We have noticed that the system floating point number will some times lose information. For this assignment, you will develop your own re number class that can be used in a similar way as system data types such as int and float . In your class rational , you can use two integers a and b to represent a rational number, which is equivalent to a/b . This is because rational number can always be written as a quotient of two integers. Your rational class should support the following functions: • The user can provide two integers to initialize an rational object; If the user does not provide any information, the rational object should be initialized as 0 ; • The user can use the operator +, -, *, and / to operate two rational objects as he/she is using the system defined types; The user can use a member function print() to print the rational number in the form of a/b . For example, the user can do the following with your class rational
We have noticed that the system floating point number will some times lose information. For this assignment, you will develop your own re number class that can be used in a similar way as system data types such as int and float . In your class rational , you can use two integers a and b to represent a rational number, which is equivalent to a/b . This is because rational number can always be written as a quotient of two integers. Your rational class should support the following functions: • The user can provide two integers to initialize an rational object; If the user does not provide any information, the rational object should be initialized as 0 ; • The user can use the operator +, -, *, and / to operate two rational objects as he/she is using the system defined types; The user can use a member function print() to print the rational number in the form of a/b . For example, the user can do the following with your class rational
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
C++

Transcribed Image Text:### Creating a Rational Number Class
We have noticed that the system floating-point number will sometimes lose information. For this assignment, you will develop your own real number class that can be used in a similar way as system data types such as `int` and `float`.
In your class `rational`, you can use two integers `a` and `b` to represent a rational number, which is equivalent to `a/b`. This is because a rational number can always be written as a quotient of two integers. Your `rational` class should support the following functions:
- **Initialization:**
- The user can provide two integers to initialize a `rational` object.
- If the user does not provide any information, the `rational` object should be initialized as `0`.
- **Operations:**
- The user can use the operators `+`, `-`, `*`, and `/` to operate two `rational` objects, as with system-defined types.
- **Printing:**
- The user can use a member function `print()` to print the rational number in the form of `a/b`.
#### Example Usage:
The user can do the following with your class `rational`:
```cpp
rational r1(2,3);
rational r2;
rational r3 = r1 + r2;
rational r4 = r1 * r2;
r3.print();
r4.print();
```
**Expected Output:**
```plaintext
2/3
0
```
#### Tips for Implementation:
- **Hint 1:** Consider the case of dividing by 0. In this case, you can return `0` directly.
- **Hint 2:** Consider reducing the fraction when possible. For instance, if the user creates a rational object as `rational a(2,4)`, you can store `1` and `2` in the object instead of `2` and `4`. You can use the function `__gcd(i1, i2)` to find the greatest common divisor (GCD) of two integers. To use this function, you need to include the header file `<algorithm>`.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
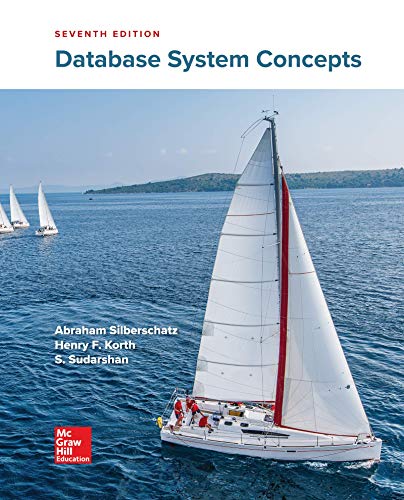
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
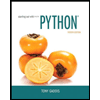
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
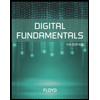
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
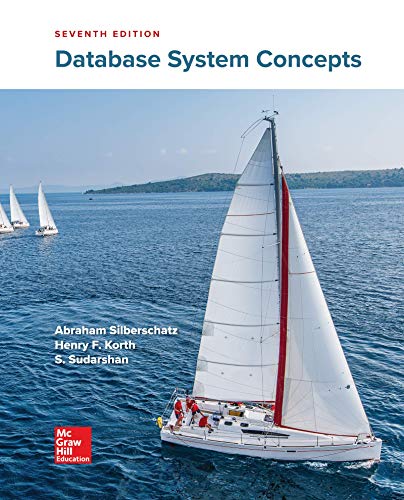
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
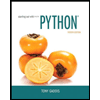
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
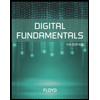
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
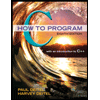
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
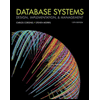
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
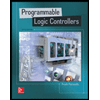
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education