Complete the following using the attached diceType class as the base: Create a derived class that allows you to reset the number of sides on a die. (Hint: You will need to make one change in diceType.) Overload the << and >> operators.
***********Instructions*******************************************
Complete the following using the attached diceType class as the base:
- Create a derived class that allows you to reset the number of sides on a die. (Hint: You will need to make one change in diceType.)
- Overload the << and >> operators.
Note: You should test each step in a client program.
******************************************************************
//diceType.h
#ifndef H_diceType
#define H_diceType
class diceType
{
public:
diceType();
// Default constructor
// Sets numSides to 6 with a random numRolled from 1 - 6
diceType(int);
// Constructor to set the number of sides of the dice
int roll();
// Function to roll a dice.
// Randomly generates a number between 1 and numSides
// and stores the number in the instance variable numRolled
// and returns the number.
int getNum() const;
// Function to return the number on the top face of the dice.
// Returns the value of the instance variable numRolled.
private:
int numSides;
int numRolled;
};
#endif // H_diceType
========================================================
//diceTypeImp.cpp
//Implementation File for the class clockType
#include <iostream>
#include <cstdlib>
#include <ctime>
#include "diceType.h"
using namespace std;
diceType::diceType()
{
srand(time(nullptr));
numSides = 6;
numRolled = (rand() % 6) + 1;
}
diceType::diceType(int sides)
{
srand(time(0));
numSides = sides;
numRolled = (rand() % numSides) + 1;
}
int diceType::roll()
{
numRolled = (rand() % numSides) + 1;
return numRolled;
}
int diceType::getNum() const
{
return numRolled;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

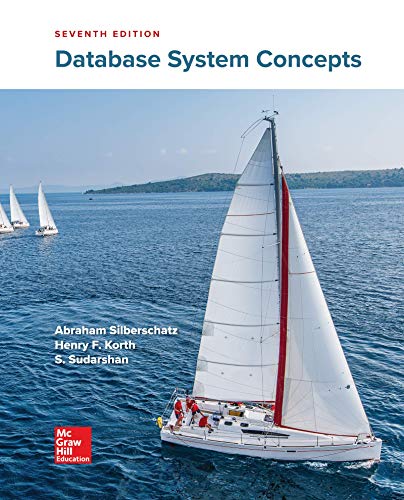
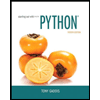
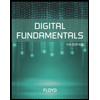
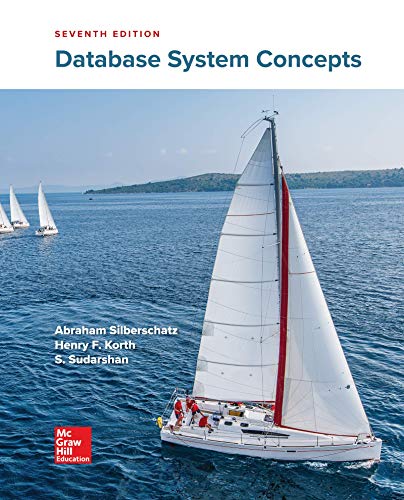
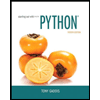
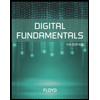
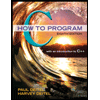
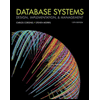
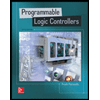