WRITE A CODE IN C++ You work for an analytics organization have been tasked with writing a program that simulate a service desk. This service desk should be able to service 100 customers that can have one of three different priorities (high, medium, and low). The duration for any customer is a random number (between 5 minutes and 8 minutes). Code a modular program that uses parallel arrays to store priority levels and service times for 100 service requests. The program must do the following: The program must first ask the user to run the simulation or quit the program using a menu. This must be done using a function that is called. Upon input of the menu choice, if the user inputs a 2, the program will exit, otherwise if the user enters a 1, the program must begin processing the 100 service requests. The program must use loops and random numbers to generate priority level (high, medium, or low) as well as the service time (between 5 and 8 minutes) for each request in parallel arrays. These arrays must not be declared globally. The following arrays must be declared and populated: priorities[] - an array of type string to hold the priority level for each service request. The size of the array is indicative of the number of requests in the simulation (100). The values in the array represent the level of priority that will associated with the request (high, medium, or low). serviceTimes[] - an array of type int to hold the service times of each service request. The size of the array is indicative of the number of requests in the simulation (100). The values in the array represent the amount of service time associated with the request. There are many ways these arrays can be populated, but as a suggestion, follow this logic: Use a loop that repeats based on the number of service request. Generate a random number for the priority level then associate a string for the value. For example, 1 = high priority, 2 = medium priority, 3 = low priority. Then add that value to the priority array. Use a function to generate the string values. In the same loop, populate the service times array. Generate a random number between 5 and 8 minutes, then add that value to the service times array. Use a function to generate the service times. Once both arrays are populated, the program must display the stats for the service desk. This includes displaying a listing of all service requests generated along with the associated service time for each service request. Then display a count of the number of requests within each priority along with the average service time of requests in each priority level. This must be done by calling a function that accepts both arrays as well as the number of service requests. Once the simulation has finished processing, display the menu and allow for the user to make selections until the user chooses to quit the program via a menu choice entered in step 1 or 2. In addition to the main() function, declare prototypes, write function definitions and correctly call the following functions: mainMenuChoice() - function must allow the user to choose whether or not they will run the simulation by displaying a menu. Validate the user input so that the user must enter either 1 or 2. If the user enters an invalid menu choice, they must be given an unlimited number of chances to enter a valid menu choice. The function must return the validated menu choice. generatePriority() - function must generate a string that represents the priority level of the service request. Each service request can be either high, medium, or low priority. (Your program should randomly allocate this priority to each service.) The function must return a string that represents the priority level of the service request. generateServiceTime() – function must randomly generate and return a value that represents the service time for each request. Each service request can need any time to be serviced between 5 and 8 minutes. (Your program must randomly allocate an integer time to each service request.) serviceDeskStats() – function must accept the arrays that contain the priorities, service times, as well as an integer that represents the number of requests in the simulation as arguments. Then the function must display a list of all of the service requests generated in the simulation with their priority level and the service time for each request. Finally, display the total amount of requests within each priority level along with the average service time within each priority level. Other Notes: Program Output and Prompts MUST MATCH the formatting of the sample runs EXACTLY. SAMPLE BELOW
WRITE A CODE IN C++ You work for an analytics organization have been tasked with writing a program that simulate a service desk. This service desk should be able to service 100 customers that can have one of three different priorities (high, medium, and low). The duration for any customer is a random number (between 5 minutes and 8 minutes). Code a modular program that uses parallel arrays to store priority levels and service times for 100 service requests. The program must do the following: The program must first ask the user to run the simulation or quit the program using a menu. This must be done using a function that is called. Upon input of the menu choice, if the user inputs a 2, the program will exit, otherwise if the user enters a 1, the program must begin processing the 100 service requests. The program must use loops and random numbers to generate priority level (high, medium, or low) as well as the service time (between 5 and 8 minutes) for each request in parallel arrays. These arrays must not be declared globally. The following arrays must be declared and populated: priorities[] - an array of type string to hold the priority level for each service request. The size of the array is indicative of the number of requests in the simulation (100). The values in the array represent the level of priority that will associated with the request (high, medium, or low). serviceTimes[] - an array of type int to hold the service times of each service request. The size of the array is indicative of the number of requests in the simulation (100). The values in the array represent the amount of service time associated with the request. There are many ways these arrays can be populated, but as a suggestion, follow this logic: Use a loop that repeats based on the number of service request. Generate a random number for the priority level then associate a string for the value. For example, 1 = high priority, 2 = medium priority, 3 = low priority. Then add that value to the priority array. Use a function to generate the string values. In the same loop, populate the service times array. Generate a random number between 5 and 8 minutes, then add that