How to implement update function using linked list into my code C++? #include using namespace std; // Node Class class Node { public: int matric; string Name; string course; int result; Node* next; }; // Stores the head of the Linked List Node* head = new Node(); // Check Function to check that if // Record Already Exist or Not bool check(int x) { // Base Case if (head == NULL) return false; Node* t = new Node; t = head; // Traverse the Linked List while (t != NULL) { if (t->matric == x) return true; t = t->next; } return false; } // Function to insert the record void Insert_Record(int matric, string Name, string course, int result) { // if Record Already Exist if (check(matric)) { cout << "Student with this " << "record Already Exists\n"; return; } // Create new Node to Insert Record Node* t = new Node(); t->matric = matric; t->Name = Name; t->course = course; t->result = result; t->next = NULL; // Insert at Begin if (head == NULL || (head->matric >= t->matric)) { t->next = head; head = t; } // Insert at middle or End else { Node* c = head; while (c->next != NULL && c->next->matric < t->matric) { c = c->next; } t->next = c->next; c->next = t; } cout << "Record Inserted " << "Successfully\n"; } // Function to search record for any // students Record with matric number void Search_Record(int matric) { // if head is NULL if (!head) { cout << "No such Record " << "Available\n"; return; } // Otherwise else { Node* p = head; while (p) { if (p->matric == matric) { cout << "Matric number\t" << p->matric << endl; cout << "Name\t\t" << p->Name << endl; cout << "Course\t" << p->course << endl; cout << "Result\t\t" << p->result << endl; return; } p = p->next; } if (p == NULL) cout << "No such Record " << "Available\n"; } } // Function to delete record students // record with given matric number // if it exist int Delete_Record(int matric) { Node* t = head; Node* p = NULL; // Deletion at Begin if (t != NULL && t->matric == matric) { head = t->next; delete t; cout << "Record Deleted " << "Successfully\n"; return 0; } // Deletion Other than Begin while (t != NULL && t->matric != matric) { p = t; t = t->next; } if (t == NULL) { cout << "Record does not Exist\n"; return -1; p->next = t->next; delete t; cout << "Record Deleted " << "Successfully\n"; return 0; } } // Function to display the Student's // Record void Show_Record() { Node* p = head; if (p == NULL) { cout << "No Record " << "Available\n"; } else { cout << "Index\tName\tCourse" << "\tResult\n"; // Until p is not NULL while (p != NULL) { cout << p->matric << " \t" << p->Name << "\t" << p->course << "\t" << p->result << endl; p = p->next; } } } // Driver code int main() { head = NULL; string Name, Course; int matric, result; // Menu-driven program while (true) { cout << "\n\t\tWelcome to Student Record " "Management System\n\n\tPress\n\t1 To " "create a new record\n\t2 To delete a " "student record\n\t3 To search a student " "record\n\t4 To view all students " "record\n\t5 To exit\n"; cout << "\nEnter your Choice\n"; int Choice; // Enter Choice cin >> Choice; if (Choice == 1) { cout << "Enter student name\n"; cin >> Name; cout << "Enter matric number of student\n"; cin >> matric; cout << "Enter student course \n"; cin >> Course; cout << "Enter student result\n"; cin >> result; Insert_Record(matric, Name, Course, result); } else if (Choice == 2) { cout << "Enter Matric Number of Student whose " "record is to be deleted\n"; cin >> matric; Delete_Record(matric); } else if (Choice == 3) { cout << "Enter Matric Number of Student whose " "record you want to Search\n"; cin >> matric; Search_Record(matric); } else if (Choice == 4) { Show_Record(); } else if (Choice == 5) { exit(0); } else { cout << "Invalid Choice " << "Try Again\n"; } } return 0; }
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
How to implement update function using linked list into my code C++?
