How can I fix my error in Python.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
How can I fix my error in Python.
![The image shows a Python traceback error from a Jupyter notebook cell. Here is the transcription and explanation:
---
**Traceback (most recent call last):**
```
Input In [32], in <cell line: 22>()
```
19 `nf_frap_h = df_nf[['FRAP-H2O']]`
21 `fig, ax = plt.subplots(3, 2, figsize=(24, 24))`
22 `ax[0,0].draw_confidence_ellipse(f_tpc_h, f_teac_h, nf_tpc_h, nf_teac_h, 2, "TPC", "TEAC", "TPC-H2O vs TEAC-H2O", 50, 50)`
23 `ax[1,0].draw_confidence_ellipse(f_tpc_h, f_frap_h, nf_frap_h, 2, "TPC", "FRAP", "TPC-H2O vs FRAP-H2O", 50, 50)`
24 `ax[2,0].draw_confidence_ellipse(f_frap_h, f_teac_h, nf_teac_h, 2, "FRAP", "TEAC", "FRAP-H2O vs TEAC-H2O", 50, 50)`
**AttributeError**: `'AxesSubplot' object has no attribute 'draw_confidence_ellipse'`
---
### Explanation:
- **Code Explanation**:
- A DataFrame `df_nf` is being used to extract a column named `'FRAP-H2O'` into `nf_frap_h`.
- A figure and axes (`ax`) are created using `matplotlib.pyplot.subplots` with a grid of 3 rows and 2 columns, and a figure size of (24, 24).
- Three lines attempt to call a method `draw_confidence_ellipse` on different subplots to draw ellipses with specified parameters.
- **Error Explanation**:
- The error message indicates that the method `draw_confidence_ellipse` doesn’t exist for `AxesSubplot` objects. This suggests either a typo or that the method hasn’t been defined or imported into the current scope.
- **Potential Solution**:
- Ensure that the method `draw_confidence_ellipse` is defined or check for correct import statements.
- Verify if there is an alternative method for drawing ellipses, or if a custom](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F892e817a-9b32-4eeb-b8fc-5dd7ffde6479%2F30177e2f-ddf9-4702-ac62-47ce7575e698%2Fi9scnc9_processed.png&w=3840&q=75)
Transcribed Image Text:The image shows a Python traceback error from a Jupyter notebook cell. Here is the transcription and explanation:
---
**Traceback (most recent call last):**
```
Input In [32], in <cell line: 22>()
```
19 `nf_frap_h = df_nf[['FRAP-H2O']]`
21 `fig, ax = plt.subplots(3, 2, figsize=(24, 24))`
22 `ax[0,0].draw_confidence_ellipse(f_tpc_h, f_teac_h, nf_tpc_h, nf_teac_h, 2, "TPC", "TEAC", "TPC-H2O vs TEAC-H2O", 50, 50)`
23 `ax[1,0].draw_confidence_ellipse(f_tpc_h, f_frap_h, nf_frap_h, 2, "TPC", "FRAP", "TPC-H2O vs FRAP-H2O", 50, 50)`
24 `ax[2,0].draw_confidence_ellipse(f_frap_h, f_teac_h, nf_teac_h, 2, "FRAP", "TEAC", "FRAP-H2O vs TEAC-H2O", 50, 50)`
**AttributeError**: `'AxesSubplot' object has no attribute 'draw_confidence_ellipse'`
---
### Explanation:
- **Code Explanation**:
- A DataFrame `df_nf` is being used to extract a column named `'FRAP-H2O'` into `nf_frap_h`.
- A figure and axes (`ax`) are created using `matplotlib.pyplot.subplots` with a grid of 3 rows and 2 columns, and a figure size of (24, 24).
- Three lines attempt to call a method `draw_confidence_ellipse` on different subplots to draw ellipses with specified parameters.
- **Error Explanation**:
- The error message indicates that the method `draw_confidence_ellipse` doesn’t exist for `AxesSubplot` objects. This suggests either a typo or that the method hasn’t been defined or imported into the current scope.
- **Potential Solution**:
- Ensure that the method `draw_confidence_ellipse` is defined or check for correct import statements.
- Verify if there is an alternative method for drawing ellipses, or if a custom
![# Answer to Exercise 4
This code is written in Python and utilizes the `matplotlib` and `numpy` libraries, along with an imported function `confidence_ellipse`. The purpose of the code is to analyze and visualize the correlation between various datasets using confidence ellipses. Here is a breakdown of the code:
1. **Imports:**
- `import matplotlib.pyplot as plt`: Imports the `matplotlib.pyplot` library as `plt`, which is used for plotting graphs.
- `import numpy as np`: Imports the `numpy` library as `np`, commonly used for numerical operations.
- `from source.ellipses import confidence_ellipse`: Imports the `confidence_ellipse` function from a module named `source.ellipses`, used for drawing confidence ellipses.
2. **Data Preparation:**
- The code extracts specific columns from DataFrame objects `df_fer` and `df_nff` into different variables:
- `f_tpc_m`, `f_teac_m`, `f_frap_m`: Extracted from `df_fer` for `'TPC-MEOH'`, `'TEAC-MEOH'`, and `'FRAP-MEOH'`, respectively.
