I need help with a java code problem that needs this output shown in image below: import java.util.Scanner; import java.util.NoSuchElementException; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int val1 = 0, val2 = 0, val3 = 0; // Initialize the variables int max; int inputCounter = 0; // Counter to keep track of the number of inputs read try { String inputLine = scnr.nextLine(); String[] parts = inputLine.split(" "); if (parts.length > 0 && !parts[0].isEmpty()) { val1 = Integer.parseInt(parts[0]); inputCounter++; } if (parts.length > 1 && !parts[1].isEmpty()) { val2 = Integer.parseInt(parts[1]); inputCounter++; } if (parts.length > 2 && !parts[2].isEmpty()) { val3 = Integer.parseInt(parts[2]); inputCounter++; } // If no inputs were provided if(inputCounter <= 1 ) { throw new NoSuchElementException(); } // Determine the max value max = val1; if (val2 > max) { max = val2; } if (val3 > max) { max = val3; } System.out.println(max); } catch (NoSuchElementException ex) { // If exception is caught, check how many inputs were read and print the results if (inputCounter == 0) { System.out.println("0 input(s) read:\nNo max"); } else if (inputCounter == 1) { System.out.println("1 input(s) read:\nMax is " + val1); } } catch (NumberFormatException ex) { System.out.println("Invalid input. Please provide integers."); } } }
I need help with a java code problem that needs this output shown in image below:
import java.util.Scanner;
import java.util.NoSuchElementException;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int val1 = 0, val2 = 0, val3 = 0; // Initialize the variables
int max;
int inputCounter = 0; // Counter to keep track of the number of inputs read
try {
String inputLine = scnr.nextLine();
String[] parts = inputLine.split(" ");
if (parts.length > 0 && !parts[0].isEmpty()) {
val1 = Integer.parseInt(parts[0]);
inputCounter++;
}
if (parts.length > 1 && !parts[1].isEmpty()) {
val2 = Integer.parseInt(parts[1]);
inputCounter++;
}
if (parts.length > 2 && !parts[2].isEmpty()) {
val3 = Integer.parseInt(parts[2]);
inputCounter++;
}
// If no inputs were provided
if(inputCounter <= 1 ) {
throw new NoSuchElementException();
}
// Determine the max value
max = val1;
if (val2 > max) {
max = val2;
}
if (val3 > max) {
max = val3;
}
System.out.println(max);
} catch (NoSuchElementException ex) {
// If exception is caught, check how many inputs were read and print the results
if (inputCounter == 0) {
System.out.println("0 input(s) read:\nNo max");
} else if (inputCounter == 1) {
System.out.println("1 input(s) read:\nMax is " + val1);
}
} catch (NumberFormatException ex) {
System.out.println("Invalid input. Please provide integers.");
}
}
}


Step by step
Solved in 3 steps with 1 images

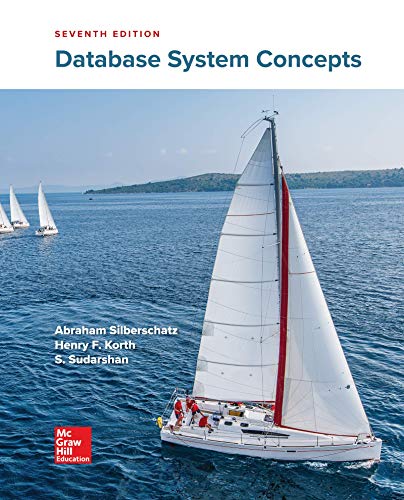
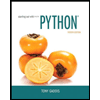
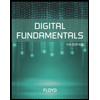
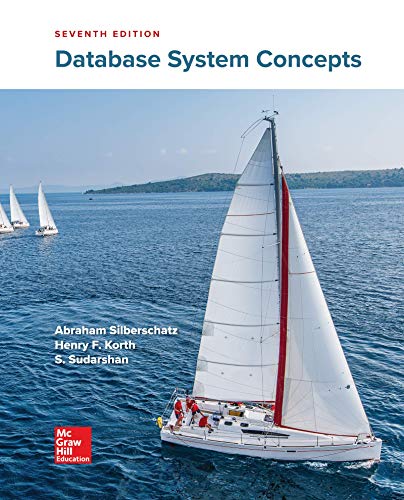
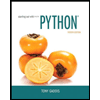
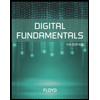
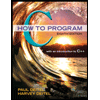
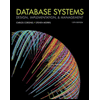
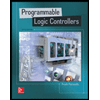