I need help fixing a java program that meets its requirements described in the image, over the code below. import java.util.Scanner; import java.util.Random; public class LabProgram { public static String coinFlip(Random rand) { int randomValue = rand.nextInt(2); // Generates a random value 0 or 1 if (randomValue == 1) { return "Heads"; } else { return "Tails"; } } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Random rand = new Random(2); // Seed used in develop mode int numFlips = scnr.nextInt(); // Read the desired number of coin flips for (int i = 0; i < numFlips; i++) { String result = coinFlip(rand); // Call coinFlip method System.out.print(result + " "); // Output the result } } }
I need help fixing a java program that meets its requirements described in the image, over the code below.
import java.util.Scanner;
import java.util.Random;
public class LabProgram {
public static String coinFlip(Random rand) {
int randomValue = rand.nextInt(2); // Generates a random value 0 or 1
if (randomValue == 1) {
return "Heads";
} else {
return "Tails";
}
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Random rand = new Random(2); // Seed used in develop mode
int numFlips = scnr.nextInt(); // Read the desired number of coin flips
for (int i = 0; i < numFlips; i++) {
String result = coinFlip(rand); // Call coinFlip method
System.out.print(result + " "); // Output the result
}
}
}


1. Input: rand (a Random object for generating random numbers)
2. Generate a random integer randomValue using rand.nextInt(2), which results in 0 or 1.
3. If randomValue is equal to 1:
4. Return "Heads".
5. Else:
6. Return "Tails".
7. Create a Scanner object scnr to read input from the user.
8. Create a Random object rand with a seed value (e.g., 2).
9. Read the desired number of coin flips (numFlips) from the user.
10. For i from 0 to numFlips - 1:
11. Call the coinFlip method with rand to get the result of a coin flip and store it in the variable result.
12. Output the result with a newline character by using System.out.println(result).
13. End of the loop.
Execution starts with main().
Step by step
Solved in 4 steps with 2 images

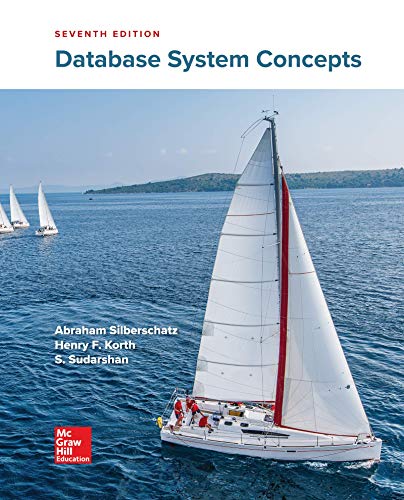
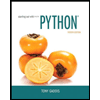
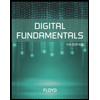
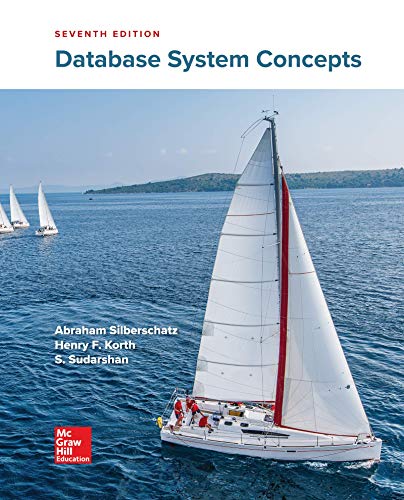
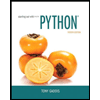
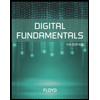
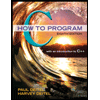
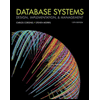
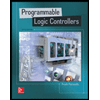