c) costPerPassenger i) 11) a) addPassengers i) ii) 111) iv) v) vi) Takes in an int number of stops and returns a double representing the cost for the passenger to ride the specified number of stops. Use the descriptions for the rate and photoFee fields to determine the cost per passenger. 1) 2) vii) double rate the cost of one run double photoFees - the fees to purchase the mandatory photo package o (a) A passenger may ride the roller coaster multiple times and the fee must only be paid once. Takes in an int number of stops and a String[] representing the passengers in a group. The roller coaster should be able to travel the requested number of runs without exceeding maxNumRuns, or the passengers cannot be added. For each passenger, add them once to the passengers array, starting from the lowest available index. 1) Only add the passengers if there are enough seats for ALL of the input passengers on the roller coaster AND if the roller coaster can complete the number of stops specified! A seat is available if the corresponding index in the array does not already have a passenger assigned to it. What value is used to represent an empty seat? 2) 3) Do not assume that passengers already on the roller coaster will always be at the front of the array; empty seats may be spread throughout the array. Note: Duplicate passenger names are allowed. 4) If all passengers in the group fit on the roller coaster, charge each passenger for their ride. Assume that each passenger is riding for the same number of stops. (Charge the new passengers added, not the ones already riding) 1) Reuse code. What method have we already created to charge a passenger? 2) Increase runsSinceInspection. No matter how many passengers there are, runs Since Inspection should only be increased once per stop. Returns true if the entire group of passengers can fit on the roller coaster and the rollercoaster can travel the specified number of stops. Returns false if any passenger in the group cannot fit on the roller coaster or the rollercoaster cannot travel the given number of stops.
Given the following code and instructions:
I'm having trouble implementing these last 2 methods.
Method for finding empty seats in the code already if it helps the process.
Ride.Java contains:
public abstract boolean inspectRide(String[] components);
public abstract double costPerPassenger(int numberOfStops);
public abstract boolean addPassengers(int numberOfStops, String[] newPassengers);
![public class RollerCoaster extends Ride {
private double rate;
private double photoFees;
private int maxNumRuns;
}
public RollerCoaster (String id, int runsSince Inspection, String[] passengers, double rate, double photoFees, int maxNumRuns) {
super(id, runsSince Inspection, passengers);
this.rate= rate;
}
public RollerCoaster (String id, int runsSince Inspection, int maxNumRuns) {
this(id, runssince Inspection, new String[4], 10.0, 15.0, maxNumRuns);
this.photoFees = photoFees;
this.maxNumRuns = maxNumRuns;
}
public RollerCoaster(String id) {
this(id, 0, new String[4], 10.0, 15.0, 200);
}
@Override
public boolean canRun(int numberOfRuns) {
return numberOfRuns >= 0 && (runsSinceInspection + numberOfRuns) <= maxNumRuns;
}
@Override
public boolean inspectRide (String[] components) {
boolean tracksClear = false;
boolean brakesOk = false;
for (String component : components) {
if (component.equalsIgnoreCase("Tracks Clear")) {
tracksClear = true;
}
} else if (component.equalsIgnoreCase("Brakes Ok")) {
brakes0k= true;
}
}
if (tracksClear && brakes0k) {
runsSince Inspection = 0;
return true;
} else {
}
return false;
}
// Helper Class for empty seats of necessary.
private int countEmptySeats() {
int count = 0;
for (String passenger : passengers) {
if (passenger == null) {
count++;
}
}
return count;
}
// Overrides the toString method from Ride
@Override
public String toString() {
return String.format("Roller Coaster %s has run %d times and has earned $ %.2f. It can only run %d more times.
"It costs $%.2f per ride and there is a one-time photo fee of $%.2f.",
id, runsSinceInspection, earnings, maxNumRuns - runsSince Inspection, rate, photoFees);
+](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F295c436f-1881-4143-81a2-89f6f40be87f%2F91532488-786e-4dbd-a397-c8123bb12c79%2F60b8uns_processed.png&w=3840&q=75)
![c) costPerPassenger
i)
ii)
a) addPassengers
i)
ii)
iii)
iv)
V)
vi)
Takes in an int number of stops and returns a double representing the cost for the passenger to ride
the specified number of stops.