#include <bits/stdc++.h>
using namespace std;
// Node Class
class Node {
public:
int matric;
string Name;
string course;
int result;
Node* next;
};
// Stores the head of the Linked List
Node* head = new Node();
// Check Function to check that if
// Record Already Exist or Not
bool check(int x)
{
// Base Case
if (head == NULL)
return false;
Node* t = new Node;
t = head;
// Traverse the Linked List
while (t != NULL) {
if (t->matric == x)
return true;
t = t->next;
}
return false;
}
// Function to insert the record
void Insert_Record(int matric, string Name,
string course, int result)
{
// if Record Already Exist
if (check(matric)) {
cout << "Student with this "
<< "record Already Exists\n";
return;
}
// Create new Node to Insert Record
Node* t = new Node();
t->matric = matric;
t->Name = Name;
t->course = course;
t->result = result;
t->next = NULL;
// Insert at Begin
if (head == NULL
|| (head->matric >= t->matric)) {
t->next = head;
head = t;
}
// Insert at middle or End
else {
Node* c = head;
while (c->next != NULL
&& c->next->matric < t->matric) {
c = c->next;
}
t->next = c->next;
c->next = t;
}
cout << "Record Inserted "
<< "Successfully\n";
}
// Function to search record for any
// students Record with matric number
void Search_Record(int matric)
{
// if head is NULL
if (!head) {
cout << "No such Record "
<< "Available\n";
return;
}
// Otherwise
else {
Node* p = head;
while (p) {
if (p->matric == matric) {
cout << "Matric number\t"
<< p->matric << endl;
cout << "Name\t\t"
<< p->Name << endl;
cout << "Course\t"
<< p->course << endl;
cout << "Result\t\t"
<< p->result << endl;
return;
}
p = p->next;
}
if (p == NULL)
cout << "No such Record "
<< "Available\n";
}
}
// Function to delete record students
// record with given matric number
// if it exist
int Delete_Record(int matric)
{
Node* t = head;
Node* p = NULL;
// Deletion at Begin
if (t != NULL
&& t->matric == matric) {
head = t->next;
delete t;
cout << "Record Deleted "
<< "Successfully\n";
return 0;
}
// Deletion Other than Begin
while (t != NULL && t->matric != matric) {
p = t;
t = t->next;
}
if (t == NULL) {
cout << "Record does not Exist\n";
return -1;
p->next = t->next;
delete t;
cout << "Record Deleted "
<< "Successfully\n";
return 0;
}
}
// Function to display the Student's
// Record
void Show_Record()
{
Node* p = head;
if (p == NULL) {
cout << "No Record "
<< "Available\n";
}
else {
cout << "Index\tName\tCourse"
<< "\tResult\n";
// Until p is not NULL
while (p != NULL) {
cout << p->matric << " \t"
<< p->Name << "\t"
<< p->course << "\t"
<< p->result << endl;
p = p->next;
}
}
}
// Driver code
int main()
{
head = NULL;
string Name, Course;
int matric, result;
// Menu-driven program
while (true) {
cout << "\n\t\tWelcome to Student Record "
"Management System\n\n\tPress\n\t1 To "
"create a new record\n\t2 To delete a "
"student record\n\t3 To search a student "
"record\n\t4 To view all students "
"record\n\t5 To exit\n";
cout << "\nEnter your Choice\n";
int Choice;
// Enter Choice
cin >> Choice;
if (Choice == 1) {
cout << "Enter student name\n";
cin >> Name;
cout << "Enter matric number of student\n";
cin >> matric;
cout << "Enter student course \n";
cin >> Course;
cout << "Enter student result\n";
cin >> result;
Insert_Record(matric, Name, Course, result);
}
else if (Choice == 2) {
cout << "Enter Matric Number of Student whose "
"record is to be deleted\n";
cin >> matric;
Delete_Record(matric);
}
else if (Choice == 3) {
cout << "Enter Matric Number of Student whose "
"record you want to Search\n";
cin >> matric;
Search_Record(matric);
}
else if (Choice == 4) {
Show_Record();
}
else if (Choice == 5) {
exit(0);
}
else {
cout << "Invalid Choice "
<< "Try Again\n";
}
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

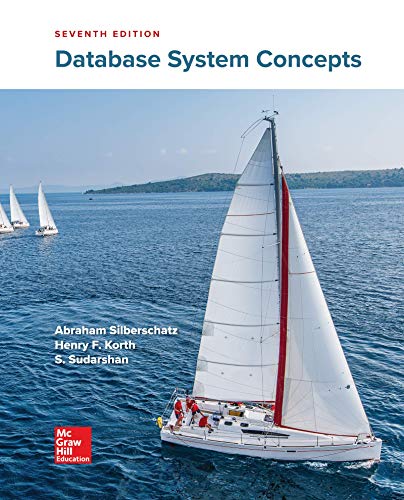
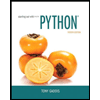
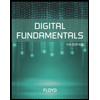
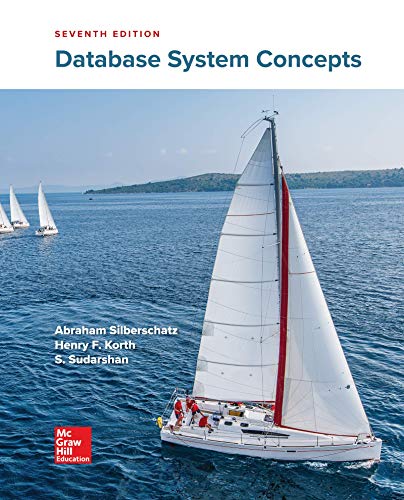
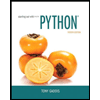
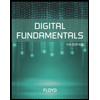
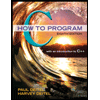
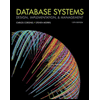
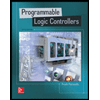