I need help putting this java code into something I can copy/paste. Given the code below, write a Circle class (and save it in a file named Circle.java) that inherits from the Shape class. Include in your Circle class, a single private field double radius. Also include a method void setRadius(double r) (which also sets area) and a method double getRadius() (which also returns the current radius). Change the accessibility modifier for area in the Shape class to be more appropriate for a base class. Make sure that ShapeDriver's main() method executes and produces the following output: Shape: area: 78.53981633974483 radius: 5.0 I also have my code that I worked on as a picture as well. Thanks in advance!
I need help putting this java code into something I can copy/paste.
Given the code below, write a Circle class (and save it in a file named Circle.java) that inherits from the Shape class. Include in your Circle class, a single private field double radius. Also include a method void setRadius(double r) (which also sets area) and a method double getRadius() (which also returns the current radius). Change the accessibility modifier for area in the Shape class to be more appropriate for a base class. Make sure that ShapeDriver's main() method executes and produces the following output:
Shape: area: 78.53981633974483 radius: 5.0
I also have my code that I worked on as a picture as well. Thanks in advance!
![Circle Class
Shape.java:
/**
* Defines a basic shape with just area
*
@author Hyrum D. Carroll
@version 0.2 (10/05/2020)
*
*
*/
public class Shape{
private double area;
public Shape(){ area = 0.0; }
public Shape( double a ){ this.area = a; }
public void setArea( double a ){ area = a; }
public double getArea({ return area; }
public String toString({
return "Shape:\n\tarea: "+ area;
}
}
ShapeDriver.java:
/**
* Create a simple Circle object
@author Hyrum D. Carroll
* @version 0.2 (10/12/2020)
*
*/
public class ShapeDriver{
public static void main( String[] args ){
Circle cir = new Circle( );
cir.setRadius( 5.0 );
System.out.println( cir.toString() );
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2c2de1d1-0a32-4c7c-b3e5-a5cde7e01056%2F6d531d4a-7ce1-403a-8311-25c280e9adac%2Fckqt5b8_processed.png&w=3840&q=75)
![Shape.java:
public class Shape {
private double area;
public Shape({
area = 0.0;
}
public Shape(double a){
this.area = a;
}
public void setArea(double a){
area = a;
}
public double getArea({
return area;
}
public String toString({
return "Shape:\n\tarea: "+ area;
}
}
ShapeDriver.java:
public class ShapeDriver {
public static void main(String[] args) {
Circle cir = new Circle();
cir.setRadius(5.0);
System.out.println(cir.toString() + "In\tradius: "+ cir.getRadius();
Cirlce.java:
public class Circle extends Shape{
private double radius;
private final double PI = 3.17;
public void setRadius(double r){
this.radius = r;
double area = PI*Math.pow(r,2);
setArea(area);
}
public double getRadius() {
return this.radius;
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2c2de1d1-0a32-4c7c-b3e5-a5cde7e01056%2F6d531d4a-7ce1-403a-8311-25c280e9adac%2Fkak2nxm_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

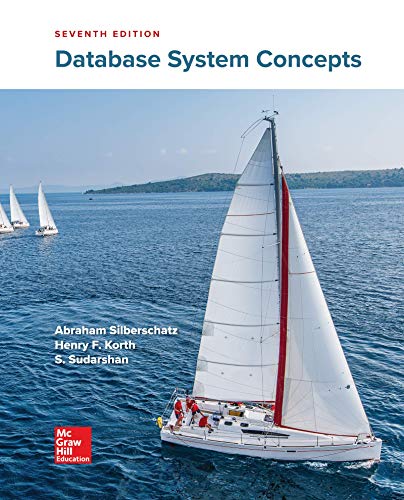
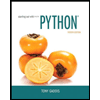
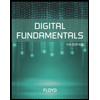
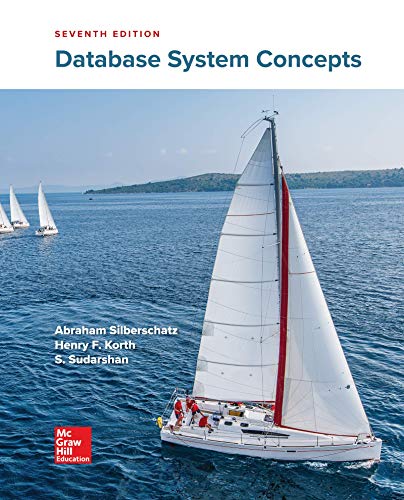
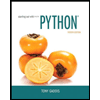
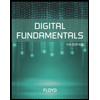
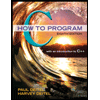
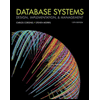
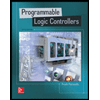