Using the data file capabilities of C++, create a Personnel file, personnel.dat on your drive and the C++ program that will write employee data to that file. Your file will contain six employees: Last Name: Jones Kirk McCoy Doe Boop Rabbitt First Name: Davy James Leonard Jayne Betty Jessica Worker ID: D1234 K2231 M1856 D0104 B2525 R0331 Hours: 20.5 40 45 25.5 40 40 Pay Rate: $10.25 $15.50 $20.97 $10.00 $16.00 $15.00 Tax Rate: 0.05 0.25 0.3 0.05 0.10 0.3 Each employee's record should also include three MORE fields for: Gross Pay = Hours X Pay Rate Taxes = Gross Pay * Tax Rate Net Pay = Gross Pay - (Gross Pay X Tax Rate) This assignment will have two parts: 1) Entering, writing, records into the file. The logic should allow the user to enter as many records as they wish, compute Gross Pay and Net Pay. Write the data to the data file, Personnl.Dat. Put a single blank between each data field to act as a default delimiter. 2) Reading records from the file and printing them on the screen. Retrieve each record. Read a record and check for the EOF. Since you really don't know how many records are in the data file, use a while loop. The loop will read the record at the end of the loop. Be sure to format appropriate values as currency. See if you can format the hours as decimal with 1 decimal position (i.e. 40 hours as 40.0 hours). Use a While loop around the main logic to let the user keep adding or reading instead of one pass through the entire program logic. One combined program…..the application must allow the user to switch between writing records and reading them (write two separate programs and your grade “suffers”). Display your employee output as an “itemized display”. Present a “menu” as an interface to the user. Enter an Option 1 - Write new Records to the Data file 2 - Display Records From the Data file to the Screen 3 - Find ALL Records with a Pay Rate Less than $ "N" Dollars Per Hour 4 - QUIT Enter Your option: 2 Output Example (reading the file’s records): Record #1 Employee ID: AD2201 Last Name: Pokemon First Name: Pikachu Hours Worked: 40.00 Pay Per Hour: $12.34 Gross Pay: $493.60 Taxes: $14.81 Net Pay: $478.79 Employee Tax Rate: 3.00% Record #2 Employee ID: AF0091 Last Name: Pokemon First Name: Squirtel Hours Worked: 35.67 Pay Per Hour: $23.54 Gross Pay: $839.67 Taxes: $25.19 Net Pay: $814.48 Employee Tax Rate: 3.00% Record #3 Employee ID: AS2333 Last Name: Tralfaz First Name: Henri Hours Worked: 34.50 Pay Per Hour: $23.45 Gross Pay: $809.03 Taxes: $161.81 Net Pay: $647.22 Employee Tax Rate: 20.00% Your data file opened under an ASCII editor such as Notepad should appear in a configuration like: AD2201 Pokemon Pikachu 40.00 12.34 493.60 14.81 14.81 463.98 0.03 0.03 AF0091 Pokemon Squirtel 35.67 23.54 839.67 25.19 33.59 780.89 0.03 0.04 AS2333 Tralfaz Henri 34.50 23.45 809.03 161.81 242.71 404.51 0.20 0.30 DA1120 Pokemon Pikachu 40.00 12.35 494.00 14.82 12.35 466.83 0.03 0.03 DC2231 Pokemon Balbasaur 33.50 12.34 413.39 8.27 4.13 400.99 0.02 0.01 Enter an Option 1 - Write new Records to the Data file 2 - Display Records From the Data file to the Screen 3 - Find ALL Records with a Pay Rate Less than $ "N" Dollars Per Hour 4 - QUIT Enter Your option: 3 Finding ALL Employees with Pay Rates Less than $ "N" Per Hour Enter the Pay Rate Amount to Find All Employees have a Pay Rate "Less" than: 15.75 Record #1 Employee ID: AD220 Last Name: Pokemon First Name: Pikachu Hours Worked: 40.00 Pay Per Hour: $12.34 Gross Pay: $493.60 Federal Taxes: $14.81 State Taxes $14.81 Net Pay: $463.98 Employee Federal Tax Rate: 3.00% Employee State Tax Rate: 3.00%
Using the data file capabilities of C++, create a Personnel file, personnel.dat on your drive and the C++ program that will write employee data to that file. Your file will contain six employees:
Last Name: Jones Kirk McCoy Doe Boop Rabbitt
First Name: Davy James Leonard Jayne Betty Jessica
Worker ID: D1234 K2231 M1856 D0104 B2525 R0331
Hours: 20.5 40 45 25.5 40 40
Pay Rate: $10.25 $15.50 $20.97 $10.00 $16.00 $15.00
Tax Rate: 0.05 0.25 0.3 0.05 0.10 0.3
Each employee's record should also include three MORE fields for:
Gross Pay = Hours X Pay Rate
Taxes = Gross Pay * Tax Rate
Net Pay = Gross Pay - (Gross Pay X Tax Rate)
This assignment will have two parts:
1) Entering, writing, records into the file.
