Auto-graded programming assignments may use a Unit test to test small parts of a program. Unlike a Compare output test, which evaluates your program's output for specific input values, a Unit test evaluates individual functions to determines if each function: • is named correctly and has the correct parameters and return type • calculates and returns the correct value (or prints the correct output) In Python labs, the line if name =='_main_': is used to separate the main code from the functions' code so that each function can be unit tested. This example lab uses multiple unit tests to test the kilo_to pounds() function. Complete a program that takes a weight in kilograms as input, converts the weight to pounds, and then outputs the weight in pounds. 1 kilogram = 2.204 pounds (Ibs). Ex: If the input is: 10 the output is: 22.040000000000003 lbs Note: Your program must define the function def kilo_to_pounds (kilos)
Auto-graded programming assignments may use a Unit test to test small parts of a program. Unlike a Compare output test, which evaluates your program's output for specific input values, a Unit test evaluates individual functions to determines if each function: • is named correctly and has the correct parameters and return type • calculates and returns the correct value (or prints the correct output) In Python labs, the line if name =='_main_': is used to separate the main code from the functions' code so that each function can be unit tested. This example lab uses multiple unit tests to test the kilo_to pounds() function. Complete a program that takes a weight in kilograms as input, converts the weight to pounds, and then outputs the weight in pounds. 1 kilogram = 2.204 pounds (Ibs). Ex: If the input is: 10 the output is: 22.040000000000003 lbs Note: Your program must define the function def kilo_to_pounds (kilos)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Python. Need help fixing my code

Transcribed Image Text:Auto-graded programming assignments may use a Unit test to test small parts of a program. Unlike a Compare output test, which evaluates your program's output for specific input values, a Unit test
evaluates individual functions to determines if each function:
• is named correctly and has the correct parameters and return type
• calculates and returns the correct value (or prints the correct output)
In Python labs, the line if name =='_main_': is used to separate the main code from the functions' code so that each function can be unit tested.
This example lab uses multiple unit tests to test the kilo_to_pounds() function.
Complete a program that takes a weight in kilograms as input, converts the weight to pounds, and then outputs the weight in pounds. 1 kilogram = 2.204 pounds (Ibs).
Ex: If the input is:
10
the output is:
22.040000000000003 lbs
Note: Your program must define the function
def kilo_to_pounds (kilos)
The program below has an error in the kilo_to_pounds() function.
1. Try submitting the program for grading (click "Submit mode", then "Submit for grading"). Notice that the first two test cases fail, but the third test case passes. The first test case fails because the
program outputs the result from the kilo_to_pounds) function, which has an error. The second test case uses a Unit test to test the kilo_to_pounds) function, which fails.
2. Change the kilo_to_pounds() function to multiply the variable kilos by 2.204, instead of dividing. The return statement should be: return (kilos * 2.204); Submit again. Now the test cases
should all pas.
Note: A common error is to mistype a function name with the incorrect capitalization. Function names are case sensitive, so if a lab program asks for a kilo_to_pounds) function, a kilo_To Pounds()
function that works for you in develop mode will result in a failed unit test (the unit test will not be able to find kilo_to_pounds).
376584.2469792.qx3zay7
2/3
LAB
АCTVITY
11.7.1: Lab training: Unit tests to evaluate your program
Load default template.
main.py
#function to convert kilos to pounds
def kilo_to_pounds (kilos):
1
#return statement as specified in question
return (kilos 2.204)
5.

Transcribed Image Text:1: Compare output ^
Input
60
Your output
Your program produced no output
Expected output
132.24 lbs
2: Unit test a
Tests KiloToPounds(10) returns 22.040000000000003
Test feedback
kilo_to_pounds (10) correctly returned 22.040000000000003.
3: Unit test A
Tests KiloToPounds(0) returns 0.0
Test feedback
kilo_to_pounds (0) correctly returned 0.0.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
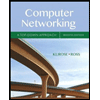
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
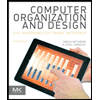
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
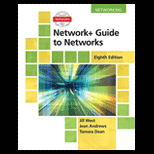
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
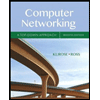
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
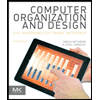
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
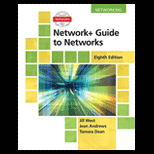
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
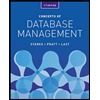
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
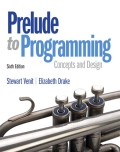
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
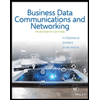
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY