The goal of this is to create two classes BookstoreBook and LibraryBook that both extend the class Book. The class Book is to be made abstract and to have these private attributes: author: String tiltle: String isbn: String To the Book class, add the abstract method: public String getBookType(); - The BookstoreBook has an additional data member to store the price of the book, and whether the book is on sale or not. If a bookstore book is on sale, we need to add the reduction percentage (like .2 off...etc). For that, add double price, boolean onSale and double saleRate as private fields to the BookstoreBook class. Obviously, saleRate is 0.0 when onSale is false. - In the LibraryBook class, we add Subject and callNumber as String. The call number is automatically generated by the following procedure: The callNumber has the format S.XX.YYY.C, where S is the subject code. The list of subject codes is provided at the end of this document, XX is the floor number that is randomly assigned (our library has 15 floors: 01, 02, ...15), YYY are the first three letters of the author’s name (we assume that all names are at least three letters long), and C is the last character of the isbn. For example, Q.09.JON.T is the call number for a Science book that is located on the 9th floor of the library and whose author’s name starts with JON. The isbn of that book ends with the character T. - In each of the three classes mentioned above, add the setters, the getters, at least two constructors (of your choosing). Override the toString method in the class Book to return a string containing the isbn, author and the title. In the BookstoreBook and LibraryBook classes, override the toString to add the additional information to the returned string, using super.toString(). The format of the string returned by the toString methods is shown on the sample run below. Additionally, each of the sub-classes must override getBookType to return “Library Book” or “Bookstore Book.” This method may be used whenever you are in need to tell if a book is a bookstore or a library book. - Feel free to add private methods to any of the classes to avoid code redundancy. - The code should handle up to 100 books. For this, your code must use one array of type Book (not an ArrayList nor any built-in data structure class) in which you reference objects of both classes BookstoreBook and LibraryBook.
How would I create this using Java. Sample run attached in image.
The goal of this is to create two classes BookstoreBook and LibraryBook that both extend the class Book. The class Book is to be made abstract and to have these private attributes:
author: String
tiltle: String
isbn: String
To the Book class, add the abstract method: public String getBookType();
- The BookstoreBook has an additional data member to store the price of the book, and whether the book is on sale or not. If a bookstore book is on sale, we need to add the reduction percentage (like .2 off...etc). For that, add double price, boolean onSale and double saleRate as private fields to the BookstoreBook class. Obviously, saleRate is 0.0 when onSale is false.
- In the LibraryBook class, we add Subject and callNumber as String. The call number is automatically generated by the following procedure:
The callNumber has the format S.XX.YYY.C, where S is the subject code. The list of subject codes is provided at the end of this document, XX is the floor number that is randomly assigned (our library has 15 floors: 01, 02, ...15), YYY are the first three letters of the author’s name (we assume that all names are at least three letters long), and C is the last character of the isbn. For example, Q.09.JON.T is the call number for a Science book that is located on the 9th floor of the library and whose author’s name starts with JON. The isbn of that book ends with the character T.
- In each of the three classes mentioned above, add the setters, the getters, at least two constructors (of your choosing). Override the toString method in the class Book to return a string containing the isbn, author and the title. In the BookstoreBook and LibraryBook classes, override the toString to add the additional information to the returned string, using super.toString(). The format of the string returned by the toString methods is shown on the sample run below. Additionally, each of the sub-classes must override getBookType to return “Library Book” or
“Bookstore Book.” This method may be used whenever you are in need to tell if a book is a bookstore or a library book.
- Feel free to add private methods to any of the classes to avoid code redundancy.
- The code should handle up to 100 books. For this, your code must use one array of type Book (not an ArrayList nor any built-in data structure class) in which you reference objects of both classes BookstoreBook and LibraryBook.
![| Welcome to the book program!
Would you like to create a book object? (yes/no): yEs (yEs, Yes,..., No, NO,nO...etc all
acceptable entries)
Enter the author, title and the isbn of the book separated by /: Ericka Jones/Java made
Easy/458792132
Got it!
Now, tell me if it is a bookstore book or a library book (enter BB for bookstore book or LB for
library book): BSB (Lb, bB...etc all acceptable entries)
Oops! That's not a valid entry. Please try again: Bookstore
Oops! That's not a valid entry. Please try again: bB
Got it!
Enter the list price of JAVA MADE EASY by ERICKA JONES: 14.99
Is it on sale? (yes/no): yes (yEs, Yes,..., No, NO,nO...etc all acceptable entries)
Deduction percentage: 15% (Enter this as a percentage)
Got it!
