Hi. I made a code but I have to change it. These are the requirements for the program code • Safe entry • No magic numbers • No global variables • File management must be in a separate c-file - I HAVEN'T DONE THIS AND I NEED HELP • All standard header files, type declarations, function prototypes etc. must be included a custom header file - I HAVEN'T DONE THIS EITHER • Functions must not be “too long and when the user chooses to quit "exit", the register must be written to a file - i haven't done this So basically I need to change the code so that it fits the requirements #include #include #include #include #define STR_LANGD 30 #define VEHICLE 10 #define NUMBEROF_PEOPLE 10 typedef struct { char name[STR_LANGD]; int age; } Person; typedef struct { Person person; char model[STR_LANGD]; char carbrand[STR_LANGD]; char registrationnumber[STR_LANGD]; } Vehicle; void initVehicle(Vehicle myVehicle[]) { int i; for (i = 0; i < VEHICLE; i++) { myVehicle[i].person.age = 0; } } int menu() { int choice = 0; printf ("1. Add a vehicle. \n"); printf ("2. Remove a vehicle \n"); printf ("3. Sort by car brand. \n"); printf ("4. Print vehicle information.\n"); printf ("5. Print the entire vehicle register. \n"); printf ("0. Exit. \n"); scanf("%d", &choice); return choice; } void AddVehicle(Vehicle myVehicle[]) { Vehicle temp; int i; fflush(stdin); printf("Enter name: \n"); scanf("%s", temp.person.name); printf("Enter personens ålder: \n"); scanf("%d", &temp.person.age); printf("Enter Vehiclestyp: \n"); scanf("%s", temp.model); printf("Enter car brand: \n"); scanf("%s", temp.carbrand); printf("Enter registration number: "); scanf("%s", temp.registrationnumber); for (i = 0; i < VEHICLE; i++) { if (myVehicle[i].person.age == 0) { myVehicle[i] = temp; break; } } } void showVehicle(Vehicle myVehicle[]) { int i; printf("\nAll vehicle in database\n"); printf("-------------------\n"); for (i = 0; i < VEHICLE; i++) { if (myVehicle[i].person.age != 0) { printf("Place: %d\n", i); printf("Name: "); puts(myVehicle[i].person.name); printf("Ålder på ägaren: %d\n", myVehicle[i].person.age); printf("Car model: %s\n", myVehicle[i].model); printf("Car brand: %s\n", myVehicle[i].carbrand); printf("Registration number: %s\n\n", myVehicle[i].registrationnumber); } } } void DeleteVehicle(Vehicle myVehicle[VEHICLE]) { int temp; printf("På vilken plats (0-10) vill du ta bort Vehicleinfo?\nPlats: "); scanf("%d", &temp); if (myVehicle[temp].person.age != 0) { myVehicle[temp].person.age = 0; } } void printinfo(Vehicle myVehicle[VEHICLE]) { int temp; printf("På vilken plats (0-10) vill du se Vehicleinfo?\nPlats: "); scanf("%d", &temp); if (myVehicle[temp].person.age != 0) { printf("\n------------Vehicleinfo-----------\n"); printf("Plats: %d", temp); printf("name: %s\n", myVehicle[temp].person.name); printf("Ålder på ägaren: %d\n", myVehicle[temp].person.age); printf("Car model: %s\n", myVehicle[temp].model); printf("Car brand: %s\n", myVehicle[temp].carbrand); printf("Registration number: %s\n", myVehicle[temp].registrationnumber); } } void carSort(Vehicle myVehicle[VEHICLE]) { int i; int j; Vehicle temp; for (i = 1; i < VEHICLE; i++) { for (j = 0; j < VEHICLE - i; j++) { if (myVehicle[j].carbrand[0] > myVehicle[j+1].carbrand[0]) { temp = myVehicle[j]; myVehicle[j] = myVehicle[j+1]; myVehicle[j+1] = temp; } } } } int main() //main: här startar alla C-program { Vehicle myVehicle[VEHICLE]; int menuchoice = 0; initVehicle(myVehicle); do { menuchoice = menu(); switch (menuchoice) { case 1: AddVehicle(myVehicle); break; case 2: DeleteVehicle(myVehicle); break; case 3: carSort(myVehicle); break; case 4: printinfo(myVehicle); break; case 5: showVehicle(myVehicle); break; default: break; } } while (menuchoice !=0); return 0; }
Hi. I made a code but I have to change it. These are the requirements for the program code
• Safe entry
• No magic numbers
• No global variables
• File management must be in a separate c-file - I HAVEN'T DONE THIS AND I NEED HELP