Use the descriptions for the rate and photoFee fields to determine the cost per passenger.
1)
2)
vii)
double rate - the cost of one run
double photoFees – the fees to purchase the mandatory photo package o
-
(a)
A passenger may ride the roller coaster multiple times and the fee must only be
paid once.
Takes in an int number of stops and a String[] representing the passengers in a group.
The roller coaster should be able to travel the requested number of runs without exceeding
maxNumRuns, or the passengers cannot be added.
For each passenger, add them once to the passengers array, starting from the lowest available
index.
1)
Only add the passengers if there are enough seats for ALL of the input passengers on the
roller coaster AND if the roller coaster can complete the number of stops specified!
A seat is available if the corresponding index in the array does not already have a
passenger assigned to it. What value is used to represent an empty seat?
2)
3)
Do not assume that passengers already on the roller coaster will always be at the front of
the array; empty seats may be spread throughout the array.
4)
Note: Duplicate passenger names are allowed.
If all passengers in the group fit on the roller coaster, charge each passenger for their ride.
Assume that each passenger is riding for the same number of stops. (Charge the new
passengers added, not the ones already riding)
1)
2) Reuse code. What method have we already created to charge a passenger?
Increase runsSinceInspection. No matter how many passengers there are, runsSinceInspection
should only be increased once per stop.
Returns true if the entire group of passengers can fit on the roller coaster and the rollercoaster can
travel the specified number of stops.
Returns false if any passenger in the group cannot fit on the roller coaster or the rollercoaster
cannot travel the given number of stops.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F295c436f-1881-4143-81a2-89f6f40be87f%2F91532488-786e-4dbd-a397-c8123bb12c79%2Fdfe1d4_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps

After implementing the suggested fix as described in the 'Follow-Up Questions', I'm still getting
-----Add Passengers-----
true
Roller Coaster Roller1 has run 10 times and has earned $363.75. It can only run 40 more times. It costs $32.43 per ride and there is a one-time photo fee of $40.32.
true
Roller Coaster Roller2 has run 15 times and has earned $0.00. It can only run 5 more times. It costs $12.43 per ride and there is a one-time photo fee of $4.00.
Instead of:
true
Roller Coaster Roller1 has run 10 times and has earned $607.41. It can only run 40 more times. It costs $32.43 per ride and there is a one-time photo fee of $40.32.
true
Roller Coaster Roller2 has run 15 times and has earned $132.30. It can only run 5 more times. It costs $12.43 per ride and there is a one-time photo fee of $4.00
I'm still having a bit of trouble implementing the charging inside the addPassenger.
Where "In addPassengers(...), if passenger from the input array gets added to the passengers array, they should be charged (use the method that charges a single passenger to charge each one)."
Using the Driver:
public static void main(String[] args) {
System.out.println("-----Roller Coaster Test-----");
RollerCoaster normalRoller = new RollerCoaster("Roller1", 5, new String[]{null, "A", "B", null, "C", "D",
null},
32.43, 40.32, 50);
RollerCoaster normalRoller2 = new RollerCoaster("Roller2", 10, new String[]{"A", "A", null, "B", null, "A",
"B"}, 12.43, 4.0, 20);
RollerCoaster brokenRoller = new RollerCoaster("Roller3", 50, new String[]{null, null, null}, 10.0, 10.0, 50);
RollerCoaster rollerDiffConst = new RollerCoaster("Roller4", 10, 100);
RollerCoaster rollerDiffConst2 = new RollerCoaster("Roller5");
RollerCoaster dupRoller = new RollerCoaster("Roller1", 5, new String[]{null, "A", "B", null, "C", "D",
null},
32.43, 40.32, 50);
System.out.println("\n-----Add Passengers-----");
String[] waiting1 = new String[]{"C", "D", "E"};
String[] waiting2 = new String[]{"A", "A", "B", "C"};
String[] waiting3 = new String[]{"A", "A"};
System.out.println(normalRoller.addPassengers(5, waiting1));
System.out.println(normalRoller);
System.out.println(normalRoller2.addPassengers(5, waiting2));
System.out.println(normalRoller2);
System.out.println(normalRoller2.addPassengers(5, waiting3));
System.out.println(normalRoller2);
System.out.println(brokenRoller.addPassengers(5, waiting3));
System.out.println(brokenRoller);
System.out.println(rollerDiffConst.addPassengers(-5, waiting1));
System.out.println(rollerDiffConst);
System.out.println(rollerDiffConst2.addPassengers(1000, waiting1));
System.out.println(rollerDiffConst2);
}
}
I should be getting the following results for the 2 true cases:
true Roller Coaster Roller1 has run 10 times and has earned $607.41. It can only run 40 more times. It costs $32.43 per ride and there is a one-time photo fee of $40.32.
