Create student object class (Student.java) Student objects should have the following attributes: student name major class name course id grade credits Create Course object class (Course.java) Course object should be the following attributes: course id instructor id room id Create testStudent class Output Create a report that has
Create student object class (Student.java)
- Student objects should have the following attributes:
- student name
- major
- class name
- course id
- grade
- credits
Create Course object class (Course.java)
- Course object should be the following attributes:
- course id
- instructor id
- room id
Create testStudent class
- Output
- Create a report that has appropriate headings and 1 line of detail for each student record read in. Detail lines should include the following information:
- student name,
- class id,
- Instructor id,
- room id,
- grade,
- credits,
- comment
- Read student information, process the information and print an output line for each student record in the ClassesData.dat file. You will also need the CoursesData.dat file for courses information.
- A second version of the data is available. You can use either version of these files. Just be sure to zip the data files your program solution uses, with your submission: NewCoursesData.txt , NewClassesData.txt
The comments section of the detail line should display the String "Great Job!" if the student gets an 'A' grade.
Zip these five files into 1 zipped file named <yourlastname>Final(example watneFinal):
- Student.java
- Course.java
- testStudent.java
- ClassesData.dat
- CoursesData.dat.
Submit the zipped file as an attachment.
ClassesData.dat:
1001Intro. to CompSci 4ALBERT, PETER A. Comp Info System A
1001Intro. to CompSci 4ALLENSON, SHEILA M. Comp Info System B
1001Intro. to CompSci 4ANDERSON, ALENE T. Comp Info System A
1001Intro. to CompSci 4HENDRIX, JAMES D. Lib Arts - MIS C
1001Intro. to CompSci 4CANNON, FREDDY B.B. Comp Info System B
1002Visual Basic 3ALBERT, PETER A. Comp Info System C
1002Visual Basic 3ALLENSON, SHEILA M. Comp Info System D
1002Visual Basic 3ANDERSON, ALENE T. Comp Info System A
1002Visual Basic 3HENDRIX, JAMES D. Lib Arts - MIS B
1002Visual Basic 3CANNON, FREDDY B.B. Comp Info System B
1003Cisco Networking I 4ALBERT, PETER A. Comp Info System C
1003Cisco Networking I 4ALLENSON, SHEILA M. Comp Info System A
1003Cisco Networking I 4ANDERSON, ALENE T. Comp Info System A
1003Cisco Networking I 4HENDRIX, JAMES D. Lib Arts - MIS D
1004Cisco Networking III3ALBERT, PETER A. Comp Info System B
1004Cisco Networking III3ALLENSON, SHEILA M. Comp Info System C
1004Cisco Networking III3ANDERSON, ALENE T. Comp Info System A
1004Cisco Networking III3CANNON, FREDDY B.B. Comp Info System B
1004Cisco Networking III3HELLER, HELEN H. Lib Arts - MIS A
1004Cisco Networking III3HENDRIX, JAMES D. Lib Arts - MIS F
CoursesData.dat:
1001WATNE BABCOCK134
1002KROSHUS HORTON30
1003SCHILLINGER LIBRARY118
1004SCHWANTZ BABCOCK134
I have two pictures. The first picture, that error appears when I try to run the Java application for the Student.java and Course.java.
The second picture, errors I receive when trying to compile the testStudent.java. I presume I'll probably get the same error as the Student and Course so I would like that fixed as well.
![Document Selector
testStudent.java x
testStudent.java
package package1;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class testStudent {
public static void main(String[] args) {
Student students[] = new Student[25];
Course courses[] = new Course[5];
String s_name, major, class_name, instructor_id, room_id;
int cou_id, credits, index, stud_count = , cou_count
char grade;
try {
File fclasses = new File("E://ClassesData.dat");
Scanner myReader
= 0;
= new Scanner (fclasses);
while (myReader.hasNextline()) {
String data = myReader.nextLine();
String[] toks
cou_id = Integer.parseInt (toks[0].substring(0, 4));
class_name =
for (index - 1;; index++) {
if (Character.isDigit(toks[index].charAt(0)))
break;
else {
class_name +=
}
}
data.split("\\s+");
%3D
toks[0].substring(4);
+ toks[index];
credits = Integer.parseInt (toks[index].substring (0, 1));
toks[index].substring (1);
for (index = index + 1;; index++) {
s_name =
Ca Expl. 9 Docu. Clip .
Tool Output
symbol:
location: class testStudent
class Student
C: \Users\Alyssa\Documents\CSCI 160\packagel\testStudent.java:9: error: cannot find symbol
Student students[]
= new Student[25];](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F859cbc42-a46d-4010-ba0f-00da25d9d32e%2F02f2bc0d-eaf5-4038-80bd-278e77b0d63e%2Ff4fcb5l_processed.png&w=3840&q=75)
![Course.java X
C:\WINDOWS\system32\cmd.exe
package package1;
public_stat ic void main(Stringl] args)
or a JavaFX applicat ion class must extend javafx.applicat ion. Applicat ion
public class Course {
Press any key to cont inue . . .
int cou id;
String inst_id, room_id;
public Course() {
cou_id = 0;
inst_id = room_id = "";
}
public void assign(int course_id, String instructor_id, String room_id) {
this.cou_id = course_id;
this.inst_id = instructor_id;
this.room id = room id;
}
public boolean match_course_id(int stud_course_id) {
if (this.cou_id -- stud_course_id) {
System.out.println("Instructor Id: " + this.inst_id);
System.out.println("Room Id: " + this.room_id);
return true;
}
return false;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F859cbc42-a46d-4010-ba0f-00da25d9d32e%2F02f2bc0d-eaf5-4038-80bd-278e77b0d63e%2Fbqm7pru_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 4 images

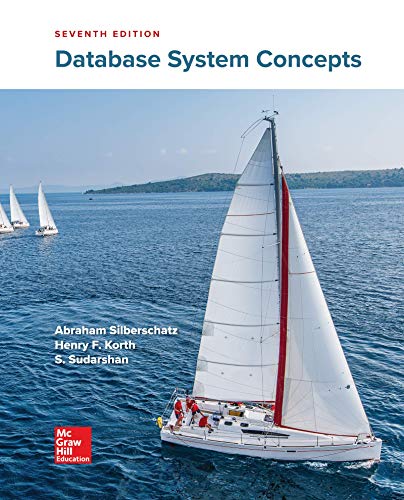
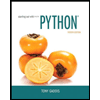
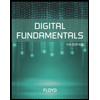
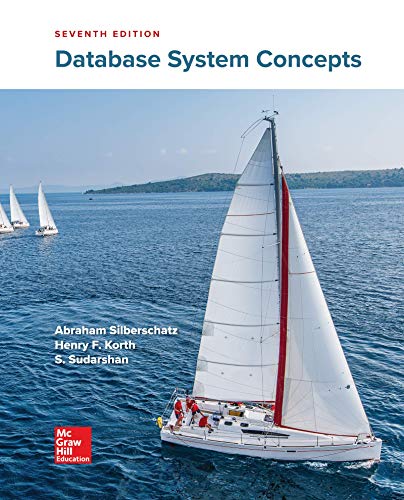
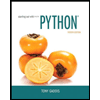
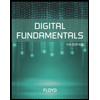
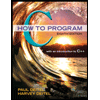
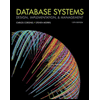
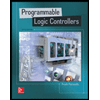