• All standard header files, type declarations, function prototypes etc. must be included
a custom header file - I HAVEN'T DONE THIS EITHER
• Functions must not be “too long
and when the user chooses to quit "exit", the register must be written to a file - i haven't done this
So basically I need to change the code so that it fits the requirements
#include
#include
#include
#include
#define STR_LANGD 30
#define VEHICLE 10
#define NUMBEROF_PEOPLE 10
typedef struct
{
char name[STR_LANGD];
int age;
}
Person;
typedef struct
{
Person person;
char model[STR_LANGD];
char carbrand[STR_LANGD];
char registrationnumber[STR_LANGD];
}
Vehicle;
void initVehicle(Vehicle myVehicle[])
{
int i;
for (i = 0; i < VEHICLE; i++)
{
myVehicle[i].person.age = 0;
}
}
int menu() {
int choice = 0;
printf ("1. Add a vehicle. \n");
printf ("2. Remove a vehicle \n");
printf ("3. Sort by car brand. \n");
printf ("4. Print vehicle information.\n");
printf ("5. Print the entire vehicle register. \n");
printf ("0. Exit. \n");
scanf("%d", &choice);
return choice;
}
void AddVehicle(Vehicle myVehicle[])
{
Vehicle temp;
int i;
fflush(stdin);
printf("Enter name: \n");
scanf("%s", temp.person.name);
printf("Enter personens ålder: \n");
scanf("%d", &temp.person.age);
printf("Enter Vehiclestyp: \n");
scanf("%s", temp.model);
printf("Enter car brand: \n");
scanf("%s", temp.carbrand);
printf("Enter registration number: ");
scanf("%s", temp.registrationnumber);
for (i = 0; i < VEHICLE; i++)
{
if (myVehicle[i].person.age == 0)
{
myVehicle[i] = temp;
break;
}
}
}
void showVehicle(Vehicle myVehicle[])
{
int i;
printf("\nAll vehicle in
printf("-------------------\n");
for (i = 0; i < VEHICLE; i++)
{
if (myVehicle[i].person.age != 0)
{
printf("Place: %d\n", i);
printf("Name: ");
puts(myVehicle[i].person.name);
printf("Ålder på ägaren: %d\n", myVehicle[i].person.age);
printf("Car model: %s\n", myVehicle[i].model);
printf("Car brand: %s\n", myVehicle[i].carbrand);
printf("Registration number: %s\n\n", myVehicle[i].registrationnumber);
}
}
}
void DeleteVehicle(Vehicle myVehicle[VEHICLE])
{
int temp;
printf("På vilken plats (0-10) vill du ta bort Vehicleinfo?\nPlats: ");
scanf("%d", &temp);
if (myVehicle[temp].person.age != 0)
{
myVehicle[temp].person.age = 0;
}
}
void printinfo(Vehicle myVehicle[VEHICLE])
{
int temp;
printf("På vilken plats (0-10) vill du se Vehicleinfo?\nPlats: ");
scanf("%d", &temp);
if (myVehicle[temp].person.age != 0)
{
printf("\n------------Vehicleinfo-----------\n");
printf("Plats: %d", temp);
printf("name: %s\n", myVehicle[temp].person.name);
printf("Ålder på ägaren: %d\n", myVehicle[temp].person.age);
printf("Car model: %s\n", myVehicle[temp].model);
printf("Car brand: %s\n", myVehicle[temp].carbrand);
printf("Registration number: %s\n", myVehicle[temp].registrationnumber);
}
}
void carSort(Vehicle myVehicle[VEHICLE])
{
int i;
int j;
Vehicle temp;
for (i = 1; i < VEHICLE; i++)
{
for (j = 0; j < VEHICLE - i; j++)
{
if (myVehicle[j].carbrand[0] > myVehicle[j+1].carbrand[0])
{
temp = myVehicle[j];
myVehicle[j] = myVehicle[j+1];
myVehicle[j+1] = temp;
}
}
}
}
int main() //main: här startar alla C-program
{
Vehicle myVehicle[VEHICLE];
int menuchoice = 0;
initVehicle(myVehicle);
do
{
menuchoice = menu();
switch (menuchoice)
{
case 1: AddVehicle(myVehicle); break;
case 2: DeleteVehicle(myVehicle); break;
case 3: carSort(myVehicle); break;
case 4: printinfo(myVehicle); break;
case 5: showVehicle(myVehicle); break;
default: break;
}
}
while (menuchoice !=0);
return 0;
}

Step by step
Solved in 3 steps with 6 images

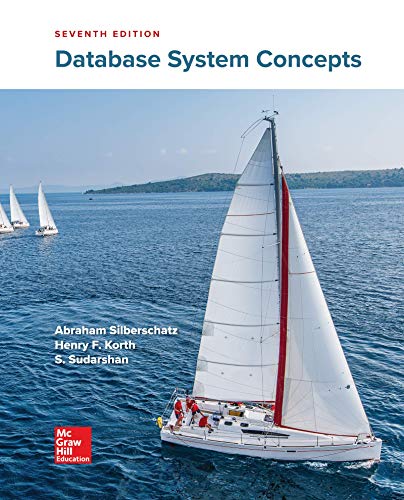
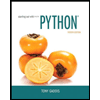
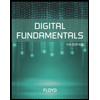
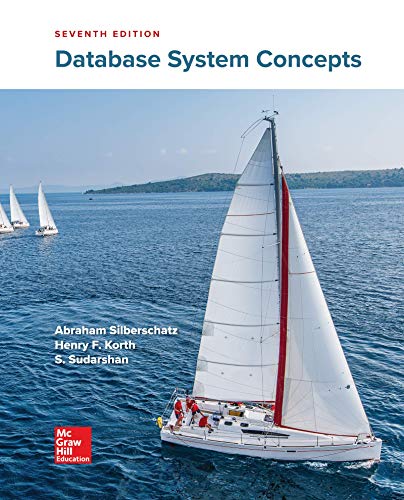
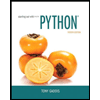
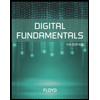
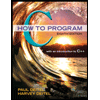
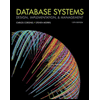
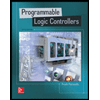