value to the service times array. Use a function to generate the service times. Once both arrays are populated, the program must display the stats for the service desk. This includes displaying a listing of all service requests generated along with the associated service time for each service request. Then display a count of the number of requests within each priority along with the average service time of requests in each priority level. This must be done by calling a function that accepts both arrays as well as the number of service requests. Once the simulation has finished processing, display the menu and allow for the user to make selections until the user chooses to quit the program via a menu choice entered in step 1 or 2. In addition to the main() function, declare prototypes, write function definitions and correctly call the following functions: mainMenuChoice() - function must allow the user to choose whether or not they will run the simulation by displaying a menu. Validate the user input so that the user must enter either 1 or 2. If the user enters an invalid menu choice, they must be given an unlimited number of chances to enter a valid menu choice. The function must return the validated menu choice. generatePriority() - function must generate a string that represents the priority level of the service request. Each service request can be either high, medium, or low priority. (Your program should randomly allocate this priority to each service.) The function must return a string that represents the priority level of the service request. generateServiceTime() – function must randomly generate and return a value that represents the service time for each request. Each service request can need any time to be serviced between 5 and 8 minutes. (Your program must randomly allocate an integer time to each service request.) serviceDeskStats() – function must accept the arrays that contain the priorities, service times, as well as an integer that represents the number of requests in the simulation as arguments. Then the function must display a list of all of the service requests generated in the simulation with their priority level and the service time for each request. Finally, display the total amount of requests within each priority level along with the average service time within each priority level. Other Notes: Program Output and Prompts MUST MATCH the formatting of the sample runs EXACTLY. SAMPLE BELOW
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
WRITE A CODE IN C++
You work for an analytics organization have been tasked with writing a
Code a modular program that uses parallel arrays to store priority levels and service times for 100 service requests.
The program must do the following:
- The program must first ask the user to run the simulation or quit the program using a menu. This must be done using a function that is called.
- Upon input of the menu choice, if the user inputs a 2, the program will exit, otherwise if the user enters a 1, the program must begin processing the 100 service requests.
- The program must use loops and random numbers to generate priority level (high, medium, or low) as well as the service time (between 5 and 8 minutes) for each request in parallel arrays. These arrays must not be declared globally.
- The following arrays must be declared and populated:
- priorities[] - an array of type string to hold the priority level for each service request. The size of the array is indicative of the number of requests in the simulation (100). The values in the array represent the level of priority that will associated with the request (high, medium, or low).
- serviceTimes[] - an array of type int to hold the service times of each service request. The size of the array is indicative of the number of requests in the simulation (100). The values in the array represent the amount of service time associated with the request.
- There are many ways these arrays can be populated, but as a suggestion, follow this logic:
- Use a loop that repeats based on the number of service request. Generate a random number for the priority level then associate a string for the value. For example, 1 = high priority, 2 = medium priority, 3 = low priority. Then add that value to the priority array. Use a function to generate the string values.
- In the same loop, populate the service times array. Generate a random number between 5 and 8 minutes, then add that value to the service times array. Use a function to generate the service times.
- Once both arrays are populated, the program must display the stats for the service desk. This includes displaying a listing of all service requests generated along with the associated service time for each service request. Then display a count of the number of requests within each priority along with the average service time of requests in each priority level. This must be done by calling a function that accepts both arrays as well as the number of service requests.
- Once the simulation has finished processing, display the menu and allow for the user to make selections until the user chooses to quit the program via a menu choice entered in step 1 or 2.
In addition to the main() function, declare prototypes, write function definitions and correctly call the following functions:
- mainMenuChoice() - function must allow the user to choose whether or not they will run the simulation by displaying a menu. Validate the user input so that the user must enter either 1 or 2. If the user enters an invalid menu choice, they must be given an unlimited number of chances to enter a valid menu choice. The function must return the validated menu choice.
- generatePriority() - function must generate a string that represents the priority level of the service request. Each service request can be either high, medium, or low priority. (Your program should randomly allocate this priority to each service.) The function must return a string that represents the priority level of the service request.
- generateServiceTime() – function must randomly generate and return a value that represents the service time for each request. Each service request can need any time to be serviced between 5 and 8 minutes. (Your program must randomly allocate an integer time to each service request.)
- serviceDeskStats() – function must accept the arrays that contain the priorities, service times, as well as an integer that represents the number of requests in the simulation as arguments. Then the function must display a list of all of the service requests generated in the simulation with their priority level and the service time for each request. Finally, display the total amount of requests within each priority level along with the average service time within each priority level.
