This function returns the number of items within the SetList. class Setlist: class Node: def __init__(self,data,set_list,next_node, prev_node): def get_data(self): def get_next (self): def get_previous (self): def get_set(self): def __init__(self): def get_front (self): def get back(self): def make_set(self, data): def find_data(self, data): def representative node(self):
This function returns the number of items within the SetList. class Setlist: class Node: def __init__(self,data,set_list,next_node, prev_node): def get_data(self): def get_next (self): def get_previous (self): def get_set(self): def __init__(self): def get_front (self): def get back(self): def make_set(self, data): def find_data(self, data): def representative node(self):
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please help me to finish this code in python

Transcribed Image Text:"write a"
Please help me to finish this code in python
The class declarations have been created in the a1_partb.py starter file. You are responsible to write all the listed
functions.
You are allowed to add data members to both Node and SetList.
Node
The Node class is declared within SetList. It stores:
• a piece of data
• a reference to the next Node in the SetList (None if Node is last node)
• a reference to the previous Node in the SetList (None if Node is first node)
• a reference to the SetList.
When a Node is initialized, it is passed a data value and a reference to the Setlist the Node belongs to. Optionally it is
also passed a reference to the next node and a reference to the previous node (in that order). If the data values are
not passed in, they are defaulted to None.
The Node function has the following member functions:
def get_data(self)
function returns data stored in node
def get_next(self)
function returns reference to next node in SetList
def get_previous(self)
function returns reference to previous node in SetList
def get_set(self)
function returns reference to the SetList
front
back
444
data
next
prev
set
cat
data
next
prev
set
fish
·
4
data
next
prev
set
dog

Transcribed Image Text:A SetList is a doubly linked list that can be used to represent a single set of a disjoint set. With a SetList, each node
contains data and is linked to other nodes in the list. The difference with a regular doubly linked list is that each node
also contains a link to the SetList object. The first node within the SetList contains the set's representative.
The following is a diagram of the set: {"cat", "fish", "dog"}. Note that values within a set do not have any particular
ordering and the exact ordering does not matter in your implementation. This means that any of the 3 can be the
representative..its simply a matter of which one is at the front of the list
When a SetList is initialized, the SetList is empty.
The SetList has the following member functions
def get_front(self)
This function returns a reference to the first node in the list
def get_back(self)
This function returns a reference to the last node in the list
def make_set(self, data)
This function adds the first value to the SetList object if the list is empty and returns a reference to newly created
Node. If the list is not empty, function does nothing and returns None.
def union_set(self, other_set)
This function is passed a SetList and performs a union with the current object. This means that the elements of
other_set are transfered to current object and other set becomes empty in the process. Function returns number of
elements transferred. Note, this process does not create or destroy any of the nodes.
def find_data(self, data)
This function is passed a data value, the function returns the a reference to the Node containing data. If data does not
exist, function returns None
def representative_node(self)
This function returns a reference to the node that holds the representative of the SetList. If SetList is empty, function
returns None
def representative(self)
This function returns the data of the representative node of the SetList. If SetList is empty, function returns None
def_len_(self)
This function returns the number of items within the SetList.
class Setlist:
class Node:
def __init__(self,data,set_list, next_node, prev_node):
def get_data(self):
def get_next(self):
def get_previous(self):
def get_set(self):
def __init__(self):
def get_front (self):
def get back(self):
def make_set(self, data):
def find_data(self, data):
def representative_node (self):
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
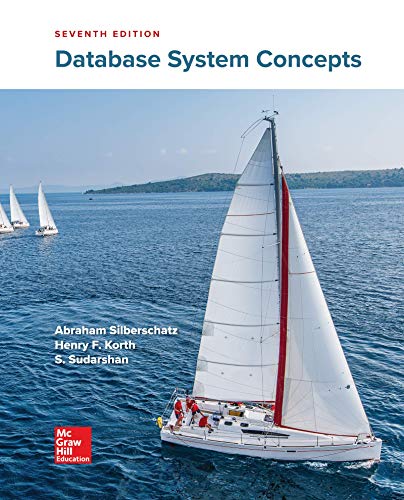
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
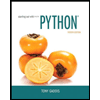
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
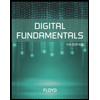
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
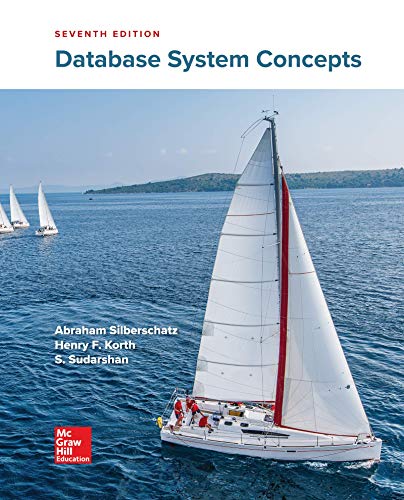
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
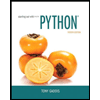
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
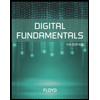
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
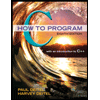
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
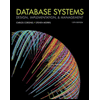
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
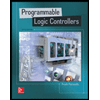
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education