Explain the functionality of each line of code in the provided C# scripts- using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class Healthbar : MonoBehaviour { [SerializeField] private Health playerHealth; [SerializeField] private Image totalHealthbar; [SerializeField] private Image currentHealthbar; private void Start() { totalHealthbar.fillAmount = playerHealth.currentHealth / 10; } private void Update() { currentHealthbar.fillAmount = playerHealth.currentHealth / 10; } } using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.SceneManagement; public class Health : MonoBehaviour { [SerializeField] private float startingHealth; public float currentHealth { get; private set; } private Animator anim; private void Awake() { currentHealth = startingHealth; anim = GetComponent(); } public void TakeDamage(float _damage) { currentHealth = Mathf.Clamp(currentHealth - _damage, 0, startingHealth); if (currentHealth <= 0) { anim.SetTrigger("death"); GetComponent().bodyType = RigidbodyType2D.Static; } } private void OnCollisionEnter2D(Collision2D collision) { if (collision.gameObject.CompareTag("Spike Trap")) { TakeDamage(1); } } private void RestartLevel() { SceneManager.LoadScene(SceneManager.GetActiveScene().name); } } using System.Collections; using System.Collections.Generic; using UnityEngine; public class GroundCheck : MonoBehaviour { GameObject Player; private void Start() { Player = gameObject.transform.parent.gameObject; } private void OnCollisionEnter2D(Collision2D collision) { if (collision.collider.tag == "Ground" || collision.collider.tag == "Platform") { Player.GetComponent().isGrounded = true; } } private void OnCollisionExit2D(Collision2D collision) { if(collision.collider.tag == "Ground"|| collision.collider.tag == "Platform") { Player.GetComponent().isGrounded = false; } } } using System.Collections; using System.Collections.Generic; using UnityEngine; public class PlatformManager : MonoBehaviour { GameObject[] platforms; GameObject currentPlatform; int index; public GameObject coin; private void Start() { NewPlatform(); } // Start is called before the first frame update public void NewPlatform() { platforms = GameObject.FindGameObjectsWithTag("Platform"); index = Random.Range(0, platforms.Length); currentPlatform = platforms[index]; coin.transform.position = new Vector2(currentPlatform.transform.position.x, currentPlatform.transform.position.y + 2f);
Explain the functionality of each line of code in the provided C# scripts-
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class Healthbar : MonoBehaviour
{
[SerializeField] private Health playerHealth;
[SerializeField] private Image totalHealthbar;
[SerializeField] private Image currentHealthbar;
private void Start()
{
totalHealthbar.fillAmount = playerHealth.currentHealth / 10;
}
private void Update()
{
currentHealthbar.fillAmount = playerHealth.currentHealth / 10;
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Health : MonoBehaviour
{
[SerializeField] private float startingHealth;
public float currentHealth { get; private set; }
private Animator anim;
private void Awake()
{
currentHealth = startingHealth;
anim = GetComponent<Animator>();
}
public void TakeDamage(float _damage)
{
currentHealth = Mathf.Clamp(currentHealth - _damage, 0, startingHealth);
if (currentHealth <= 0)
{
anim.SetTrigger("death");
GetComponent<Rigidbody2D>().bodyType = RigidbodyType2D.Static;
}
}
private void OnCollisionEnter2D(Collision2D collision)
{
if (collision.gameObject.CompareTag("Spike Trap"))
{
TakeDamage(1);
}
}
private void RestartLevel()
{
SceneManager.LoadScene(SceneManager.GetActiveScene().name);
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GroundCheck : MonoBehaviour
{
GameObject Player;
private void Start()
{
Player = gameObject.transform.parent.gameObject;
}
private void OnCollisionEnter2D(Collision2D collision)
{
if (collision.collider.tag == "Ground" ||
collision.collider.tag == "Platform")
{
Player.GetComponent<Movement2D>().isGrounded = true;
}
}
private void OnCollisionExit2D(Collision2D collision)
{
if(collision.collider.tag == "Ground"||
collision.collider.tag == "Platform")
{
Player.GetComponent<Movement2D>().isGrounded = false;
}
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlatformManager : MonoBehaviour
{
GameObject[] platforms;
GameObject currentPlatform;
int index;
public GameObject coin;
private void Start()
{
NewPlatform();
}
// Start is called before the first frame update
public void NewPlatform()
{
platforms = GameObject.FindGameObjectsWithTag("Platform");
index = Random.Range(0, platforms.Length);
currentPlatform = platforms[index];
coin.transform.position = new Vector2(currentPlatform.transform.position.x, currentPlatform.transform.position.y + 2f);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps

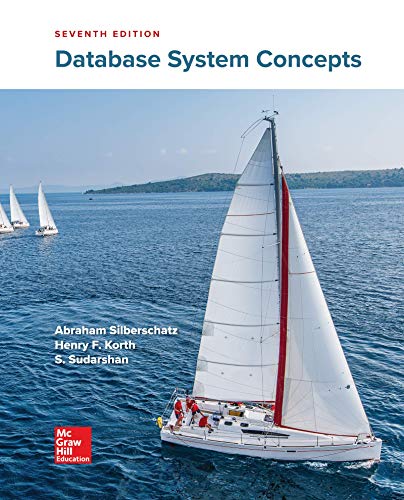
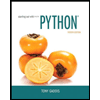
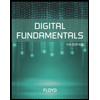
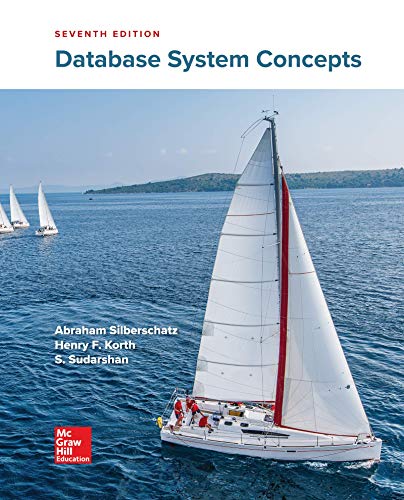
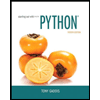
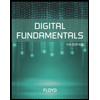
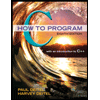
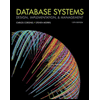
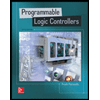