- `f_tpc_h`, `f_teac_h`, `f_frap_h`: Extracted from `df_fer` for `'TPC-H2O'`, `'TEAC-H2O'`, and `'FRAP-H2O'`.
- `nf_tpc_m`, `nf_teac_m`, `nf_frap_m`: Extracted from `df_nff` for `'TPC-MEOH'`, `'TEAC-MEOH'`, and `'FRAP-MEOH'`.
- `nf_tpc_h`, `nf_teac_h`, `nf_frap_h`: Extracted from `df_nff` for `'TPC-H2O'`, `'TEAC-H2O'`, and `'FRAP-H2O'`.
3. **Plotting:**
- `fig, ax = plt.subplots(3, 2, figsize=(24, 24))`: Creates a figure with a 3x2 grid of subplots, each with a size of 24x24 inches.
- Each subplot uses the `draw_confidence_ellipse` function to plot confidence ellipses for various data combinations:
- `ax[0,0]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F892e817a-9b32-4eeb-b8fc-5dd7ffde6479%2F30177e2f-ddf9-4702-ac62-47ce7575e698%2Ful0qzz8_processed.png&w=3840&q=75)
Transcribed Image Text:# Answer to Exercise 4
This code is written in Python and utilizes the `matplotlib` and `numpy` libraries, along with an imported function `confidence_ellipse`. The purpose of the code is to analyze and visualize the correlation between various datasets using confidence ellipses. Here is a breakdown of the code:
1. **Imports:**
- `import matplotlib.pyplot as plt`: Imports the `matplotlib.pyplot` library as `plt`, which is used for plotting graphs.
- `import numpy as np`: Imports the `numpy` library as `np`, commonly used for numerical operations.
- `from source.ellipses import confidence_ellipse`: Imports the `confidence_ellipse` function from a module named `source.ellipses`, used for drawing confidence ellipses.
2. **Data Preparation:**
- The code extracts specific columns from DataFrame objects `df_fer` and `df_nff` into different variables:
- `f_tpc_m`, `f_teac_m`, `f_frap_m`: Extracted from `df_fer` for `'TPC-MEOH'`, `'TEAC-MEOH'`, and `'FRAP-MEOH'`, respectively.
- `f_tpc_h`, `f_teac_h`, `f_frap_h`: Extracted from `df_fer` for `'TPC-H2O'`, `'TEAC-H2O'`, and `'FRAP-H2O'`.
- `nf_tpc_m`, `nf_teac_m`, `nf_frap_m`: Extracted from `df_nff` for `'TPC-MEOH'`, `'TEAC-MEOH'`, and `'FRAP-MEOH'`.
- `nf_tpc_h`, `nf_teac_h`, `nf_frap_h`: Extracted from `df_nff` for `'TPC-H2O'`, `'TEAC-H2O'`, and `'FRAP-H2O'`.
3. **Plotting:**
- `fig, ax = plt.subplots(3, 2, figsize=(24, 24))`: Creates a figure with a 3x2 grid of subplots, each with a size of 24x24 inches.
- Each subplot uses the `draw_confidence_ellipse` function to plot confidence ellipses for various data combinations:
- `ax[0,0]
Expert Solution

Step 1
Error
The error occurs because 'AxesSubplot' does not have a draw_confidence_ellipse method in its package.
- If you want to draw a confidence ellipse into the Axes object, try for a different alternative such as using the confidence_ellipse method.
- One example implementation is described in the following step.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
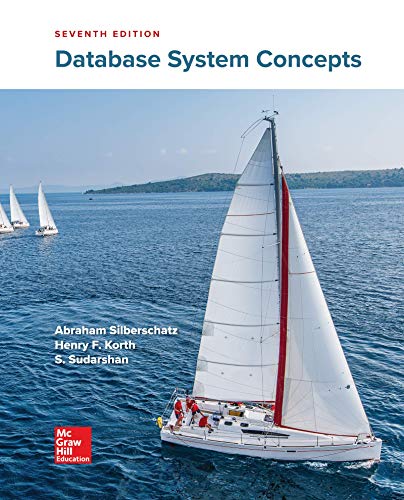
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
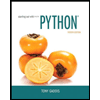
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
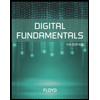
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
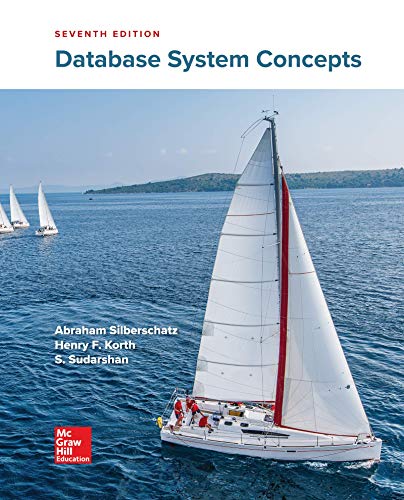
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
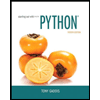
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
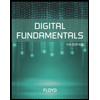
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
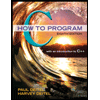
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
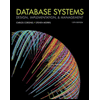
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
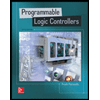
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education