3. What is the below program doing? How is it doing this? Be precise #include using std::cout; using std::endl; int main(){ } float myValue = 10.0; float* maybeFirst, maybeSecond = 5.0, *maybe Third; maybeFirst = &myValue; for(int i = 0; i < 4; i++){ if(i % 2 == 0){ } else{ } maybeThird = &maybeSecond; maybe Third = &myValue; *maybeFirst += *maybe Third; } cout << "My value is: " << myValue << endl; return 0;
3. What is the below program doing? How is it doing this? Be precise #include using std::cout; using std::endl; int main(){ } float myValue = 10.0; float* maybeFirst, maybeSecond = 5.0, *maybe Third; maybeFirst = &myValue; for(int i = 0; i < 4; i++){ if(i % 2 == 0){ } else{ } maybeThird = &maybeSecond; maybe Third = &myValue; *maybeFirst += *maybe Third; } cout << "My value is: " << myValue << endl; return 0;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:The program provided is written in C++ and demonstrates basic pointer manipulation and control flow using a loop. Here’s a detailed explanation of what the code is doing:
### Explanation
1. **Header Files and Namespace:**
- `#include <iostream>`: This includes the standard input-output stream library, which allows the program to use `cout` and `endl` for console output.
- `using std::cout; using std::endl;`: These statements indicate that `cout` and `endl` can be used directly without prefixing them with `std::`.
2. **Main Function:**
- `int main(){`: The entry point of the program.
3. **Variable Declarations:**
- `float myValue = 10.0;`: A floating-point variable `myValue` is initialized to 10.0.
- `float* maybeFirst, maybeSecond = 5.0, *maybeThird;`:
- `maybeFirst` and `maybeThird` are pointer variables that can point to `float` values.
- `maybeSecond` is a floating-point variable initialized to 5.0.
4. **Pointer Initialization:**
- `maybeFirst = &myValue;`: `maybeFirst` is assigned the address of `myValue`.
5. **For Loop:**
- `for(int i = 0; i < 4; i++){`: A loop that will execute 4 times with the variable `i` ranging from 0 to 3.
- `if(i % 2 == 0){ maybeThird = &maybeSecond; } else { maybeThird = &myValue; }`:
- Checks if `i` is even. If true, `maybeThird` points to `maybeSecond`; otherwise, `maybeThird` points to `myValue`.
6. **Pointer Dereferencing and Arithmetic:**
- `*maybeFirst += *maybeThird;`:
- This adds the value that `maybeThird` points to `maybeFirst`.
- Since `maybeFirst` initially points to `myValue`, the value of `myValue` gets updated.
7. **Output:**
- `cout << "My value is: " << myValue << endl;`: Prints the updated value of `myValue` after the loop finishes executing.
8. **Return Statement:**
- `return 0;
Expert Solution

Step 1: Providing Algorithm of code .
1. Initialize a float variable myValue with a value of 10.0.
2. Declare float variables maybeFirst, maybeSecond with an initial value of 5.0, and a pointer maybeThird.
3. Set maybeFirst to the initial value of myValue.
4. For i from 0 to 3:
a. If i is even:
i. Point maybeThird to maybeSecond.
b. If i is odd:
i. Point maybeThird to myValue.
c. Add the value pointed to by maybeThird to maybeFirst.
5. Print "My value is: " followed by the value of maybeFirst.
6. Exit.
Step by step
Solved in 5 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
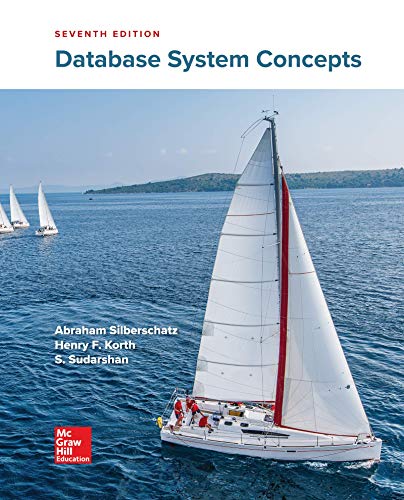
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
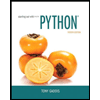
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
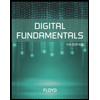
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
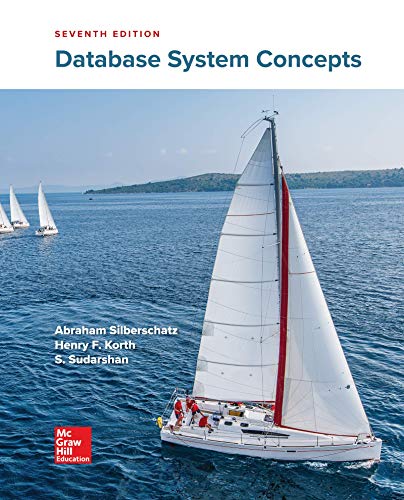
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
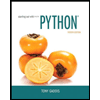
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
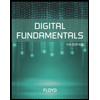
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
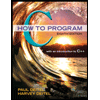
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
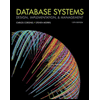
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
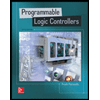
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education