Copy the main function and complete set function. The set function takes two arrays x, y and their lengths as input. If the entire array y is found in the array x, the part is set to 0. a1 1 2 3 4 1 2 3 4 5 a2 1 2 3 000400045 al a2 b1 b2 cl : c2 d1 d2 1 0 0 0 1000 5 2 3 4 0 0 1 3 500 36700 3 3 0 2 3 0 2 3 0 0 2 3 1 0 0 0 0 0 1 2 3 4 0 0 0 0 0 1 2 3 1 2 3 4 5
Copy the main function and complete set function. The set function takes two arrays x, y and their lengths as input. If the entire array y is found in the array x, the part is set to 0. a1 1 2 3 4 1 2 3 4 5 a2 1 2 3 000400045 al a2 b1 b2 cl : c2 d1 d2 1 0 0 0 1000 5 2 3 4 0 0 1 3 500 36700 3 3 0 2 3 0 2 3 0 0 2 3 1 0 0 0 0 0 1 2 3 4 0 0 0 0 0 1 2 3 1 2 3 4 5
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Use C++
![**Instructions:**
- Copy the main function and complete the **set** function.
- The **set** function takes two arrays, **x**, **y** and their lengths as input.
- If the entire array **y** is found in the array **x**, the part is set to 0.
**Diagrams:**
1. **Arrays Diagram:**
- **a1:** Initial array [1, 2, 3, 4, 1, 2, 3, 4, 5]
- **a2:** Sub-array to search [1, 2, 3]
After applying the function, modified **a1** becomes:
- 0, 0, 0, 4, 0, 0, 0, 4, 5
**Code Explanation:**
- **a1:** The original array where we need to search for the sub-array **a2.**
- **a2:** The sub-array we are trying to find in **a1.** If **a2** appears in **a1,** the corresponding section of **a1** is replaced with zeros.
**Input Data for Function:**
- **a1:** [1 0 0 0 1 0 0 0 5]
- **a2:** [2 3 4]
- **b1:** [0 0 1 3 5 0 0 3 6 7 0 0]
- **b2:** [3 3]
- **c1:** [0 2 3 0 2 3 0 0 2 3]
- **c2:** [1]
- **d1:** [0 0 0 1 2 3 4 0 0 0 0 0 0 1 2 3]
- **d2:** [1 2 3 4 5]
This educational resource is intended to illustrate how specific elements of an array can be modified based on the presence of a sub-array.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcdbdf4c8-5459-45de-99f8-cd8d71031beb%2F53d280a3-1fb3-4a50-b181-b8828f62295c%2F4kwz1wp_processed.jpeg&w=3840&q=75)
Transcribed Image Text:**Instructions:**
- Copy the main function and complete the **set** function.
- The **set** function takes two arrays, **x**, **y** and their lengths as input.
- If the entire array **y** is found in the array **x**, the part is set to 0.
**Diagrams:**
1. **Arrays Diagram:**
- **a1:** Initial array [1, 2, 3, 4, 1, 2, 3, 4, 5]
- **a2:** Sub-array to search [1, 2, 3]
After applying the function, modified **a1** becomes:
- 0, 0, 0, 4, 0, 0, 0, 4, 5
**Code Explanation:**
- **a1:** The original array where we need to search for the sub-array **a2.**
- **a2:** The sub-array we are trying to find in **a1.** If **a2** appears in **a1,** the corresponding section of **a1** is replaced with zeros.
**Input Data for Function:**
- **a1:** [1 0 0 0 1 0 0 0 5]
- **a2:** [2 3 4]
- **b1:** [0 0 1 3 5 0 0 3 6 7 0 0]
- **b2:** [3 3]
- **c1:** [0 2 3 0 2 3 0 0 2 3]
- **c2:** [1]
- **d1:** [0 0 0 1 2 3 4 0 0 0 0 0 0 1 2 3]
- **d2:** [1 2 3 4 5]
This educational resource is intended to illustrate how specific elements of an array can be modified based on the presence of a sub-array.