Here is your bookstore book information
[458792132-JAVA MADE EASY by ERICKA JONES- $14.99 listed for $12.74] (this output is
what the toString() returns)
If Java Made Easy wasn't on sale, your code should print: [458792132-JAVA MADE EASY by
ERICKA JONES, $14.99]
Would you like to create a book object? (yes/no): yeah
Oops! That's not a valid entry. Please try again: yes
Enter the author, title and the isbn of the book separated by /: Eryc Jones/Java as a healing
language/95879213m
Got it!
Now, tell me if it is a bookstore book or a library book (enter BB for bookstore book or LB for
library book): LB
What's the subject: Medicine (MeDiciNE, MEDICINE.... are acceptable entries. See list of
subjects at the end of this document.)
Got it!
Here is your library book information
[958792130-JAVA AS A HEALING LANGUAGE by ERYC JONES-R.09.ERI.M]
Would you like to create a book object? (yes/no): yes
Enter the author, title and the isbn of the book separated by /: Erica Jone/The Art of Java
1958792139
Got it!](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F20023d03-02cd-4467-b86f-33bfcabdc003%2Fa7b10027-d941-4c7a-8cc9-f79a57116a50%2Fld57s4o_processed.png&w=3840&q=75)
![Now, tell me if it is a bookstore book or a library book (enter BB for bookstore book or LB for
library book): LB
What's the subject: Fine art
Oops! That's not a valid entry. Please try again: Fine arts
Got it!
Here is your library book information
[958792139-THE ART OF JAVA by ERICA JONE-N.01.ERI.9]
Would you like to create a book object? (yes/no): no
Sure!
Here are all the books you entered...
Library Books (2)
[958792130-JAVA AS A HEALING LANGUAGE by ERYC JONES-R.09.ERI.M]
[958792139-THE ART OF JAVA by ERICA JONE-N.01.ERI.9]
Bookstore Books (1)
[458792132-JAVA MADE EASY by ERYCKA JONES, $14.99 listed for $12.74]
Would you like to search for a book? (yes/no): yes
Search by isbn, author or title? Author (IsbN, author, title...etc are acceptable entries. Here
assume that the user keys in correct entries)
Enter the first three letters of the author: Eri
We found 1 Library Book (s) and 1 Book Store Book(s):
[458792132-JAVA MADE EASY by ERYCKA JONES, $14.99 listed for $12.74]
[958792139-THE ART OF JAVA by ERICA JONE-N.01.ERI.9]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F20023d03-02cd-4467-b86f-33bfcabdc003%2Fa7b10027-d941-4c7a-8cc9-f79a57116a50%2Fnffjhuf_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

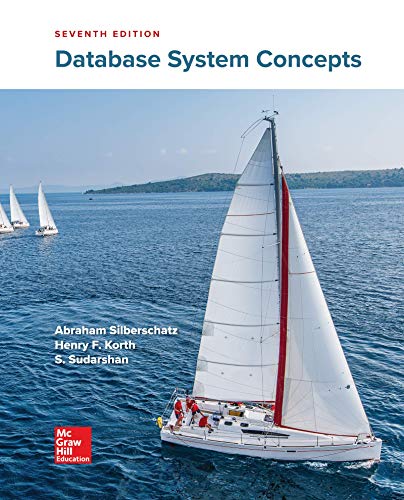
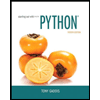
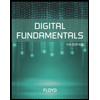
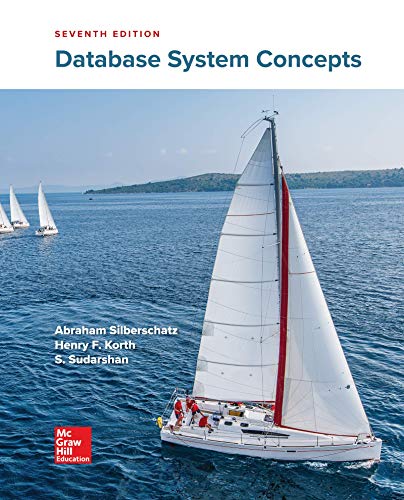
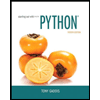
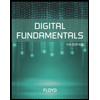
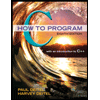
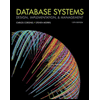
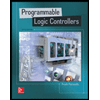