This is my program. I need help with the math. import java.util.*; import javax.swing.JOptionPane; public class Craps { public static void main(String[] args) { int Bet, Die; int WinC = 0, LossC = 0, GameC = 0, GameN = 1; double startingBal = 0, curBal = 0; double balD, balDe, balDec, balI, balIn, balInc; char Answer = '\0'; Scanner crapsGame = new Scanner(System.in); System.out.println("Welcome to the Craps Game"); curBal = StartingBal(startingBal); System.out.println("Your starting balance is: $" + curBal); do { System.out.println("Please input your bet for the game>> "); Bet = crapsGame.nextInt(); System.out.println("Game #" + GameN + " Starting with bet of: $" + Bet ); if (Bet < 1 || Bet > curBal) { System.out.println("Invalid bet – Your balance is only: $" + curBal); while (Bet < 1 || Bet > curBal) { System.out.println("Please input your bet for the game>> "); Bet = crapsGame.nextInt(); if (Bet > 1 && Bet <= curBal) { break; } else { System.out.println("Invalid bet – Your balance is only: $" + curBal); System.out.println("Please input your bet for the game>> $"); Bet = crapsGame.nextInt(); } } } Die = dieRoll(); System.out.println("Initial Dice Roll: " + Die + " -- Roll " + Die + " Again To Win" ); if (Die == 7 || Die == 11) { System.out.println("You Won!"); GameN += 1; GameC += 1; WinC += 1; curBal += Bet; System.out.println("Your current balance is $" + curBal); } else if(Die == 2 || Die == 3 || Die == 12) { System.out.println("You Lost!"); GameN += 1; GameC += 1; LossC += 1; curBal -= Bet; System.out.println("Your Current Balance is $" + curBal); } else { while(true) { int antTry = dieRoll(); System.out.println("Your next roll was a " + antTry); if(antTry == Die) { System.out.println("You Won!"); WinC += 1; curBal += Bet; System.out.println("Your current balance is $" + curBal); GameC += 1; GameN += 1; break; } else if(antTry == 7) { System.out.println("You Lost!"); LossC += 1; curBal -= Bet; System.out.println("Your current balance is $" + curBal); GameC += 1; GameN += 1; break; } } } if (curBal == 0) { System.out.println("Uh Oh! You Are Uneligible To Play!"); break; } System.out.println("Type Yes To Play Again!"); Answer = crapsGame.next().charAt(0); } while (Answer == 'y' || Answer == 'Y' ); if (curBal != 0 ){ System.out.println("Aww... You Done Playing :("); } System.out.println("Your ending account balance is: $" + curBal); if (curBal < startingBal) { balD = (startingBal - curBal); //balDe = (startingBal - balD); //balDec = (balDe / startingBal); System.out.println("Your starting balance has decreased by $" + (balD)); //+ " //(-% + balDec + )); } else if (curBal > startingBal) { balI = (curBal - startingBal); //balIn = (balI - startingBal); //balInc = (balIn / curBal); System.out.println("Your starting balance has increased by $" + (balI)); //+ " (+%" + balInc + ")"); } System.out.println("Of the " + GameC + " games played, you have won " + WinC + " games (" +(((GameC - LossC)* 100/ GameC) + "%) and lost " + LossC + " games (" + (((GameC - WinC)*100) / GameC)) + "%)"); if (curBal == 0 || Answer != 'y' || Answer != 'Y'){ System.out.println("Thank You For Playing, Play Again to Get More $$$!!"); } } OUTPUT SHOUD BE Sadly, you are no longer eligible to play Your ending account balance is: $ 0.00 Your starting balance has decreased by $200.00 (-100%) Of the 10 games played, you have won 3 games (30.0 %) and lost 7 games (70.0 %
This is my program. I need help with the math.
