I am having trouble on figuring out how to tackle this test case, below is my code and image one is the test case I am having trouble at(since it will tested automatically, i dont have the testcase.) image two is the main method, plz debug and fix it. Provide the screenshot of the code and output when finsih. import java.util.*; import java.io.*; public class TweetBot { private List tweets; private int index; public TweetBot(List tweets){ if(tweets.size() < 1){ throw new IllegalArgumentException("need contain at least one tweet!"); } this.tweets = new ArrayList<>(tweets); } public int numTweets(){ return tweets.size(); } public void addTweet(String tweet) { tweets.add(tweet); if (tweets.size() == 1) { index = 0; } } public String nextTweet() { if (tweets.size() == 1) { index = 0; return tweets.get(0); } else { if (index == tweets.size()) { index = 0; } String tweet = tweets.get(index); index++; return tweet; } } public void removeTweet(String tweet) { int tweetIndex = tweets.indexOf(tweet); if (tweetIndex == -1) { // Tweet was not found in the list, so the state of the TweetBot should be unchanged return; } tweets.remove(tweetIndex); if (index > tweetIndex) { // If the removed tweet was before the current index, shift the index back by 1 index--; } else if (index == tweets.size()) { // If the current index is now out of bounds, set it to the last index in the list index = tweets.size() - 1; } } public void reset(){ index = 0; } } This is the wrong output I got: A tweet about something controversial Remember to vote! Look at this meme :O A tweet about something controversial Remember to vote! Look at this meme :O Hii, How are everyone? A tweet about something controversial Remember to vote! Look at this meme :O The most frequent word is: a //plz ignore this
I am having trouble on figuring out how to tackle this test case, below is my code and image one is the test case I am having trouble at(since it will tested automatically, i dont have the testcase.) image two is the main method, plz debug and fix it. Provide the screenshot of the code and output when finsih.
import java.util.*;
import java.io.*;
public class TweetBot {
private List<String> tweets;
private int index;
public TweetBot(List<String> tweets){
if(tweets.size() < 1){
throw new IllegalArgumentException("need contain at least one tweet!");
}
this.tweets = new ArrayList<>(tweets);
}
public int numTweets(){
return tweets.size();
}
public void addTweet(String tweet) {
tweets.add(tweet);
if (tweets.size() == 1) {
index = 0;
}
}
public String nextTweet() {
if (tweets.size() == 1) {
index = 0;
return tweets.get(0);
} else {
if (index == tweets.size()) {
index = 0;
}
String tweet = tweets.get(index);
index++;
return tweet;
}
}
public void removeTweet(String tweet) {
int tweetIndex = tweets.indexOf(tweet);
if (tweetIndex == -1) {
// Tweet was not found in the list, so the state of the TweetBot should be unchanged
return;
}
tweets.remove(tweetIndex);
if (index > tweetIndex) {
// If the removed tweet was before the current index, shift the index back by 1
index--;
} else if (index == tweets.size()) {
// If the current index is now out of bounds, set it to the last index in the list
index = tweets.size() - 1;
}
}
public void reset(){
index = 0;
}
}
This is the wrong output I got:
A tweet about something controversial
Remember to vote!
Look at this meme :O
A tweet about something controversial
Remember to vote!
Look at this meme :O
Hii, How are everyone?
A tweet about something controversial
Remember to vote!
Look at this meme :O
The most frequent word is: a //plz ignore this
![nextTweet works correctly even after tweets are added
Failed: Make sure that nextTweet returns the most recently added tweet if there was
previously only a single tweet in the bot. ex. If bot=[t1], nextTweet would return t1. If
addTweet(t1), then nextTweet should return t2, not t1 again ==> expected: <lol hi XD>
but was: <Does this work>
x
Show stacktrace](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6c680976-d041-43f6-b2b7-59630778639d%2F1e493c71-179a-498f-adb0-c8afcf27a567%2Fgw4ng6l_processed.png&w=3840&q=75)
![- import java.util.*;
2 import java.io.*;
3
public class TwitterMain {
public static void main(String[] args) throws FileNotFoundException {
Scanner input = new Scanner(new File("tweets.txt")); // Make Scanner over tweet file
List<String> tweets = new ArrayList<>();
while (input.hasNextLine()) { // Add each tweet in file to List
tweets.add(input.nextLine());
}
TweetBot bot = new TweetBot (tweets); // Create TweetBot object with list of tweets
System.out.println(bot.nextTweet()); // should print "tweet about something controversial"
System.out.println(bot.nextTweet());
System.out.println(bot.nextTweet());
// should print "Remember to vote!"
// should print "Look at this meme:0"
System.out.println(bot.nextTweet()); // should print "tweet about something controversial" (because the index has wrapped around)
bot.add Tweet ("This is a new tweet!");
System.out.println(bot.nextTweet()); // should print "This is a new
bot.addTweet ("Hii, How are everyone?");
System.out.println(bot.nextTweet()); // should print "Hii, How are everyone?"
bot.removeTweet ("This is a new tweet!");
a new tweet!"
System.out.println(bot.nextTweet());
System.out.println(bot.nextTweet());
System.out.println(bot.nextTweet());
System.out.println(bot.nextTweet());
Twitter Trends trends = new TwitterTrends (bot); // Create TwitterTrends object](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6c680976-d041-43f6-b2b7-59630778639d%2F1e493c71-179a-498f-adb0-c8afcf27a567%2Fgvgmxpo_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

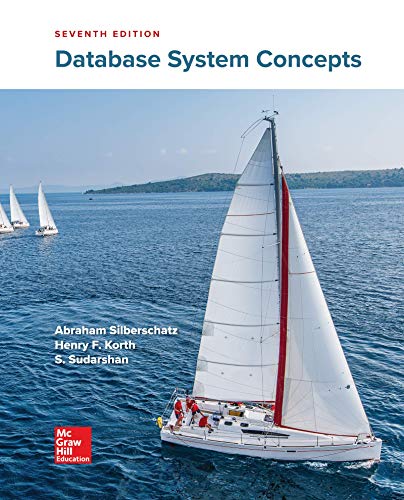
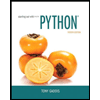
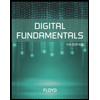
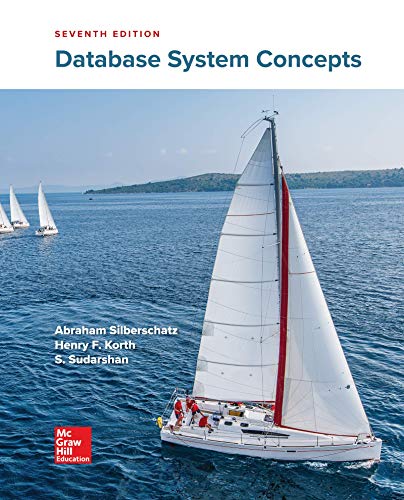
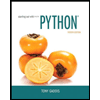
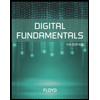
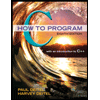
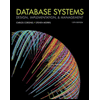
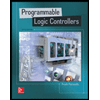