Consider using the following Card class. public class Card { private String name; public Card() { name = ""; } public Card(String n) { name = n; } public String getName() { return name; } public boolean isExpired() { return false; } public String format() { return "Card holder: " + name; } } Use this class as a superclass to implement a hierarchy of related classes: Write declarations for each of the subclasses. For each subclass, supply private instance variables. Leave the bodies of the constructors and the format methods blank for now. Class Data IDCard ID number CallingCard Card number, PIN DriverLicesnse Expirationyear Important: DriverLicense is subclass of IDCard class Lab 8.2 Provide constructors for Card subclasses Implement constructors for each of the three subclasses. Each constructor should call the superclass constructor to set the name. Here is one example: public IDCard(String n, String id) { super(n); idNumber = id; } Lab 8.3 Declare format methods for subclasses Replace the implementation of the format method for the three subclasses. The methods should produce a formatted description of the card details. The subclass methods should call the superclass format method to get the formatted name of the cardholder.
Lab 8.1 Declare Card subclasses
Consider using the following Card class.
public class Card
{
private String name;
public Card()
{
name = "";
}
public Card(String n)
{
name = n;
}
public String getName()
{
return name;
}
public boolean isExpired()
{
return false;
}
public String format()
{
return "Card holder: " + name;
}
}
Use this class as a superclass to implement a hierarchy of related classes:
Write declarations for each of the subclasses. For each subclass, supply private instance variables. Leave the bodies of the constructors and the format methods blank for now.
Class | Data |
IDCard | ID number |
CallingCard | Card number, PIN |
DriverLicesnse | Expirationyear |
Important: DriverLicense is subclass of IDCard class
Lab 8.2 Provide constructors for Card subclasses
Implement constructors for each of the three subclasses. Each constructor should call the superclass constructor to set the name. Here is one example:
public IDCard(String n, String id)
{
super(n);
idNumber = id;
}
Lab 8.3 Declare format methods for subclasses
Replace the implementation of the format method for the three subclasses. The methods should produce a formatted description of the card details. The subclass methods should call the superclass format method to get the formatted name of the cardholder.
Lab 9 Unit Testing for three subclasses
Create each object for all three subclasses, print out each object information by invoking format method.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

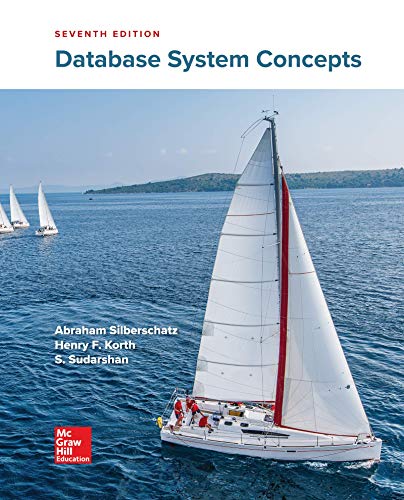
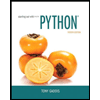
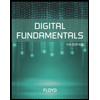
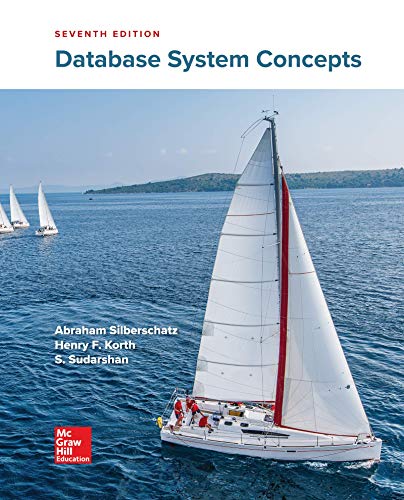
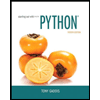
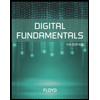
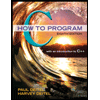
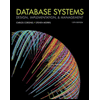
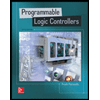