I am trying to create a class called Car in Python with yearModel, make, and speed as attributes. I want to create a two-element constructor that accepts values to set yearModel and make. I need getter/setter functions for each attribute. An accelerate function to increment speed by 5 and a brake function to decrement by 5. I also want a main driver function to accept user input for a year and make of the car. I want to repeatedly call the accelerate and brake functions. I need to output the incrementing speed and decrementing braking speeds. As well as the cars year and make and starting 0 speed. This is the code I have so far, I mainly need help figuring out how to ask for user input at the end. class Car: def __init__(self, yearModel, make, speed): self.yearModel = yearModel self.make = make self.speed = 0 def getyearModel(self): return(self.yearModel) def getmake(self): return(self.make) def getspeed(self): return(self.speed) def accelerate(self): self.speed += 5 def brake(self): self.speed -= 5 def get_speed(self): return self.speed def main(): my_car=Car(yearModel,make, speed)
I am trying to create a class called Car in Python with yearModel, make, and speed as attributes. I want to create a two-element constructor that accepts values to set yearModel and make. I need getter/setter functions for each attribute. An accelerate function to increment speed by 5 and a brake function to decrement by 5. I also want a main driver function to accept user input for a year and make of the car. I want to repeatedly call the accelerate and brake functions. I need to output the incrementing speed and decrementing braking speeds. As well as the cars year and make and starting 0 speed.
This is the code I have so far, I mainly need help figuring out how to ask for user input at the end.
class Car:
def __init__(self, yearModel, make, speed):
self.yearModel = yearModel
self.make = make
self.speed = 0
def getyearModel(self):
return(self.yearModel)
def getmake(self):
return(self.make)
def getspeed(self):
return(self.speed)
def accelerate(self):
self.speed += 5
def brake(self):
self.speed -= 5
def get_speed(self):
return self.speed
def main():
my_car=Car(yearModel,make, speed)

Step by step
Solved in 4 steps with 2 images

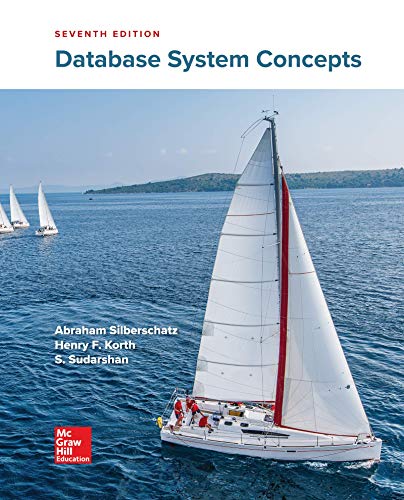
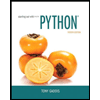
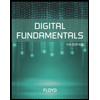
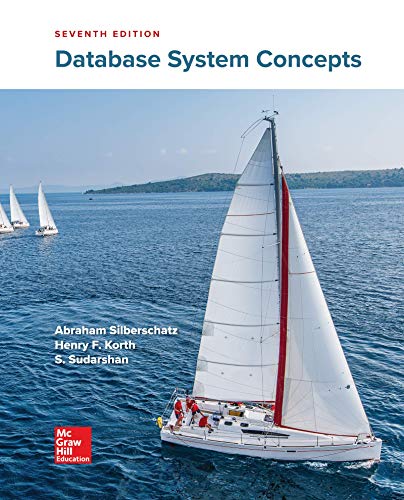
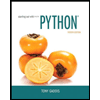
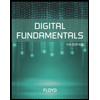
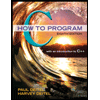
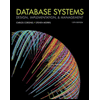
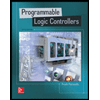