• All of Luis' friends are Python programmers, so he wants to share the decoding function with them. Write another method to decode Luis' message. You'll need to reverse the encryption scheme that Luis uses. After each vowel in the message (a, e, i, o, or u), he adds a letter (r, s, or t) followed by the same vowel. Function heading: def decode_message(message): Parameter Specification: An encoded sentence Return Value Specification: The decoded version of the input sentence Sample Function Call: decode_message("Herelloto Wororld") Sample Return Value: "Hello World" Sample Function Call: decode_message("Disinneter tosonisight asat Tararata Thasairi.") Sample Return Value: "Dinner tonight at Tara Thai." Sample Function Call: decode_message("Meteeset asat seseveten otoclosock.") Sample Return Value: "Meet at seven oclock."
• All of Luis' friends are Python programmers, so he wants to share the decoding function with them. Write another method to decode Luis' message. You'll need to reverse the encryption scheme that Luis uses. After each vowel in the message (a, e, i, o, or u), he adds a letter (r, s, or t) followed by the same vowel. Function heading: def decode_message(message): Parameter Specification: An encoded sentence Return Value Specification: The decoded version of the input sentence Sample Function Call: decode_message("Herelloto Wororld") Sample Return Value: "Hello World" Sample Function Call: decode_message("Disinneter tosonisight asat Tararata Thasairi.") Sample Return Value: "Dinner tonight at Tara Thai." Sample Function Call: decode_message("Meteeset asat seseveten otoclosock.") Sample Return Value: "Meet at seven oclock."
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:• All of Luis' friends are Python programmers, so he wants to share the decoding function with them. Write another method to decode
Luis' message.
You'll need to reverse the encryption scheme that Luis uses. After each vowel in the message (a, e, I, o, or u), he adds a letter (r, s, or t)
followed by the same vowel.
Function heading: def decode_message(message):
Parameter Specification: An encoded sentence
Return Value Specification: The decoded version of the input sentence
Sample Function Call: decode_message("Herelloto Wororld")
Sample Return Value: "Hello World"
Sample Function Call:
decode_message("Disinneter tosonisight asat Tararata Thasairi.")
Sample Return Value: "Dinner tonight at Tara Thai."
Sample Function Call:
decode_message("Meteeset asat seseveten otoclosock.")
Sample Return Value: "Meet at seven oclock."

Transcribed Image Text:Program: final.py
Specification
First, download the template file, final.py. This file contains the function names and documentation.
You are required to add a header comment block with your name, the file name, the current semester, and year. Do not change the function
headers, just add your implementation for each function where indicated.
Add descriptive comments to your code. Remember to indent all the code for the function.
Here are the functions that you will write:
• Calculating tips is a common operation and the first function you write for the final project will do exactly that. The function
calculate_tip accepts two parameters, the bill amount and the percent to tip, and returns the amount of the tip to write into the bill. The
function should return the string "Invalid charge amount" if the charge is not more that 0 and "Invalid tip percent" if the tip is less than
0.
Function heading: def calculate tip(charge, tip_percent):
Parameter Specification: charge is the total ch and tip_percent is the % of total
Return Value Specification: the amount to tip based on the charge and percent given
Sample Function Call: calculate tip(27.5, 20)
Sample Return Value: 5.5
Sample Function Call: calculate_tip(-1.0, 15)
Sample Return Value: Invalid charge amount
Sample Function Call: calculate_tip(27.5, -1)
Sample Return Value: Invalid tip percent
The function classify_student has an integer as a parameter and returns the string that represents the year according to this
table:
Year
Credits
Freshmen one to 23 credit hours
Sophomores24 to 53 credit hours
Juniors 54 to 84 credit hours
Seniors 85 credit hours and more
Function heading: def classify_student(credits):
Parameter Specification: A positive integer
Return Value Specification: One of the following: Freshman, Sophomore, Junior, Senior
Sample Function Call: classify_student(20)
Sample Return Value: Freshman
Sample Function Call: classify_student(120)
Sample Return Value: Senior
Sample Function Call: classify_student(0)
Sample Return Value: Insufficient credits for classification
• Luis likes to hold secret gatherings of his friends. He restricts who knows about them by sending out an encoded version of the
invitation. The function encode_message has a string (the message) as a parameter and returns the encoded message as a string.
Here's the encryption scheme that Luis uses. After each vowel in the message (a, e, I, o, or u), he randomly selects a letter from (r, s, or t)
followed by the same vowel.
Function heading: def encrypt_message(message):
Parameter Specification: A sentence in plain English
Return Value Specification: The encoded version of the input sentence
Sample Function Call: encrypt_message("Hello World")
Sample Return Value: "Herelloto Wororld " or "Heselloro Wotorld" or some other variation
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
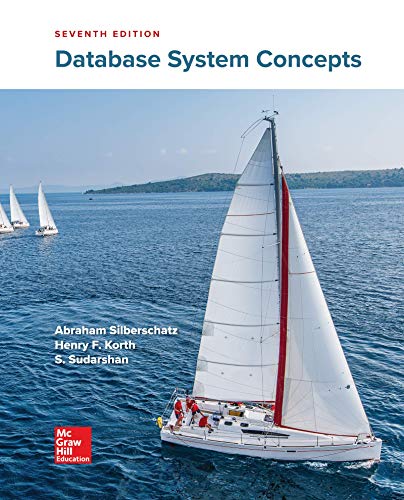
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
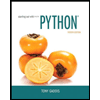
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
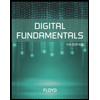
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
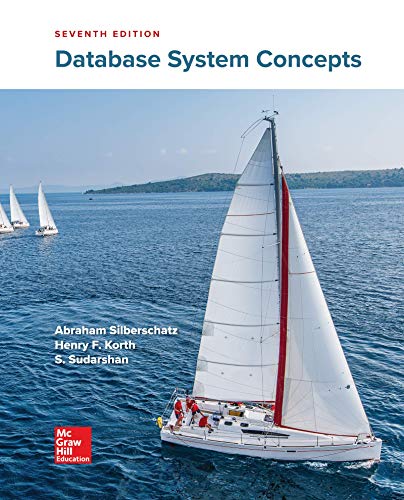
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
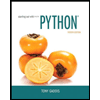
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
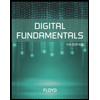
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
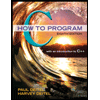
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
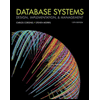
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
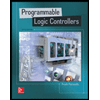
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education