Declare integer variables that will represent five dice. Using random numbers, "roll" the dice (so each die can have only the values from 1 to 6). Make sure you "seed" the random number generator as shown in Program 3-25. Print which roll you're on and what each die has on it. If a round has no matches, please indicate that. When all five dice have the same value, stop the loop and print out "YAHTZEE!". Output should look similar to this:
Instructions for the assignment can be found in the picture linked to this post. The programs must be written in c++ and requires that the random number generator be seeded like this program:
Program 3.25


#include<iostream>
#include<cstdlib>
#include<ctime>
int main()
{
unsigned seed=time(0);
srand(seed);
int i=1; //variable to store the number of roll
while(true){
//rand%6 generates a random number in range of 0 to 5 so adding gives vales from 1 to 6
int dice1= rand()%6 +1;
int dice2= rand()%6 +1;
int dice3= rand()%6 +1;
int dice4= rand()%6 +1;
int dice5= rand()%6 +1;
//printing roll information
cout << "Roll " << i << " : " << dice1 << " " << dice2 << " " << dice3 << " " << dice4 << " " << dice5;
//you can use nested for instead of this if condition
//compare each dice value with the previous dice
//and print no matches only if all are unique
if(dice2!=dice1 && dice3!=dice1 && dice3!=dice2 && dice4!=dice3 && dice4!=dice2 && dice4!=dice1 && dice5!=dice4 && dice5!=dice3 && dice5!=dice2 && dice5!=dice1){
cout << " No matches this time!";
}
//if all dice shows same value
else if(dice1==dice2 && dice2==dice3 && dice3==dice4 && dice4==dice5){
cout << "\nYAHTZEE" << endl;
return 0; //stop execution
}
cout << endl;
i++; //incrementiing roll
}
return 0;
}
Step by step
Solved in 2 steps with 1 images

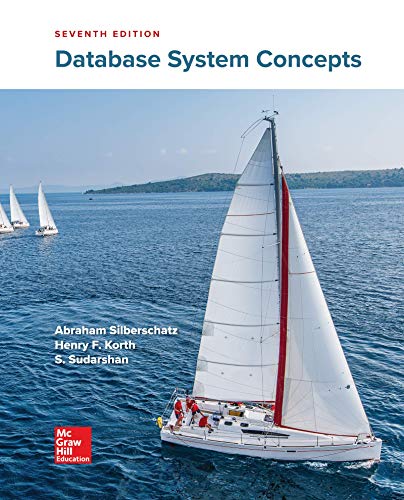
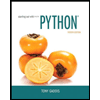
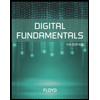
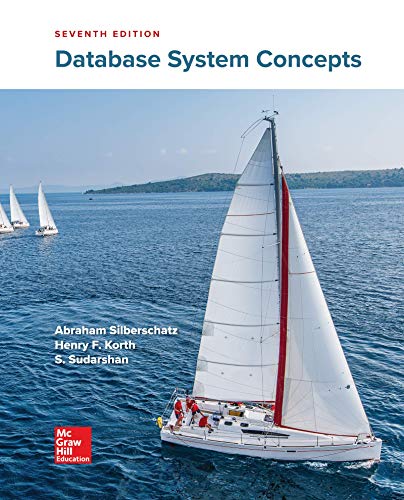
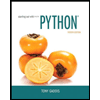
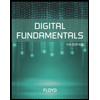
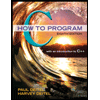
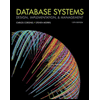
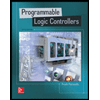