![```cpp
int main()
{
int a1[] = {1, 2, 3, 4, 1, 2, 3, 4, 5};
int a2[] = {2, 3, 4};
int b1[] = {3, 3, 1, 3, 5, 3, 3, 3, 6, 7, 3, 3};
int b2[] = {3, 3};
int c1[] = {1, 2, 3, 1, 2, 3, 1, 1, 2, 3};
int c2[] = {1};
int d1[] = {1, 2, 3, 4, 5, 1, 2, 3, 4, 1, 2, 3, 4, 5, 1, 2, 3};
int d2[] = {1, 2, 3, 4, 5};
zero(a1, 9, a2, 3);
zero(b1, 12, b2, 2);
zero(c1, 10, c2, 1);
zero(d1, 17, d2, 5);
cout << "a1 : " << print(a1, 9) << endl;
cout << "a2 : " << print(a2, 3) << endl;
cout << "b1 : " << print(b1, 12) << endl;
cout << "b2 : " << print(b2, 2) << endl;
cout << "c1 : " << print(c1, 10) << endl;
cout << "c2 : " << print(c2, 1) << endl;
cout << "d1 : " << print(d1, 17) << endl;
cout << "d2 : " << print(d2, 5) << endl;
}
```
### Explanation:
This C++ code snippet defines a `main()` function containing various integer arrays and invokes a placeholder function `zero()`. The purpose of the `zero()` function is not clear from the code snippet itself, as its implementation is not provided. However,](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcdbdf4c8-5459-45de-99f8-cd8d71031beb%2F53d280a3-1fb3-4a50-b181-b8828f62295c%2Fb4kkd6n_processed.jpeg&w=3840&q=75)
Transcribed Image Text:```cpp
int main()
{
int a1[] = {1, 2, 3, 4, 1, 2, 3, 4, 5};
int a2[] = {2, 3, 4};
int b1[] = {3, 3, 1, 3, 5, 3, 3, 3, 6, 7, 3, 3};
int b2[] = {3, 3};
int c1[] = {1, 2, 3, 1, 2, 3, 1, 1, 2, 3};
int c2[] = {1};
int d1[] = {1, 2, 3, 4, 5, 1, 2, 3, 4, 1, 2, 3, 4, 5, 1, 2, 3};
int d2[] = {1, 2, 3, 4, 5};
zero(a1, 9, a2, 3);
zero(b1, 12, b2, 2);
zero(c1, 10, c2, 1);
zero(d1, 17, d2, 5);
cout << "a1 : " << print(a1, 9) << endl;
cout << "a2 : " << print(a2, 3) << endl;
cout << "b1 : " << print(b1, 12) << endl;
cout << "b2 : " << print(b2, 2) << endl;
cout << "c1 : " << print(c1, 10) << endl;
cout << "c2 : " << print(c2, 1) << endl;
cout << "d1 : " << print(d1, 17) << endl;
cout << "d2 : " << print(d2, 5) << endl;
}
```
### Explanation:
This C++ code snippet defines a `main()` function containing various integer arrays and invokes a placeholder function `zero()`. The purpose of the `zero()` function is not clear from the code snippet itself, as its implementation is not provided. However,
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
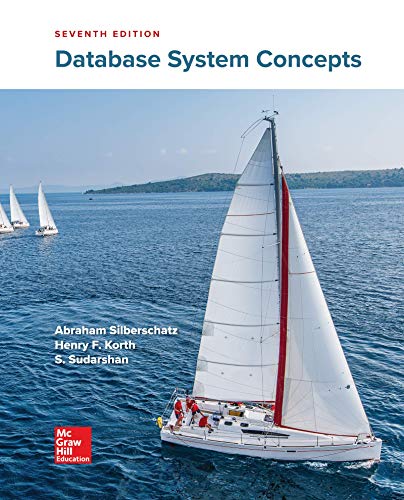
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
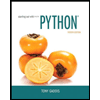
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
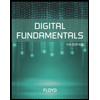
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
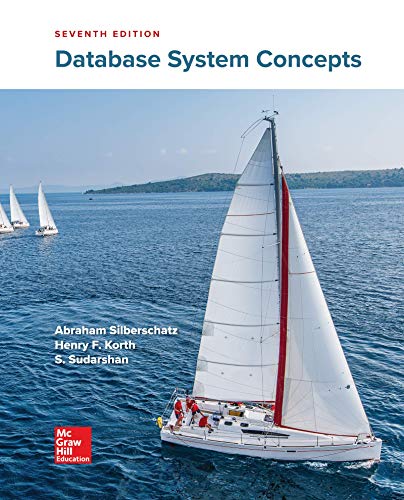
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
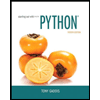
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
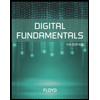
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
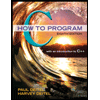
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
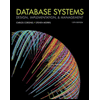
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
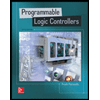
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education