true Roller Coaster Roller2 has run 15 times and has earned $132.30. It can only run 5 more times. It costs $12.43 per ride and there is a one-time photo fee of $4.00.
But I've been getting 363.75 for the first case and 0.00 for the 2nd. I've attached both completed programs.
![public class RollerCoaster extends Ride {
private double rate;
private double photoFees;
private int maxNumRuns;
public RollerCoaster (String id, int runsSince Inspection, String[] passengers, double rate, double photoFees, int maxNumRuns) {
super(id, runsSinceInspection, passengers);
this.rate= rate;
}
public RollerCoaster (String id, int runsSince Inspection, int maxNumRuns) {
this(id, runsSince Inspection, new String[4], 10.0, 15.0, maxNumRuns);
this.photoFees = photoFees;
this.maxNumRuns = maxNumRuns;
}
public Roller Coaster (String id) {
this(id, 0, new String[4], 10.0, 15.0, 200);
}
@Override
public boolean canRun(int numberOfRuns) {
return numberOfRuns >= 0 && (runsSince Inspection + numberOfRuns) <= maxNumRuns;
}
@Override
public double costPerPassenger (int numberOfStops) {
return numberOfStops * (rate + photoFees);
}
@Override
public boolean inspectRide (String[] components) {
}
boolean tracksClear = false;
boolean brakes0k = false;
for (String component : components) {
if (component.equalsIgnoreCase ("Tracks Clear")) {
tracksClear = true;
} else if (component.equals IgnoreCase ("Brakes Ok")) {
brakes0k = true;
}
}
if (tracksClear && brakes0k) {
runsSince Inspection = 0;
return true;
} else {
}
return false;
|
}
@Override
public boolean addPassengers (int numberOfStops, String[] newPassengers) {
// Check if there are enough seats and if the roller coaster can travel the specified number of stops
if (countEmptySeats() >= newPassengers.length && canRun(numberOfStops)) {
}
// Iterate through the new passengers and add them to empty seats
int emptySeat Index = 0;
for (String passenger: newPassengers) {
if (emptySeat Index < passengers.length && passengers [emptySeat Index] == null) {
passengers [emptySeat Index] = passenger;
// Charge the new passenger for their ride
}
}
chargePassenger (numberOfStops);
// Implement charging logic (e.g., store passengerCost somewhere)
emptySeat Index++; // Move to the next empty seat
}
// Increase runsSince Inspection only once per stop
runsSince Inspection += numberOfStops;
return true; // All passengers fit and can travel the specified number of stops
} else {
return false; // Some passengers cannot fit or the roller coaster cannot travel the given number of stops
}
private int countEmptySeats() {
int count = 0;
for (String passenger : passengers) {
if (passenger == null) {
count++;
}
}
return count;
}
// Overrides the toString method from Ride
@Override
public String toString() {
return String.format("Roller Coaster %s has run %d times and has earned $ %.2f. It can only run %d more times.