Other Notes:
- Program Output and Prompts MUST MATCH the formatting of the sample runs EXACTLY.
- SAMPLE BELOW

Transcribed Image Text:C:\Users Owner Documents CPCC\Summer 2022 CSC 134 Updates Final Programming Exam Demo.exe
******Welcome to the Service Desk Simulator ******
Choose from one of the following options:
1. Process 100 Service Desk Requests
2. Exit
Enter your choice (1 or 2): 3
ERROR: INVALID MENU CHOICE
Choose from one of the following options:
1. Process 100 Service Desk Requests
2. Exit
Enter your choice (1 or 2): 0
ERROR: INVALID MENU CHOICE
Choose from one of the following options:
1. Process 100 Service Desk Requests
2. Exit
Enter your choice (1 or 2): 1
Type here to search
H
70°F Clear
I
(49)
O
12:12 AM
4/29/2022

Transcribed Image Text:C:\Users Owner Documents CPCC Summer 2022 CSC 134 Updates Final Programming Exam Demo.exe
Service Request #67
low
high
low
high
high
low
medium
high
medium
medium
medium
low
low
low
Service Request #68
Service Request #69
Service Request #70
Service Request #71
Service Request #72
Service Request #73
Service Request #74
Service Request #75
Service Request #76
Service Request #77
Service Request #78
Service Request #79
Service Request #80
Service Request #81
Service Request #82
Service Request #83
Service Request #84
Service Request #85
Service Request #86
Service Request #87
Service Request #38
Service Request #89
Service Request #90
Service Request #91
Service Request #92
Service Request #93
Service Request #94
Service Request #95
Service Request #96
Service Request #97
Service Request #98
Service Request #99
Service Request #100
high
low
medium
high
medium
high
medium
low
low
low
high
medium
low
low
high
medium
low
medium
low
high
Stats:
5 minutes
7 minutes
S minutes
6 minutes
8 minutes
8 minutes
6 minutes
7 minutes
5 minutes
7 minutes
7 minutes
5 minutes
Enter your choice (1 or 2): -
Type here to search
8 minutes
8 minutes
8 minutes
6 minutes
8 minutes
6 minutes
7 minutes
5 minutes
5 minutes
6 minutes
8 minutes
5 minutes
7 minutes
8 minutes
7 minutes
5 minutes
8 minutes
6 minutes
8 minutes
6 minutes
6 minutes
7 minutes
Total of High Priority Requests: 26
Average service time of the High Priority Requests: 6.73 minutes
Total of Medium Priority Requests: 38
Average service time of the Medion Priority Requests: 6.45 minutes
Total # of Low Priority Requests: 36
Average service time of the Low Priority Requests: 6.47 minutes
***Welcome to the Service Desk Simulator ******
Choose from one of the following options:
1. Process 100 Service Desk Requests
2. Exit
H
70°F Clear
I
O
12:12 AM
4/29/2022
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
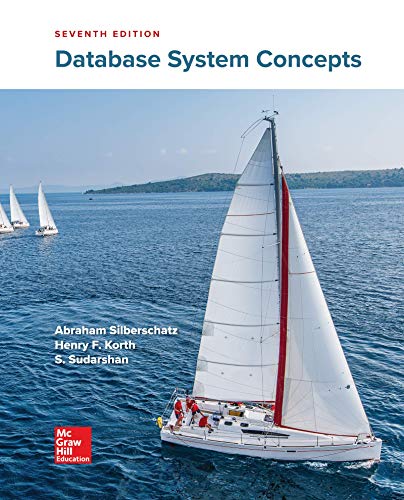
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
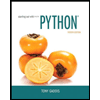
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
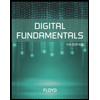
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
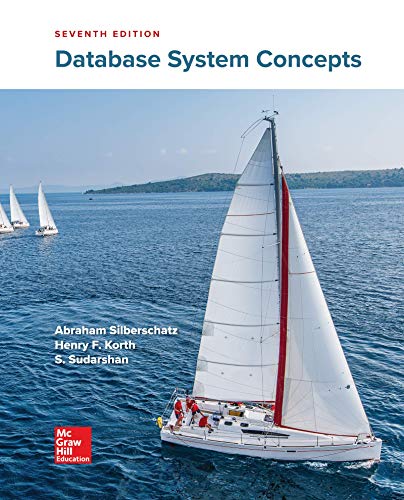
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
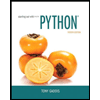
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
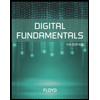
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
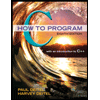
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
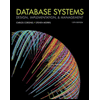
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
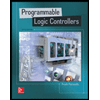
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education