import java.util.*;
import javax.swing.JOptionPane;
public class Craps
{
public static void main(String[] args)
{
int Bet, Die;
int WinC = 0, LossC = 0, GameC = 0, GameN = 1;
double startingBal = 0, curBal = 0;
double balD, balDe, balDec, balI, balIn, balInc;
char Answer = '\0';
Scanner crapsGame = new Scanner(System.in);
System.out.println("Welcome to the Craps Game");
curBal = StartingBal(startingBal);
System.out.println("Your starting balance is: $" + curBal);
do
{
System.out.println("Please input your bet for the game>> ");
Bet = crapsGame.nextInt();
System.out.println("Game #" + GameN + " Starting with bet of: $" + Bet );
if (Bet < 1 || Bet > curBal)
{
System.out.println("Invalid bet – Your balance is only: $" + curBal);
while (Bet < 1 || Bet > curBal)
{
System.out.println("Please input your bet for the game>> ");
Bet = crapsGame.nextInt();
if (Bet > 1 && Bet <= curBal)
{
break;
}
else
{
System.out.println("Invalid bet – Your balance is only: $" + curBal);
System.out.println("Please input your bet for the game>> $");
Bet = crapsGame.nextInt();
}
}
}
Die = dieRoll();
System.out.println("Initial Dice Roll: " + Die + " -- Roll " + Die + " Again To Win" );
if (Die == 7 || Die == 11)
{
System.out.println("You Won!");
GameN += 1;
GameC += 1;
WinC += 1;
curBal += Bet;
System.out.println("Your current balance is $" + curBal);
}
else if(Die == 2 || Die == 3 || Die == 12)
{
System.out.println("You Lost!");
GameN += 1;
GameC += 1;
LossC += 1;
curBal -= Bet;
System.out.println("Your Current Balance is $" + curBal);
}
else
{
while(true)
{
int antTry = dieRoll();
System.out.println("Your next roll was a " + antTry);
if(antTry == Die)
{
System.out.println("You Won!");
WinC += 1;
curBal += Bet;
System.out.println("Your current balance is $" + curBal);
GameC += 1;
GameN += 1;
break;
}
else if(antTry == 7)
{
System.out.println("You Lost!");
LossC += 1;
curBal -= Bet;
System.out.println("Your current balance is $" + curBal);
GameC += 1;
GameN += 1;
break;
}
}
}
if (curBal == 0) {
System.out.println("Uh Oh! You Are Uneligible To Play!");
break;
}
System.out.println("Type Yes To Play Again!");
Answer = crapsGame.next().charAt(0);
}
while (Answer == 'y' || Answer == 'Y' );
if (curBal != 0 ){
System.out.println("Aww... You Done Playing :(");
}
System.out.println("Your ending account balance is: $" + curBal); if (curBal < startingBal)
{
balD = (startingBal - curBal);
//balDe = (startingBal - balD);
//balDec = (balDe / startingBal);
System.out.println("Your starting balance has decreased by $" + (balD));
//+ " //(-% + balDec + ));
}
else if (curBal > startingBal)
{
balI = (curBal - startingBal);
//balIn = (balI - startingBal);
//balInc = (balIn / curBal);
System.out.println("Your starting balance has increased by $" + (balI)); //+ " (+%" + balInc + ")");
}
System.out.println("Of the " + GameC + " games played, you have won " + WinC + " games (" +(((GameC - LossC)* 100/ GameC) + "%) and lost "
+ LossC + " games (" + (((GameC - WinC)*100) / GameC)) + "%)");
if (curBal == 0 || Answer != 'y' || Answer != 'Y'){
System.out.println("Thank You For Playing, Play Again to Get More $$$!!");
}
}
OUTPUT SHOUD BE
Sadly, you are no longer eligible to play
Your ending account balance is: $ 0.00
Your starting balance has decreased by $200.00 (-100%)
Of the 10 games played, you have won 3 games (30.0 %) and lost 7 games (70.0 %)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

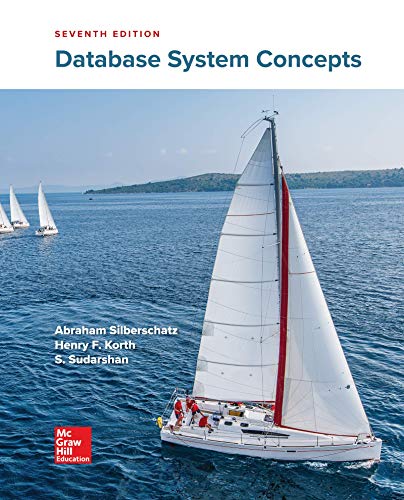
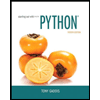
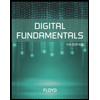
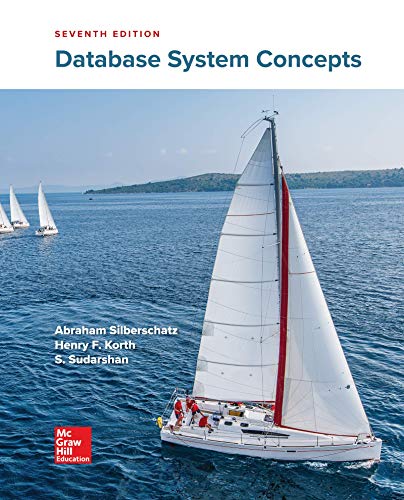
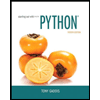
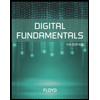
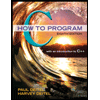
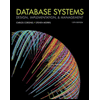
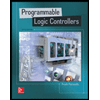