Create a class
First programming homework
Create a class of records for a gradebook called Rec.
The data should be private, and should include a firstname, lastname, array of three grades, and a field for average grade.
Build two constructors: a default constructor and a constructor that takes the first and last name.
Build a function to read the data, either from a file or from cin. The read function reads the two names and three grades, but does not read the average grade.
Build a write function that writes the data either to a file or to cout. The write function prints all of the data on one line with spaces between fields.
Build a function to calculate the average grade field.
Build an overloaded operator == to compare two records. This should be implemented as a friend function.
Declare the constructors and functions in the body of the class, but implement them outside the class.
I will provide you with a driver called hw1.cpp in ~cthorpe/public/142 and with a test file HW1.txt in the same directory
HW1.txt contains:
Pete Jones 1 2 4
I attached hw1.cpp


As per bartleby guidelines, for a multipart question, i am answering first 3 subparts of the question, please post a separate question for rest of the query.
for making the data private, private access modifier is used.
default constructor and parameterized constructor are created in public access modifier. Default constructor does not take arguments . Paramaeterized constructor, first name and last name values are passed as arguments.
Step by step
Solved in 2 steps

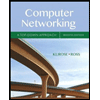
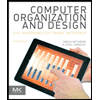
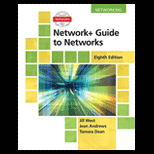
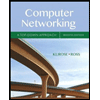
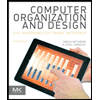
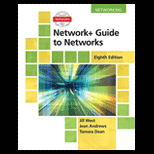
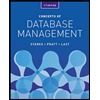
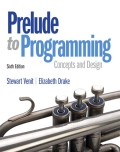
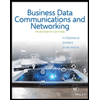