Write a C++ program that interacts with a text file that contains a list of student IDs and their corresponding grades for their test. The program will import the file contents into the program, separate the IDs and their grades, and then sort the students based on their grade in descending order. The program will then display the top 10 scores, as well as the names of the students who earned those scores. You will make a class called Student. This class will have three attributes, one that stores their name, one that stores their grade, and one that stores a unique ID number. Additionally, there will be setters and getters for each of the attributes, where the setter for “grade” should ensure that the grade is valid (i.e., between 0 and 100). In addition to the setters and getters, you will overload the relational operators (i.e., ==, < > <= >= !=) in order to make student objects sortable. The following rules apply for comparing students: Two or three student objects are equal if their IDs match. student_1 is “less than” student_2 based on the following priority: student_1’s grade is less than student_2’s If the grades are the same, then student_1’s name is less than student_2’s If both name and grade are the same, then student_1’s ID is less than student_2’s. Apply and interpret these rules for the rest of the relational operators. Once you have finished the class definition and test whether objects are correctly comparable, then you will load the student data from the file and use this data to generate a vector of student objects that contain the data. Afterwards, you will sort the vector. To do this, you will need to implement a sorting algorithm (Note: You are NOT permitted to use one of C++’s built in sorting algorithms). You are allowed to choose either Quick Sort or Merge Sort for your algorithm.
Please write C++ code for the following. Please show all source code. Thank you.
Write a C++ program that interacts with a text file that contains a list of student IDs and their corresponding grades for their test. The program will import the file contents into the program, separate the IDs and their grades, and then sort the students based on their grade in descending order. The program will then display the top 10 scores, as well as the names of the students who earned those scores.
You will make a class called Student. This class will have three attributes, one that stores their name, one that stores their grade, and one that stores a unique ID number. Additionally, there will be setters and getters for each of the attributes, where the setter for “grade” should ensure that the grade is valid (i.e., between 0 and 100).
In addition to the setters and getters, you will overload the relational operators (i.e., ==, < > <= >= !=) in order to make student objects sortable.
The following rules apply for comparing students:
- Two or three student objects are equal if their IDs match.
- student_1 is “less than” student_2 based on the following priority:
- student_1’s grade is less than student_2’s
- If the grades are the same, then student_1’s name is less than student_2’s
- If both name and grade are the same, then student_1’s ID is less than student_2’s.
- Apply and interpret these rules for the rest of the relational operators.
- Once you have finished the class definition and test whether objects are correctly comparable, then you will load the student data from the file and use this data to generate a
vector of student objects that contain the data. Afterwards, you will sort the vector. To do this, you will need to implement a sortingalgorithm (Note: You are NOT permitted to use one of C++’s built in sorting algorithms). You are allowed to choose either Quick Sort or Merge Sort for your algorithm. You may also use an existing solution, but it MUST be cited by placing a comment above the source code.
The citation requirements are as followed:
- /*
- Website Name:
- Date Accessed:
- URL:
- */

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

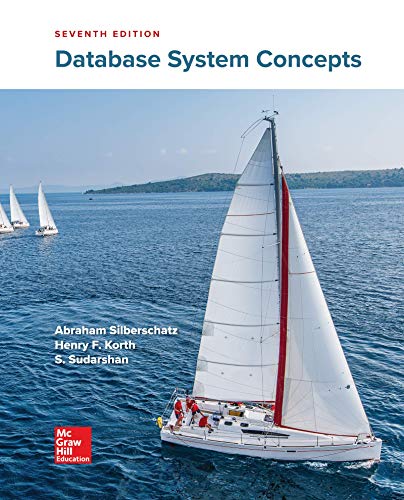
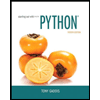
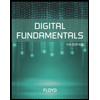
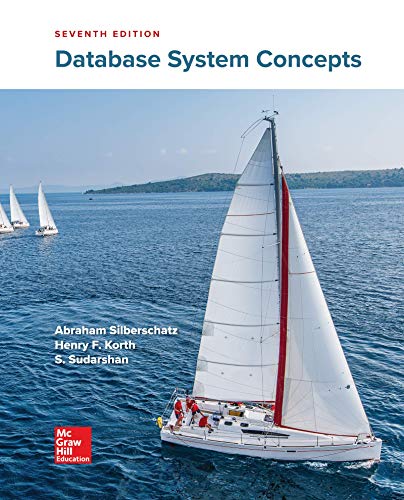
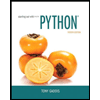
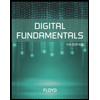
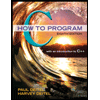
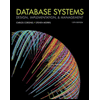
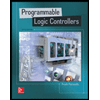