"It costs $%.2f per ride and there is a one-time photo fee of $ %.2f.",
id, runsSince Inspection, earnings, maxNumRuns - runsSince Inspection, rate, photoFees);](https://content.bartleby.com/qna-images/question/295c436f-1881-4143-81a2-89f6f40be87f/75c086d9-a3a6-407e-9f73-35b7da58b4da/ibynemh_thumbnail.png)
![public abstract class Ride {
protected final String id;
protected double earnings;
}
protected int runsSince Inspection;
protected String[] passengers;
public Ride (String id, int runsSince Inspection, String[] passengers) {
this.id = id;
this.earnings = 0;
this.runsSince Inspection = runsSince Inspection;
this.passengers = new String[passengers.length];
for (int i = 0; i < passengers.length; i++) {
this.passengers [i] passengers[i];
}
}
public Ride (String id, String[] passengers) {
this(id, 0, passengers);
}
public abstract boolean canRun(int number of Runs);
public abstract boolean inspectRide (String[] components);
public abstract double costPerPassenger (int numberOfStops);
public abstract boolean addPassengers (int numberOfStops, String[] newPassengers);
public String getPassengerList() {
String passengerList = "Passenger List for + id + ":\n";
for (String passenger
passengers) {
}
}
public final void chargePassenger (int numberOfStops) {
earnings += costPerPassenger (numberOfStops);
}
=
if (passenger != null) {
}
}
return passengerList.trim();
public boolean removePassenger (String passengerName) {
for (int i = 0; i < passengers.length; i++) {
if (passengers[i] != null && passengers [i].equals IgnoreCase (passengerName)) {
passengers[i] = null;
return true;
}
passengerList += passenger + "\n";
}
}
return false;
@Override
public boolean equals(Object obj) {
if (this ==
obj) {
return true;
}
if (obj == null || getClass() != obj.getClass()) {
return false;
}
Ride other= (Ride) obj;
return id.equals(other.id) && runsSince Inspection == other.runsSince Inspection;
@Override
public String toString() {
return id + " has run " + runsSinceInspection + times and has earned $" + earnings
+](https://content.bartleby.com/qna-images/question/295c436f-1881-4143-81a2-89f6f40be87f/75c086d9-a3a6-407e-9f73-35b7da58b4da/vqh3iap_thumbnail.png)
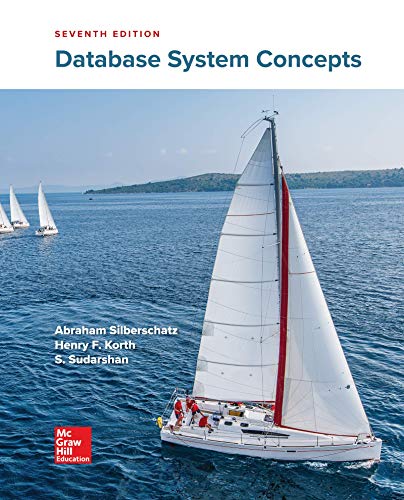
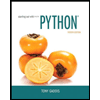
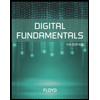
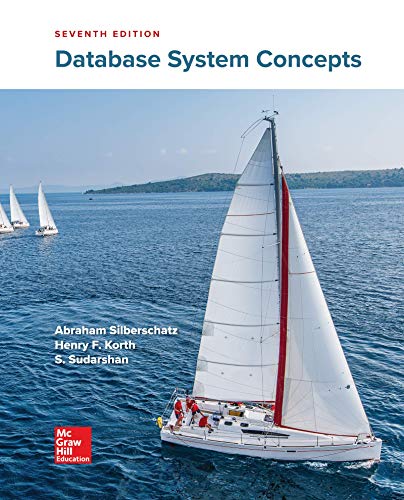
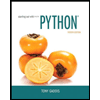
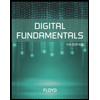
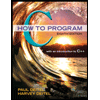
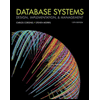
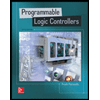