for the class Student that contains 3 attributes: name, studentNumber and course. You are to provide the setter and getter methods as well as the body of the constructor that has a missing body. You are also required to provide an implementation to the method showStudentInformation() so that once it is called it should display the name, stud
Student.java
/*
Given the partial code for the class Student that contains 3 attributes: name, studentNumber and course.
You are to provide the setter and getter methods as well as the body of the constructor that has a missing body.
You are also required to provide an implementation to the method showStudentInformation() so that once it is
called it should display the name, studentNumber and course of the Student object.
*/
public class Student
{
private String name;
private int studentNumber;
private String course;
// this is constructor #1 - no need to add anything here
public Student()
{}
// this is constructor #2 - you have to provide the body of this constructor
public Student(String name, int studentNumber, String course)
{
// complete the body of this constructor
}
// put your setters here
// put your getters here
public void showStudentInformation()
{
// this method should display the name, studentNumber and the course of the student object.
}
}
TestStudent.java
// Module 2 Assignment
public class TestStudent
{
public static void main(String args[])
{
Student s1 = new Student("Lawrence", 1111, "BS Computer Science");
Student s2 = new Student();
Student s3 = new Student();
// set the properties of Student s2, assign a name, studentNumber and course
// set the properties of Student s3, assign a name, studentNumber and course
// print the identity of Student s1
s1.showStudentInformation();
// print the identity of Student s2 by calling the getter methods
// print the identity of Student s3 by calling the showStudentInformation() method
}
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

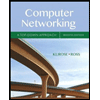
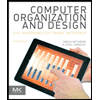
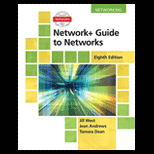
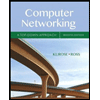
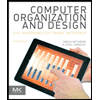
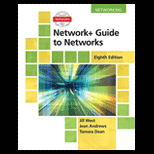
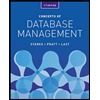
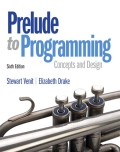
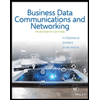