Please fill in the blanks for C /* This program will print students’ information and remove students using linked list: */ #include #include //Declare a struct student (node) with 5 members //each student node contains an ID, an age, graduation year, // 1 pointer points to the next student, and 1 pointer points to the previous student. struct student { int ID; int age; int classOf; __1__ __2__ next; __3__ __4__ prev; }; /* - This function takes the head pointer and iterates through the list to get all 3 integers needed from the user, and save it to our list - Update the pointer to point to the next node. */ __5__ scanLL(__6__ __7__ head) { while(__8__ __9__ __10__) //this condition makes sure the list is not at the end { printf("Lets fill in the information. Enter 3 integers for the student's ID, age, and graduation year: "); scanf("%i %i %i",&__11__, &__12__, &__13__); //in order: ID, age, graduation year head = head->next; } } /*This function prints the list forwards or backwards depends on the flag. If flag is 1, print forward and 0, print backwards. Depends on such flag, we will set our link to move to next or previous student*/ __14__ printLL(__15__ __16__ head, int flag) { while(__17__ __18__ __19__) { printf("ID: %5d.\tAge: %3d.\tClass Of: %4d.\n\n",__20__, __21__, __22__); if(flag == 1) head = __23__; //forwards else head = __24__; //backwards } } /*This function will take an address of the node we need to remove*/ _25__ removeNode(__26__ __27__ stu2Rm) { //removing first node, set its next node as first node if(__28__ == NULL) // check if it's first node __29__ -> __30__ -> __31__ = __32__; //removing last node, set its previous node as last node else if(__33__ == NULL) //check if it's last node __34__ -> __35__ -> __36__ = __37__; //if it's any other node, update both of its previous and next else { __38__ -> __39__ -> __40__ = __41__; //update the previous link Case 3(A) __42__ -> __43__ -> __44__ = __45__; //update the next link Case 3(B) } } int main() { struct student s1, s2, s3, s4; struct __46__ head = __47__; // we'll do in this order s1 -> s2 -> s3 -> s4 __48__ = __49__; //make a link from s1 to s2 __50__ = __51__; //make a link from s2 to s3 __52__ = __53__; //make a link from s3 to s4 __54__ = __55__; //make a link to show s4 is the end __56__ = __57__; //make a link from s4 to s3 __58__ = __59__; //make a link from s3 to s2 __60__ = __61__; //make a link from s2 to s1 __62__ = __63__; //make a link to show s1 is the end scanLL(head); printf("\nPrinting forwards:\n"); printLL(__64__ , __65__); //print back the student's info from the list. //Printing backwards head = __66__; //set the start of the list printf("\nPrinting backwards:\n"); printLL(__67__ , __68__); //Get a student to remove int node2Rm; printf("\nEnter a node to remove. 1 for s1 through 4 for s4.\n"); scanf("%i", &node2Rm); struct student* rmNode; //this is used to check to print again after removing a node switch (node2Rm) { //rmNode will save the address of the node needed to be removed case 1: rmNode = __69__; break; case 2: rmNode =__70__; break; case 3: rmNode = __71__; break; case 4: rmNode = __72__; break; default: printf("Invalid choice. Bye\n"); exit(0); } printf("Removing node: \n"); removeNode(rmNode); //remove the node //Forwards print head = (rmNode == &s1) ? __73__ : __74__; printf("\nPrinting forwards AFTER removing a node:\n"); printLL(__75__,__76__); //Backwards print head = (rmNode == &s4) ? __77__ : __78__; printf("\nPrinting backwards AFTER removing a node:\n"); printLL(__79__,__80__); return 0
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Please fill in the blanks for C
/* This program will print students’ information and remove students using linked list: */
#include<stdio.h>
#include<stdlib.h>
//Declare a struct student (node) with 5 members
//each student node contains an ID, an age, graduation year,
// 1 pointer points to the next student, and 1 pointer points to the previous student.
struct student
{
int ID;
int age;
int classOf;
__1__ __2__ next;
__3__ __4__ prev;
};
/* - This function takes the head pointer and iterates through the list to get all 3 integers needed from the user, and save it to our list
- Update the pointer to point to the next node. */
__5__ scanLL(__6__ __7__ head)
{
while(__8__ __9__ __10__) //this condition makes sure the list is not at the end
{
printf("Lets fill in the information. Enter 3 integers for the student's ID, age, and graduation year: ");
scanf("%i %i %i",&__11__, &__12__, &__13__); //in order: ID, age, graduation year
head = head->next;
}
}
/*This function prints the list forwards or backwards depends on the flag.
If flag is 1, print forward and 0, print backwards.
Depends on such flag, we will set our link to move to next or previous student*/
__14__ printLL(__15__ __16__ head, int flag)
{
while(__17__ __18__ __19__)
{
printf("ID: %5d.\tAge: %3d.\tClass Of: %4d.\n\n",__20__, __21__, __22__);
if(flag == 1) head = __23__; //forwards
else head = __24__; //backwards
}
}
/*This function will take an address of the node we need to remove*/
_25__ removeNode(__26__ __27__ stu2Rm)
{
//removing first node, set its next node as first node
if(__28__ == NULL) // check if it's first node
__29__ -> __30__ -> __31__ = __32__;
//removing last node, set its previous node as last node
else if(__33__ == NULL) //check if it's last node
__34__ -> __35__ -> __36__ = __37__;
//if it's any other node, update both of its previous and next
else
{
__38__ -> __39__ -> __40__ = __41__; //update the previous link Case 3(A)
__42__ -> __43__ -> __44__ = __45__; //update the next link Case 3(B)
}
}
int main()
{
struct student s1, s2, s3, s4;
struct __46__ head = __47__;
// we'll do in this order s1 -> s2 -> s3 -> s4
__48__ = __49__; //make a link from s1 to s2
__50__ = __51__; //make a link from s2 to s3
__52__ = __53__; //make a link from s3 to s4
__54__ = __55__; //make a link to show s4 is the end
__56__ = __57__; //make a link from s4 to s3
__58__ = __59__; //make a link from s3 to s2
__60__ = __61__; //make a link from s2 to s1
__62__ = __63__; //make a link to show s1 is the end
scanLL(head);
printf("\nPrinting forwards:\n");
printLL(__64__ , __65__); //print back the student's info from the list.
//Printing backwards
head = __66__; //set the start of the list
printf("\nPrinting backwards:\n");
printLL(__67__ , __68__);
//Get a student to remove
int node2Rm;
printf("\nEnter a node to remove. 1 for s1 through 4 for s4.\n");
scanf("%i", &node2Rm);
struct student* rmNode; //this is used to check to print again after removing a node
switch (node2Rm)
{
//rmNode will save the address of the node needed to be removed
case 1:
rmNode = __69__;
break;
case 2:
rmNode =__70__;
break;
case 3:
rmNode = __71__;
break;
case 4:
rmNode = __72__;
break;
default:
printf("Invalid choice. Bye\n");
exit(0);
}
printf("Removing node: \n");
removeNode(rmNode); //remove the node
//Forwards print
head = (rmNode == &s1) ? __73__ : __74__;
printf("\nPrinting forwards AFTER removing a node:\n");
printLL(__75__,__76__);
//Backwards print
head = (rmNode == &s4) ? __77__ : __78__;
printf("\nPrinting backwards AFTER removing a node:\n");
printLL(__79__,__80__);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

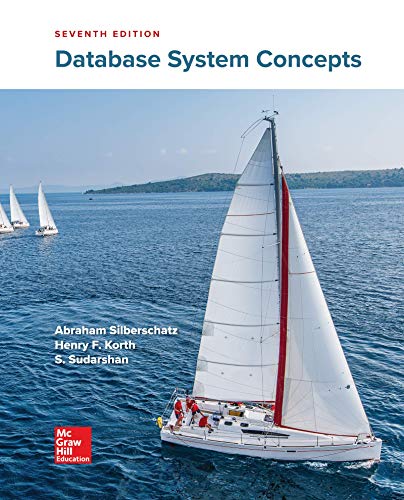
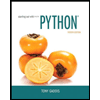
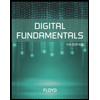
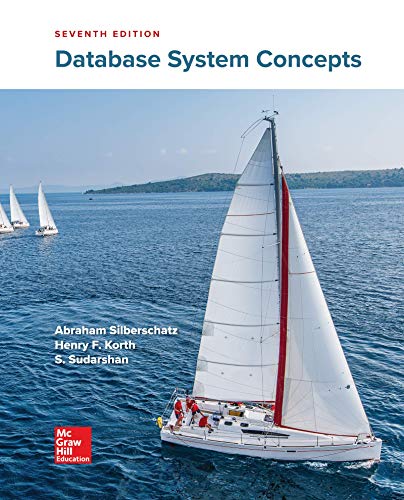
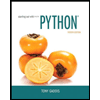
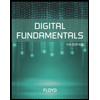
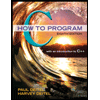
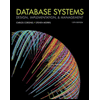
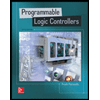