* This class describes a tweet. A tweet has a message in it, a unique ID, * a count of the number of likes, and a count of the number of times it * has been retweeted. In addition, the Tweet class will have a static * variable to count the total number of tweets to ever be created * (retweets don't count as a new tweet). * * You may NOT import any library class. * * * */ public class Tweet { /** * This constructor creates a tweet with the given message. * Assume that the message is not null because only the tweet() method * from the TwitterUser class will call this constructor. There are * no length requirements on Tweets. * * The very first tweet to ever be created will have an ID of 0, the * next one will have 1, and so on and so forth. It may help you to use * the static count variable to set the ID. * * You will have to initialize other instance variables appropriately. * @param message the text of the tweet */ public Tweet(String message) { throw new UnsupportedOperationException("Implement this"); /** * Makes appropriate changes in the instance variables to reflect that * this tweet has been liked. */ public void like() { throw new UnsupportedOperationException("Implement this"); } /** * * @return the ID of this tweet */ public long getID() { /** * * @return the text of this tweet */ public String getText() { throw new UnsupportedOperationException("Implement this"); } /** * * @return the number of likes this tweet has received */ public long getNumLikes() { throw new UnsupportedOperationException("Implement this"); } /** * * @return the number of times this tweet has been retweeted */ public long getNumRetweets() { throw new UnsupportedOperationException("Implement this"); } /** * * @return the total number of tweets to ever be created */ public static long getNumTweets() { throw new UnsupportedOperationException("Implement this"); } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
/**
* This class describes a tweet. A tweet has a message in it, a unique ID,
* a count of the number of likes, and a count of the number of times it
* has been retweeted. In addition, the Tweet class will have a static
* variable to count the total number of tweets to ever be created
* (retweets don't count as a new tweet).
*
* You may NOT import any library class.
*
*
*
*/
public class Tweet {
/**
* This constructor creates a tweet with the given message.
* Assume that the message is not null because only the tweet() method
* from the TwitterUser class will call this constructor. There are
* no length requirements on Tweets.
*
* The very first tweet to ever be created will have an ID of 0, the
* next one will have 1, and so on and so forth. It may help you to use
* the static count variable to set the ID.
*
* You will have to initialize other instance variables appropriately.
* @param message the text of the tweet
*/
public Tweet(String message) {
throw new UnsupportedOperationException("Implement this");
/**
* Makes appropriate changes in the instance variables to reflect that
* this tweet has been liked.
*/
public void like() {
throw new UnsupportedOperationException("Implement this");
}
/**
*
* @return the ID of this tweet
*/
public long getID() {
/**
*
* @return the text of this tweet
*/
public String getText() {
throw new UnsupportedOperationException("Implement this");
}
/**
*
* @return the number of likes this tweet has received
*/
public long getNumLikes() {
throw new UnsupportedOperationException("Implement this");
}
/**
*
* @return the number of times this tweet has been retweeted
*/
public long getNumRetweets() {
throw new UnsupportedOperationException("Implement this");
}
/**
*
* @return the total number of tweets to ever be created
*/
public static long getNumTweets() {
throw new UnsupportedOperationException("Implement this");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 4 images

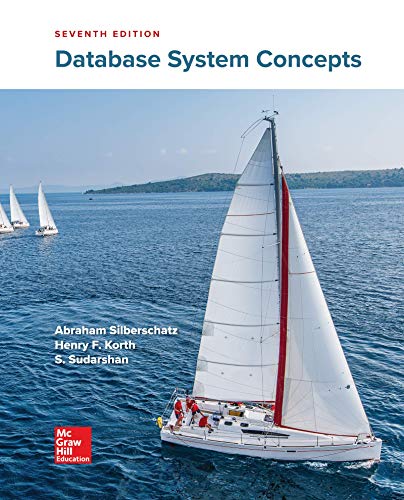
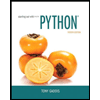
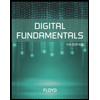
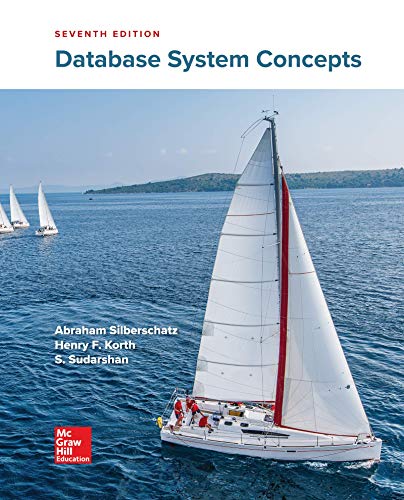
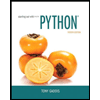
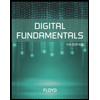
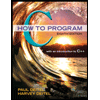
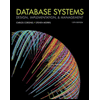
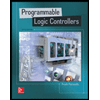