Finish the Java program: Code: import java.awt.Point; import java.util.Random; class Main { publicstaticvoid main(String[] args) { Point player1_location, player2_location; System.out.println("This program randomly generates (x,y) locations"); System.out.println("between 1-10 for two players, repeating until"); System.out.println("one of their coordinates are the same for both players."); do { player1_location = randomNewPlayerLocation(); player2_location = randomNewPlayerLocation(); System.out.println("Player1 location: (" + player1_location.x +"," + player1_location.y + ")"); System.out.println("Player2 location: (" + player2_location.x +"," + player2_location.y + ")"); System.out.println(); // DON'T CHANGE ANY OF THE ABOVE CODE }while( /* WRITE YOUR LOOP CONDITION HERE */ ); } // Generates random (x,y) coordinates between 1-10, then // returns a Point object with those coordinates. static Point randomNewPlayerLocation() { // WRITE YOUR FUNCTION BODY HERE } } Sample outputs in the picture.
Finish the Java program: Code: import java.awt.Point; import java.util.Random; class Main { publicstaticvoid main(String[] args) { Point player1_location, player2_location; System.out.println("This program randomly generates (x,y) locations"); System.out.println("between 1-10 for two players, repeating until"); System.out.println("one of their coordinates are the same for both players."); do { player1_location = randomNewPlayerLocation(); player2_location = randomNewPlayerLocation(); System.out.println("Player1 location: (" + player1_location.x +"," + player1_location.y + ")"); System.out.println("Player2 location: (" + player2_location.x +"," + player2_location.y + ")"); System.out.println(); // DON'T CHANGE ANY OF THE ABOVE CODE }while( /* WRITE YOUR LOOP CONDITION HERE */ ); } // Generates random (x,y) coordinates between 1-10, then // returns a Point object with those coordinates. static Point randomNewPlayerLocation() { // WRITE YOUR FUNCTION BODY HERE } } Sample outputs in the picture.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Finish the Java
Code:
import java.awt.Point;
import java.util.Random;
class Main
{
publicstaticvoid main(String[] args)
{
Point player1_location, player2_location;
System.out.println("This program randomly generates (x,y) locations");
System.out.println("between 1-10 for two players, repeating until");
System.out.println("one of their coordinates are the same for both players.");
do
{
player1_location = randomNewPlayerLocation();
player2_location = randomNewPlayerLocation();
System.out.println("Player1 location: (" + player1_location.x
+"," + player1_location.y + ")");
System.out.println("Player2 location: (" + player2_location.x
+"," + player2_location.y + ")");
System.out.println();
// DON'T CHANGE ANY OF THE ABOVE CODE
}while( /* WRITE YOUR LOOP CONDITION HERE */ );
}
// Generates random (x,y) coordinates between 1-10, then
// returns a Point object with those coordinates.
static Point randomNewPlayerLocation()
{
// WRITE YOUR FUNCTION BODY HERE
}
}
Sample outputs in the picture.

Transcribed Image Text:**Sample Program Output for Educational Website**
This program randomly generates (x, y) locations between 1-10 for two players, repeating until one of their coordinates are the same for both players.
### Sample Outputs:
1.
- Player1 location: (8, 7)
- Player2 location: (2, 9)
2.
- Player1 location: (9, 9)
- Player2 location: (4, 1)
3.
- Player1 location: (3, 8)
- Player2 location: (3, 8)
---
4.
- Player1 location: (10, 3)
- Player2 location: (3, 1)
5.
- Player1 location: (1, 5)
- Player2 location: (9, 2)
6.
- Player1 location: (4, 9)
- Player2 location: (7, 1)
7.
- Player1 location: (10, 7)
- Player2 location: (2, 1)
8.
- Player1 location: (6, 7)
- Player2 location: (4, 5)
9.
- Player1 location: (8, 5)
- Player2 location: (4, 2)
10.
- Player1 location: (1, 6)
- Player2 location: (9, 6)
The program stops executing when one of the coordinates matches for both players, as seen in scenarios where the coordinates become equal.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
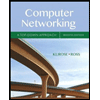
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
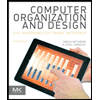
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
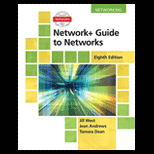
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
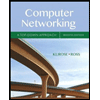
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
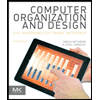
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
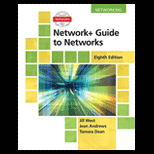
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
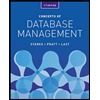
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
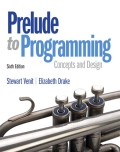
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
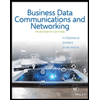
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY