#include using namespace std; #define SIZE 5 //creating the queue using array int A[SIZE]; int front = -1; int rear = -1; //function to check if the queue is empty bool isempty() { if(front == -1 && rear == -1) return true; else return false; } //function to enter elements in queue void enqueue ( int value ) { //if queue is full if ((rear + 1)%SIZE == front) cout<<"Queue is full \n"; else { //now the first element is inserted if( front == -1) front = 0; //inserting element at rear end rear = (rear+1)%SIZE; A[rear] = value; } } //function to remove elements from queue void dequeue ( ) { if( isempty() ) cout<<"Queue is empty\n"; else //only one element if( front == rear ) front = rear = -1; else front = ( front + 1)%SIZE; } //function to show the element at front void showfront() { if( isempty()) cout<<"Queue is empty\n"; else cout<<"element at front is:"<
#include <iostream>
using namespace std;
#define SIZE 5
//creating the queue using array
int A[SIZE];
int front = -1;
int rear = -1;
//function to check if the queue is empty
bool isempty() {
if(front == -1 && rear == -1)
return true;
else
return false;
}
//function to enter elements in queue
void enqueue ( int value ) {
//if queue is full
if ((rear + 1)%SIZE == front)
cout<<"Queue is full \n";
else {
//now the first element is inserted
if( front == -1)
front = 0;
//inserting element at rear end
rear = (rear+1)%SIZE;
A[rear] = value;
}
}
//function to remove elements from queue
void dequeue ( ) {
if( isempty() )
cout<<"Queue is empty\n";
else
//only one element
if( front == rear )
front = rear = -1;
else
front = ( front + 1)%SIZE;
}
//function to show the element at front
void showfront() {
if( isempty())
cout<<"Queue is empty\n";
else
cout<<"element at front is:"<<A[front];
}
//function to display the queue
void displayQueue() {
if(isempty())
cout<<"Queue is empty\n";
else {
int i;
if( front <= rear ) {
for( i=front ; i<= rear ; i++)
cout<<A[i]<<" ";
}
else {
i=front;
while( i < SIZE) {
cout<<A[i]<<" ";
i++;
}
i=0;
while( i <= rear)
{
cout<<A[i]<<" ";
i++;
}
}
}
}
//function to check if Queue is empty
// emptyCheck(){
// }
//function to check if Queue is full
//function to purge the Queue
void purge_queue(){
cout<<"Deleting the entire queue..."<<endl;
delete[] isempty();
exit(1);
}
This is a Queue Program using Circular Array. I would like to know how can I implement the rest of these functions :
- Function to check if the Queue is empty?
- Function to check if the Queue is Full
- Function to purge or Destroy the Queue

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

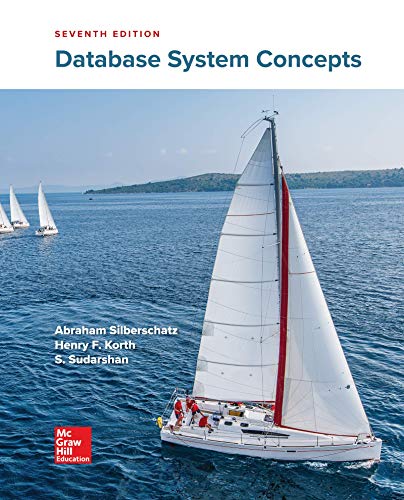
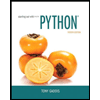
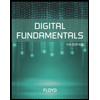
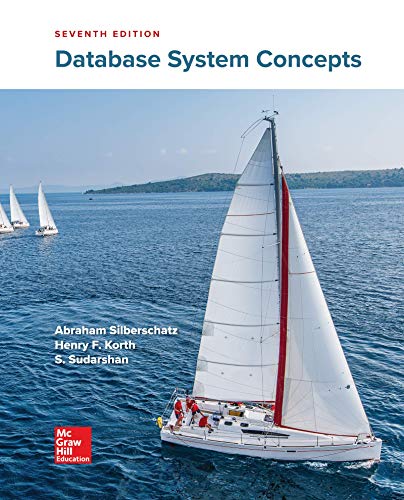
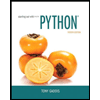
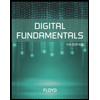
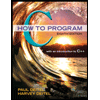
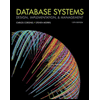
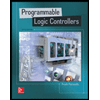