Checkpoint A Implement these functions from the template following the description (specification) in their docstring: generate_lottery_numbers() count_matches () play_lottery_once() sim_many_plays () By default, this project does not generate any interesting printed output. So, you should use one of these strategies in your work: • Use Python Tutor to do incremental development, focusing on one function at a time in isolation • Invent some of your own intermediate output, to get feedback while in develop mode Submit your work to get feedback from unit tests ● Hint: you can use random.randint (a, b) to select random numbers between a and b. Hint: The behavior of sim_many_plays will simulate a single player and their overall winnings at the end of each play. For example, if they play 4 times and lose each time, the record of their overall winnings in each round would be [-1, -2, -3, -4].
Checkpoint A Implement these functions from the template following the description (specification) in their docstring: generate_lottery_numbers() count_matches () play_lottery_once() sim_many_plays () By default, this project does not generate any interesting printed output. So, you should use one of these strategies in your work: • Use Python Tutor to do incremental development, focusing on one function at a time in isolation • Invent some of your own intermediate output, to get feedback while in develop mode Submit your work to get feedback from unit tests ● Hint: you can use random.randint (a, b) to select random numbers between a and b. Hint: The behavior of sim_many_plays will simulate a single player and their overall winnings at the end of each play. For example, if they play 4 times and lose each time, the record of their overall winnings in each round would be [-1, -2, -3, -4].
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
i need help answering this question while using python
![1 def generate_lottery_numbers():
*** Generates a list of 5 random integers between 1 and 42,
inclusive, with no duplicates.
-234567891912345678928212222425252728298123458789¥12457898123科55拓望能卵卵乳酸發科5%的品
10
20
30
12 def count_matches (my_list, lottery_list):
40
50
Returns:
60
61 if
list: A list of lottery numbers
# Type your code here.
co
pass
""" Takes two lists of equal length representing the player's chosen
number list and the generated lottery list and returns the number of
between my_list and lottery_list.
matches
For example, count_matches ([10, 6, 20, 5, 7], [30, 6, 7, 40, 5])
will return 3, since both lists contain 5, 6, 7.
Parameters:
my_list (list): Your lottery numbers.
lottery list (list): A list of the winning numbers.
32 def play_lottery_once():
Returns:
int: The number of matching integers
# Type your code here.
pass
*** Uses generate_lottery_numbers () and count_matches () to return the
reward gained in playing the lottery one time. The lottery costs $1
to enter. The game award is determined according to the Table
derived from data published by the state of Georgia.
(If a player wins "one free play", we simply calculate this as
winning $1).
47 def sim_many_plays (n):
Returns:
int: The total dollar amount gained by playing the game
# Type your code here.
pass
""" Simulates a single person playing the lottery n times, to
determine their overall winnings at the end of each simulated lottery
Parameters:
n (int): The number of times the person plays the lottery.
Returns:
list: The total winnings after each of the n lotterys
pass
# Type your code here.
_name__ == "__main_":
seed = int(input('Enter a seed for the simulation: '))
random.seed (seed)
winnings = sim_many_plays(1000)
# Simulate 1000 plays by one person and plot the winnings.
plotwinsAcrossManyPlays (winnings)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faeb21d71-799d-47a2-8fd7-3cf7cc65097d%2F0cefc059-7a5f-45b4-8327-951ec7f3fb43%2Fz8d1lmn_processed.png&w=3840&q=75)
Transcribed Image Text:1 def generate_lottery_numbers():
*** Generates a list of 5 random integers between 1 and 42,
inclusive, with no duplicates.
-234567891912345678928212222425252728298123458789¥12457898123科55拓望能卵卵乳酸發科5%的品
10
20
30
12 def count_matches (my_list, lottery_list):
40
50
Returns:
60
61 if
list: A list of lottery numbers
# Type your code here.
co
pass
""" Takes two lists of equal length representing the player's chosen
number list and the generated lottery list and returns the number of
between my_list and lottery_list.
matches
For example, count_matches ([10, 6, 20, 5, 7], [30, 6, 7, 40, 5])
will return 3, since both lists contain 5, 6, 7.
Parameters:
my_list (list): Your lottery numbers.
lottery list (list): A list of the winning numbers.
32 def play_lottery_once():
Returns:
int: The number of matching integers
# Type your code here.
pass
*** Uses generate_lottery_numbers () and count_matches () to return the
reward gained in playing the lottery one time. The lottery costs $1
to enter. The game award is determined according to the Table
derived from data published by the state of Georgia.
(If a player wins "one free play", we simply calculate this as
winning $1).
47 def sim_many_plays (n):
Returns:
int: The total dollar amount gained by playing the game
# Type your code here.
pass
""" Simulates a single person playing the lottery n times, to
determine their overall winnings at the end of each simulated lottery
Parameters:
n (int): The number of times the person plays the lottery.
Returns:
list: The total winnings after each of the n lotterys
pass
# Type your code here.
_name__ == "__main_":
seed = int(input('Enter a seed for the simulation: '))
random.seed (seed)
winnings = sim_many_plays(1000)
# Simulate 1000 plays by one person and plot the winnings.
plotwinsAcrossManyPlays (winnings)
![Checkpoint A
Implement these functions from the template following the description (specification) in their docstring:
• generate_lottery_numbers()
• count_matches ()
• play_lottery_once()
sim_many_plays ()
By default, this project does not generate any interesting printed output. So, you should use one of these strategies in your work:
• Use Python Tutor to do incremental development, focusing on one function at a time in isolation
• Invent some of your own intermediate output, to get feedback while in develop mode
• Submit your work to get feedback from unit tests
Hint: you can use random.randint (a, b) to select random numbers between a and b.
Hint: The behavior of sim_many_plays will simulate a single player and their overall winnings at the end of each play. For example, if they
play 4 times and lose each time, the record of their overall winnings in each round would be [-1, -2, -3, -4].](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faeb21d71-799d-47a2-8fd7-3cf7cc65097d%2F0cefc059-7a5f-45b4-8327-951ec7f3fb43%2Fg6era0p_processed.png&w=3840&q=75)
Transcribed Image Text:Checkpoint A
Implement these functions from the template following the description (specification) in their docstring:
• generate_lottery_numbers()
• count_matches ()
• play_lottery_once()
sim_many_plays ()
By default, this project does not generate any interesting printed output. So, you should use one of these strategies in your work:
• Use Python Tutor to do incremental development, focusing on one function at a time in isolation
• Invent some of your own intermediate output, to get feedback while in develop mode
• Submit your work to get feedback from unit tests
Hint: you can use random.randint (a, b) to select random numbers between a and b.
Hint: The behavior of sim_many_plays will simulate a single player and their overall winnings at the end of each play. For example, if they
play 4 times and lose each time, the record of their overall winnings in each round would be [-1, -2, -3, -4].
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
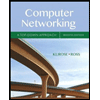
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
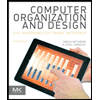
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
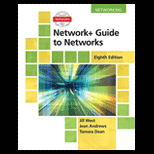
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
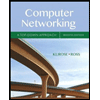
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
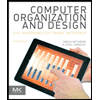
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
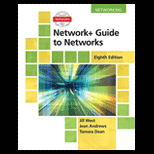
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
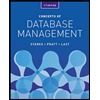
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
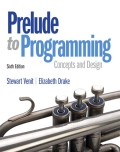
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
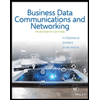
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY