallons of gas used ''' #variables total_miles_driven = float(input("Enter the no of miles driven: ")) time_driven = float(input("Enter how many hours did it driven: ")) gallon_gas = float(input("Enter the gallons of gas used: ")) #Speed equation speed = float(total_miles_driven) / float(time_driven) #MPG equation MPG = float(total_miles_driven) / float(gallon_gas) #print including speed print("The milesPerGallon of the car is {MPG}".format(MPG=round(MPG, 2))) print("The speed you were traveling is:", speed)
Abstracting Calculations. We can use functions to hide the complexity of a calculation away from the main part of a
You will design a new version of the program you created below. In this new version, you will create a function for the formula used in that program.
Your program shall:
- Define a function for the formula used in the program with the appropriate parameters, and return value.
- Use a meaningful name for this function.
- Call the function in the main part of the program.
- Run and test the program to make sure it works correctly
Summary
The program is to take the number of miles driven and the gallons of gas used
and then calculate the car's miles-per-gallon using the formula:
MPG = Miles driven / Gallons of gas used
'''
#variables
total_miles_driven = float(input("Enter the no of miles driven: "))
time_driven = float(input("Enter how many hours did it driven: "))
gallon_gas = float(input("Enter the gallons of gas used: "))
#Speed equation
speed = float(total_miles_driven) / float(time_driven)
#MPG equation
MPG = float(total_miles_driven) / float(gallon_gas)
#print including speed
print("The milesPerGallon of the car is {MPG}".format(MPG=round(MPG, 2)))
print("The speed you were traveling is:", speed)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

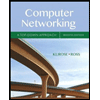
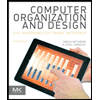
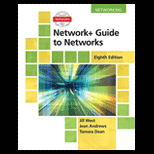
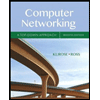
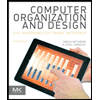
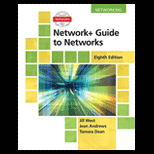
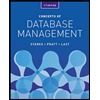
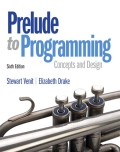
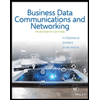