In this java assignment, we will be creating a paystub for an employee using classes, files, getters, and setters. Each file should only have one class and the class should share the same name as the file. We are going to implement the following classes: Employee - This class represent the employee. It needs the following fields exposed via getters and setters: Employee ID (hard code it to 1) First name, last name, middle initial Address, city, zip Phone, email Hourly rate PayPeriod - This class represents an employee's payment information. An employee will eventually have more than one pay period. It needs the following fields exposed via getters and setters: Pay period Id (hard code to 123456) Employee Id Start date, end date Number of hours PayrollManager - This class provides the functionality we need to compute and display the payroll. It should implement the following methods: double CalculateGrossPay (Employee, PayPeriod) - this should return the total gross for the payperiod double CalculateRegularPay (Employee, PayPeriod) - this should return the gross pay at the regular rate for the pay period double CalculateOvertimePay (Employee, PayPeriod) - this should return ONLY the gross overtime for the payperiod void PrintPaystub(Employee, PayPeriod) - this should print out the paystub for the employee for the period. Note: here, we should use the other methods we defined above to implement this method. Your main program should then do the following: Gather input and put it in an instance of Employee Gather payroll info and put it in an instance of PayPeriod (default dates for now) Call PrintPaystub to display it
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
In this java assignment, we will be creating a paystub for an employee using classes, files, getters, and setters. Each file should only have one class and the class should share the same name as the file. We are going to implement the following classes:
Employee - This class represent the employee. It needs the following fields exposed via getters and setters:
- Employee ID (hard code it to 1)
- First name, last name, middle initial
- Address, city, zip
- Phone, email
- Hourly rate
PayPeriod - This class represents an employee's payment information. An employee will eventually have more than one pay period. It needs the following fields exposed via getters and setters:
- Pay period Id (hard code to 123456)
- Employee Id
- Start date, end date
- Number of hours
PayrollManager - This class provides the functionality we need to compute and display the payroll. It should implement the following methods:
- double CalculateGrossPay (Employee, PayPeriod) - this should return the total gross for the payperiod
- double CalculateRegularPay (Employee, PayPeriod) - this should return the gross pay at the regular rate for the pay period
- double CalculateOvertimePay (Employee, PayPeriod) - this should return ONLY the gross overtime for the payperiod
- void PrintPaystub(Employee, PayPeriod) - this should print out the paystub for the employee for the period. Note: here, we should use the other methods we defined above to implement this method.
Your main program should then do the following:
- Gather input and put it in an instance of Employee
- Gather payroll info and put it in an instance of PayPeriod (default dates for now)
- Call PrintPaystub to display it

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

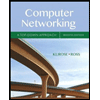
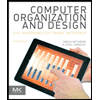
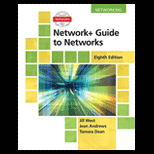
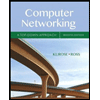
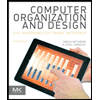
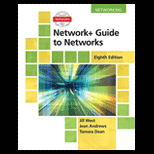
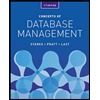
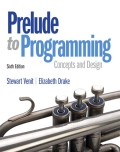
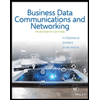