Assume there is no compiling or linking error. Review the following pieces of code and briefly explain potential issues. A. std::string &get_hello() { std::string s = "hello"; return s; } B. class time { int hour, min, sec; public: bool set(int h, int m, int s); int get_hour() const; int get_min() const; int get_sec() const; }; C. class collection { }; class my_array : public collection { std::vector vec_; }; void test() { collection *p = new my_array; delete p; }
Assume there is no compiling or linking error. Review the following
pieces of code and briefly explain potential issues.
A. std::string &get_hello() {
std::string s = "hello";
return s;
}
B. class time {
int hour, min, sec;
public:
bool set(int h, int m, int s);
int get_hour() const;
int get_min() const;
int get_sec() const;
};
C. class collection {
};
class my_array : public collection {
std::
};
void test() {
collection *p = new my_array;
delete p;
}

Explanation:
A. The get_hello function returns a reference to a string, but the string is declared locally within the function. This means that when the function returns, the string will be destroyed and the reference will be invalid.
B. The time class does not have a constructor defined, so the member variables will not be initialized. The set function should be marked as const because it does not modify the object.
C. The destructor for my_array is not defined, so it will inherit the destructor from collection. This will not work properly because the vector will not be destroyed.
Step by step
Solved in 2 steps

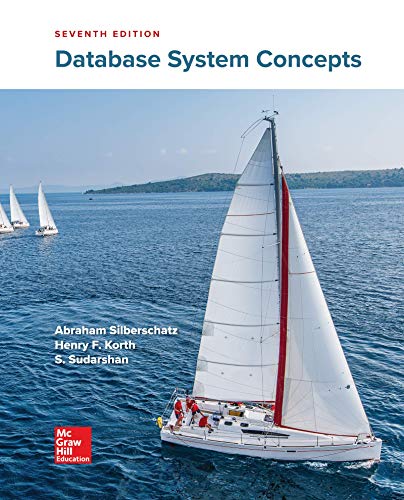
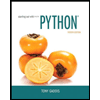
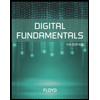
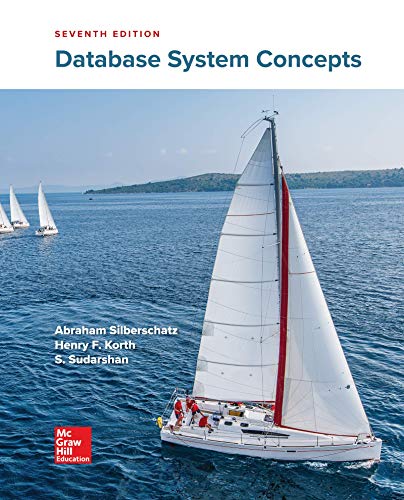
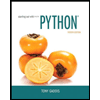
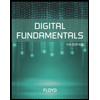
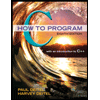
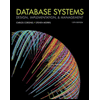
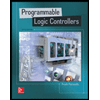