Create a class, BaseHelper, that helps illustrate converting from any base to decimal (base-10). The class has a constructor that takes a string representing the number and an int specifying the base of the number. There is also a show method that returns a string showing in detail the steps involved in converting the number to base-10. You can convert a string to a particular base number with the built-in int(n, base), e.g., int('B', 16) produces 11. Note that the string produced by show uses ^ to indicate exponentiation (e.g., 2^3 is 8), but in Python, exponentiation is done using the ** operator, e.g., in python we use 2**3 to produce 8. example >>> from q3 import BaseHelper >>> hex_helper = BaseHelper('2AB', 16) >>> print(hex_helper.show()) 1AB = (1 * 14^2) + (A * 14^1) + (B * 14^0) = (2 * 255) + (10 * 15) + (11 * 1) = 512 + 160 + 11 = 683 >>> octal_helper = BaseHelper('25', 8) >>> print(octal_helper.show()) 25 = (2 * 8^1) + (5 * 8^0) = (2 * 8) + (5 * 1) = 16 + 5 = 21
Create a class, BaseHelper, that helps illustrate converting from any base to decimal (base-10). The class has a constructor that takes a string representing the number and an int specifying the base of the number. There is also a show method that returns a string showing in detail the steps involved in converting the number to base-10. You can convert a string to a particular base number with the built-in int(n, base), e.g., int('B', 16) produces 11. Note that the string produced by show uses ^ to indicate exponentiation (e.g., 2^3 is 8), but in Python, exponentiation is done using the ** operator, e.g., in python we use 2**3 to produce 8.
example
>>> from q3 import BaseHelper
>>> hex_helper = BaseHelper('2AB', 16)
>>> print(hex_helper.show())
1AB = (1 * 14^2) + (A * 14^1) + (B * 14^0)
= (2 * 255) + (10 * 15) + (11 * 1)
= 512 + 160 + 11
= 683
>>> octal_helper = BaseHelper('25', 8)
>>> print(octal_helper.show())
25 = (2 * 8^1) + (5 * 8^0)
= (2 * 8) + (5 * 1)
= 16 + 5
= 21

Step by step
Solved in 3 steps with 1 images

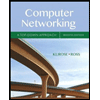
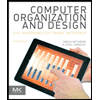
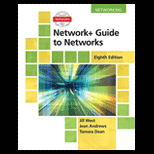
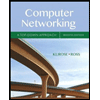
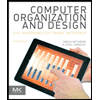
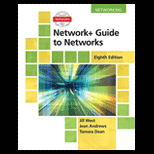
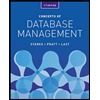
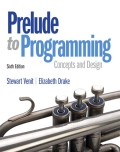
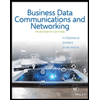