The logic should allow the user to enter as many records as they wish, compute Gross Pay and Net Pay. Write the data to the data file, Personnl.Dat. Put a single blank between each data field to act as a default delimiter.
2) Reading records from the file and printing them on the screen.
Retrieve each record. Read a record and check for the EOF. Since you really don't know how many records are in the data file, use a while loop. The loop will read the record at the end of the loop.
Be sure to format appropriate values as currency. See if you can format the hours as decimal with 1 decimal position (i.e. 40 hours as 40.0 hours). Use a While loop around the main logic to let the user keep adding or reading instead of one pass through the entire program logic.
One combined program…..the application must allow the user to switch between writing records and reading them (write two separate programs and your grade “suffers”).
Display your employee output as an “itemized display”. Present a “menu” as an interface to the user.
Enter an Option
1 - Write new Records to the Data file
2 - Display Records From the Data file to the Screen
3 - Find ALL Records with a Pay Rate Less than $ "N" Dollars Per Hour
4 - QUIT
Enter Your option: 2
Output Example (reading the file’s records):
Record #1
Employee ID: AD2201
Last Name: Pokemon First Name: Pikachu
Hours Worked: 40.00 Pay Per Hour: $12.34 Gross Pay: $493.60
Taxes: $14.81 Net Pay: $478.79
Employee Tax Rate: 3.00%
Record #2
Employee ID: AF0091
Last Name: Pokemon First Name: Squirtel
Hours Worked: 35.67 Pay Per Hour: $23.54 Gross Pay: $839.67
Taxes: $25.19 Net Pay: $814.48
Employee Tax Rate: 3.00%
Record #3
Employee ID: AS2333
Last Name: Tralfaz First Name: Henri
Hours Worked: 34.50 Pay Per Hour: $23.45 Gross Pay: $809.03
Taxes: $161.81 Net Pay: $647.22
Employee Tax Rate: 20.00%
Your data file opened under an ASCII editor such as Notepad should appear in a configuration like:
AD2201 Pokemon Pikachu 40.00 12.34 493.60 14.81 14.81 463.98 0.03 0.03
AF0091 Pokemon Squirtel 35.67 23.54 839.67 25.19 33.59 780.89 0.03 0.04
AS2333 Tralfaz Henri 34.50 23.45 809.03 161.81 242.71 404.51 0.20 0.30
DA1120 Pokemon Pikachu 40.00 12.35 494.00 14.82 12.35 466.83 0.03 0.03
DC2231 Pokemon Balbasaur 33.50 12.34 413.39 8.27 4.13 400.99 0.02 0.01
Enter an Option
1 - Write new Records to the Data file
2 - Display Records From the Data file to the Screen
3 - Find ALL Records with a Pay Rate Less than $ "N" Dollars Per Hour
4 - QUIT
Enter Your option: 3
Finding ALL Employees with Pay Rates Less than $ "N" Per Hour
Enter the Pay Rate Amount to Find All Employees have a Pay Rate "Less" than: 15.75
Record #1
Employee ID: AD220
Last Name: Pokemon First Name: Pikachu
Hours Worked: 40.00 Pay Per Hour: $12.34 Gross Pay: $493.60
Federal Taxes: $14.81 State Taxes $14.81 Net Pay: $463.98
Employee Federal Tax Rate: 3.00% Employee State Tax Rate: 3.00%

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

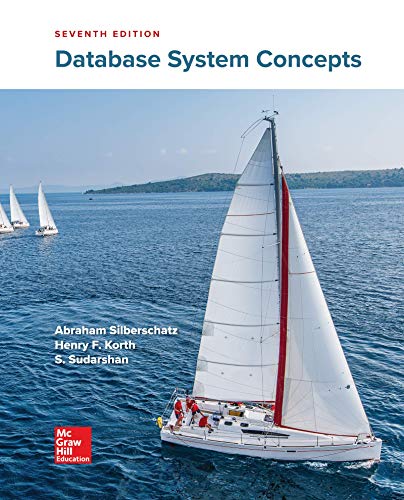
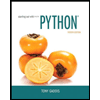
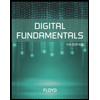
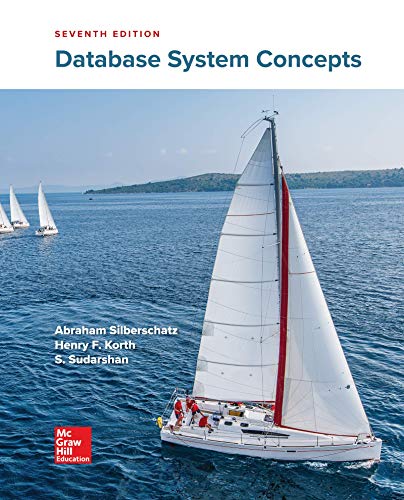
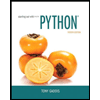
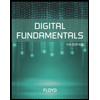
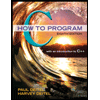
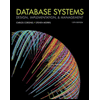
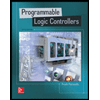