Write Python function to generate prime numbers. def gen_prime(n): Ist = gen_prime(10) print(Ist) 1,2,3,5,7,11,13,17,19,23 Use shift+enter to run each cell. submit .py file
Write Python function to generate prime numbers. def gen_prime(n): Ist = gen_prime(10) print(Ist) 1,2,3,5,7,11,13,17,19,23 Use shift+enter to run each cell. submit .py file
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help with these python codes

Transcribed Image Text:**Generating Prime Numbers Using Python**
To create a Python function that generates prime numbers, follow these steps:
```python
def gen_prime(n):
# Define the function logic here
pass
lst = gen_prime(10)
print(lst)
```
This code snippet will output:
```
1, 2, 3, 5, 7, 11, 13, 17, 19, 23
```
To execute each cell of code, use `shift+enter`.
Once completed, submit your `.py` file for evaluation.

Transcribed Image Text:### Prime Number Detection with Python
**Objective:**
Write a Python function to detect if a number is prime or not.
**Function Definition:**
```python
def is_prime(n):
# Your code here
```
**Task:**
Use the function to detect whether the following numbers are prime:
- 2
- 5
- 99
- 87
- 101
- 115
**Instructions:**
1. Implement the brute force approach discussed in class.
2. Submit your code.
3. Develop new ideas to optimize and improve your algorithm.
**Note:** Ensure the output is user-friendly.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 8 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
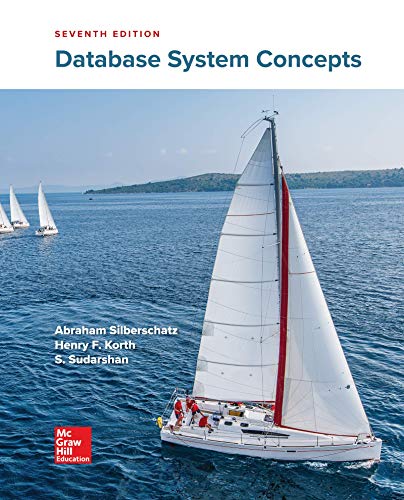
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
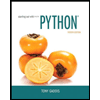
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
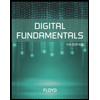
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
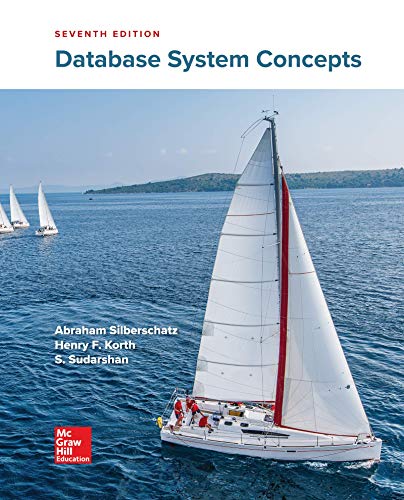
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
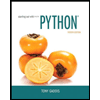
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
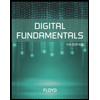
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
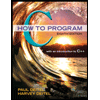
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
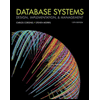
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
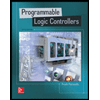
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education